Question
create a java program with 2 classes. First Class: The first class is called Student this class will implement Comparable (see the code below). Class
create a java program with 2 classes.
First Class:
The first class is called Student this class will implement Comparable (see the code below).
Class student will have three private fields String studentName, int studentID, int studetnAge.
Class student will also have a constructor that takes in all three of those parameters to create an instance of student.
For this class I am not requiring a default constructor, but you need getter and setter methods for each field.
If you look into the Comprarable interface (remember press control and hover over Comparable then left click twice and you can view the interface code to know what you need to implement) you only need to implement one method called compareTo().
The compareTo method should sort the students by age o This means you need to get the student age and compare it to another age. (see code below)
Lastly, you should override your toString method similar to assignment 2 so that you can output the studentID, Name, and Age on one line.
Second Class:
The second class will be your Main class which will contain your main method (where all the output comes out from).
In this class you will initialize at least three students and pass the Name, Student ID, and Age to each instance being initialized.
After that you will create an ArrayList of type student, and insert the instances of Student into your ArrayList (Hint: arrayListVariableName.add(student variable name).
Now you can use the Collections.sort(arraylist) method to sort your students by ascending age.
Lastly utilize a for loop in order to iterate through the arraylist and print out each element. (hint: this is just like GenericsDemo2 except instead of a generic array we are utilizing an ArrayList of type student.
Code:
public class Student implements Comparable {
//place fields here
public Student(int studentID, String studentName, int studentAge) {
//complete constructor here
}
//getter methods and setter methods go here
//This Override method is required to implement Comparable sort the student by age in ascending order
@Override
public int compareTo(Student compareStudent) {
//(Student) here is the casting, getStudentAge should be a getter method for the age field.
int compareAge = ((Student) compareStudent).getStudentAge();
//this is the return for ascending order, since you are new to sorting I gave you the methodology
//you no longer have to write any code for this method.
return this.studentage compareAge;
}
@Override
public String toString(){
return // fill in code here and remember the return is the studentID, the student name, and the studentAge on one line
}//end of the toString method bracket
}//end of the Student class bracket
public class Main {
public static void main(String args[]){
//Create ArrayList of type student
//Create 3 or more students (make sure the age is unique, so we can test the sorting algorithm). You need to use the new keyword here.
//Use the Collections.sort(ArraList) method, remember for this to work you have to import java.util.*;
//Use a for loop in order to output each element in the array list.
}//bracket that closes off your main method
}//bracket that closes off your Main class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
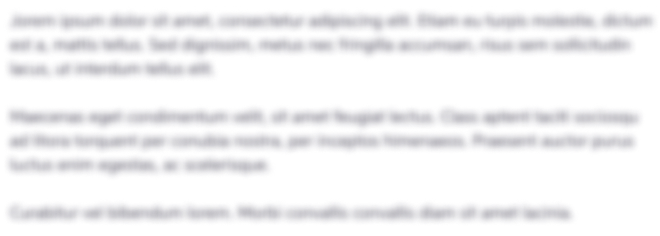
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started