Question
Create a Java program that demonstrate understanding of reading input from the console by creating an interactive program. Related SLOs: SLO #1: Use
Create a Java program that demonstrate understanding of reading input from the console by creating an interactive program.
Related SLOs:
SLO #1: Use an appropriate programming environment to design, code, compile, run, and debug computer programs.
SLO #4: Demonstrate working with primitive data types, strings, and arrays.
Instructions:
Create a program that will assist a person traveling outside of the U.S.
Using jGRASP, write a Java program named LastnameFirstname03.java, using your last name (Doe) and your first name (John), that does the following:
-Ask the user for their name. Address them by name and welcome them to the city/country (pick whichever country you want).
-Ask the user what 3 items they want to buy.
-Ask the user what the price is for each item.
-Add up the total for the items. Present the total of all items in both US Dollars and the country's currency. (See Example Output)
Output the answer so it's accurate to two digits after the decimal and displays at least 6 digits total
Note: You'll need to google the conversion rate to convert between US Dollars and the other currency.
-Say goodbye to the user.
Important: Please remember that you (the programmer) are getting input from the user (someone who uses your program). You do not have any control over what they will type in, so they can type anything they want for the items and the prices. Your program should reflect the user's input. Note that in the Example Output below, the user's input is denoted by orange font (same as jGrasp, as shown in the lecture videos).
Your program MUST:
Use at least 1 constant
Use at least 3 variables
Print the contents of every variable in your program used for getting input from the user
Include comments that explain what you are doing in your code
Adhere to the Java coding standard
Your program should not use code/concepts not yet covered. Use syntax for basic java programing:
Java skeleton : you need this for every Java program you'll write.
System.out.print() : used for outputting text such as "Hello, world!"
Syntax for declaring a variable: datatype variableName; for example in previous programs:
- int: integers like 100
- double: decimals like 3.1459
- char: a single character like '?'
- boolean: a boolean value
- String: a string of characters like "Hello, CIS 105 class! :)
Remember to always begin your code with the following documentation comments:
/**
* Short description of the program.
*
* @author Last Name, First Name
* @assignment CIS 105 Assignment XX
* @date Today's Date
* @bugs Short description of bugs in the program, if any.
*/
Expected Output:
This is an example of what your program should output:
----jGRASP exec: java JaneMary03
Greetings! What's your name?
Mary
Welcome to New Zealand, Mary!
I see that you're interested in buying some items.
Please list the three items you'd like to purchase:
1. fish and chips
2. one of those red double-decker buses
3. bo'owh'o'wo'er
Sure, let me add up those items for you!
Please enter the cost for each item:
fish and chips: £7.49
one of those red double-decker buses: £450000
bo'owh'o'wo'er: £1.25
Okay! Here is your receipt:
£7.49 fish and chips
£450,000.00 one of those red double-decker buses
£1.25 bo'owh'o'wo'er
Total: £450,008.74
The equivalent in US dollars is: $612,011.89
Enjoy your items and have a great time in New Zealand!
----jGRASP: operation complete.
Criteria:
-Java coding standard errors
-Submit to specifications.
-use at least 1 constant
-use at least 3 variables
-use/print out all variable and constant values
-output/calculations are correct
-properly commented
-no miscellaneous mistakes, bugs, or problems.
Step by Step Solution
3.45 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
java A program that assists a person traveling outside of the US It asks the user for their name three items they want to buy and the prices of those ...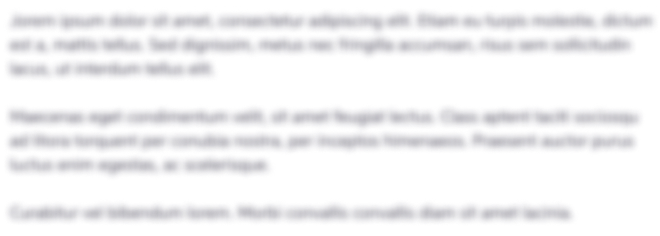
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started