Question
create a GUI front end for Homework #1. See details below. 1. Create a GUI that has two check boxes which allow a user to
create a GUI front end for Homework #1. See details below.
1. Create a GUI that has two check boxes which allow a user to select between an employee and a manager.
2. Create a GUI that pops up ONLY if the employee box is selected. This new window should contain JLabels with JTextFields that allow the user to enter in all the employee's relevant information - e.g. Name, Title, Age, and Salary. Use the same inputs from the Jane Doe case in Homework #1. The new window should also allow the user to raise the employee's salary by a certain percentage - JTextField (% Increase). Use the employee raiseSalary(int percent) method from Homework #1. Lastly, the new window should include a calculate button which computes and outputs the new salary when pressed. In this case, the new salary would be 82125.0.
3. Create a GUI that pops up ONLY if the manager box is selected. This new window should contain JLabels with JTextFields that allow the user to enter in all the manager's relevant information - e.g. Name, Title, Age, Years of Experience, Highest Degree Earned, Salary, and Promotion Bonus. Use the same inputs from the John Doe case in Homework #1. The new window should also allow the user to raise the manager's salary by a certain percentage - JTextField (% Increase). Use the manager raiseSalary(int percent) method from Homework #1. Lastly, the new window should include a calculate button which computes and outputs the new salary when pressed. In this case, the new salary would be 114500.0.
4. Create a GUI error message that pops up if the user enters invalid information into the GUI from question #2.
5. Create a GUI error message that pops up if the user enters invalid information into the GUI from question #3.
Develop a report that contains an overview of the source code. It should also include print screens of the GUIs from each scenario mentioned above. Copy source code and paste in the report's Appendix similar to what was provided in the Homework #1 assignment. Note, all responses should be contained in ONE WORD or PDF document. Other formats will not be accepted.
APPENDIX
Traits.java
public interface Traits{
public String getName();
public String getTitle();
}
Employee.java
public class Employee implements Traits {
private String myName;
private String myTitle;
protected double mySalary;
private int myAge;
public Employee(String name, String title, double salary, int age) {
myName = name;
myTitle = title;
mySalary = salary;
myAge = age;
}
public String getName() {
return myName;
}
public String getTitle(){
return myTitle;
}
public double getSalary() {
return mySalary;
}
public int getAge(){
return myAge;
}
public void raiseSalary(int percent) {
mySalary = mySalary + 0.95 * percent * 0.01 * mySalary;
}
public void raiseSalary(int percent, int cost_of_living_adjustment) {
mySalary = mySalary + 0.95 * percent * 0.01 * mySalary + cost_of_living_adjustment * 0.01 * mySalary;
}
}
Manager.java
public class Manager extends Employee{
private int yearsWorked;
private String highestDegree;
private double promotionBonus;
public Manager(String name, String title, double salary, int age, int experience, String degree, double bonus){
super(name, title, salary, age);
yearsWorked = experience;
highestDegree = degree;
promotionBonus = bonus;
}
public int getExperience(){
return yearsWorked;
}
public String getDegree(){
return highestDegree;
}
public double getBonus(){
return promotionBonus;
}
public void raiseSalary(int percent) {
mySalary = mySalary + 0.95 * percent * 0.01 * mySalary + promotionBonus;
}
}
Test.java
public class Test{
public static void main(String args[]){
Manager m = new Manager("John Doe", "Department Head", 100000.0, 54, 31, "PhD", 5000.0);
Employee e1 = new Employee("Jane Doe", "Resource Analyst", 75000.0, 41);
Employee e2 = new Employee("Jim Doe", "Engineer", 85000.0, 45);
System.out.println("Name : " + m.getName());
System.out.println("Title : " + m.getTitle());
System.out.println("Age : " + m.getAge());
System.out.println("Years of Experience : " + m.getExperience());
System.out.println("Highest Degree Earned : " + m.getDegree());
System.out.println("Salary : " + m.getSalary());
System.out.println("Promotion Bonus : " + m.getBonus());
m.raiseSalary(10);
System.out.println("Salary Raised!");
System.out.println("New Salary : " + m.getSalary());
System.out.println(" ");
System.out.println("Name : " + e1.getName());
System.out.println("Title : " + e1.getTitle());
System.out.println("Age : " + e1.getAge());
System.out.println("Salary : " + e1.getSalary());
e1.raiseSalary(10);
System.out.println("Salary Raised");
System.out.println("New Salary : " + e1.getSalary());
System.out.println(" ");
System.out.println("Name : " + e2.getName());
System.out.println("Title : " + e2.getTitle());
System.out.println("Age : " + e2.getAge());
System.out.println("Salary : " + e2.getSalary());
e2.raiseSalary(10,1);
System.out.println("Salary Raised");
System.out.println("New Salary : " + e2.getSalary());
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
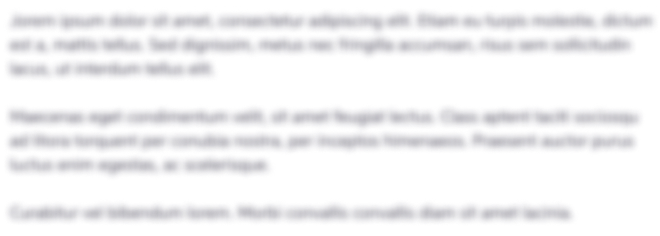
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started