Question
Create a JUNIT test program in Java for this program: package edu.odu.cs.cs350; import java.util.Arrays; /** * A duration represents a period of elapsed time, e.g.,
Create a JUNIT test program in Java for this program:
package edu.odu.cs.cs350; import java.util.Arrays; /** * A duration represents a period of elapsed time, e.g., 4 hours, 23 minutes, 2 seconds. * This is differentiated from a point in time (e.g., 4:23:02AM). A meeting that * has a duration of 2 hours has that same duration no matter where it was held. A * starting time (point) for that of 2:00PM EST can be unambiguous only if the time * zone is added. * ** Because durations are often used in calculations, both positive and * negative values are possible. * *
* Most of the accessor functions for this class will respond with normalized * values, where the normalization rules are as follows: *
- *
- The seconds and minutes components will have an absolute * value in the range 0..59, inclusive. *
- The hours component will have an absolute value in the * range 0..23, inclusive. *
- The sign of each component matches the sign of the * overall duration. A duration of -61 seconds, for example, * has normalized components of -1 seconds and -1 minutes. *
*This is the template to fill in for the solution - please fill in these functions to complete the JUNIT Test Class*
/** * */ package edu.odu.cs.cs350;
import static org.junit.Assert.*;
import org.junit.After; import org.junit.AfterClass; import org.junit.Before; import org.junit.BeforeClass; import org.junit.Test;
/** * @author Colten Everitt * */ public class DurationClassTest {
/** * @throws java.lang.Exception */ @BeforeClass public static void setUpBeforeClass() throws Exception { }
/** * @throws java.lang.Exception */ @AfterClass public static void tearDownAfterClass() throws Exception { }
/** * @throws java.lang.Exception */ @Before public void setUp() throws Exception { }
/** * @throws java.lang.Exception */ @After public void tearDown() throws Exception { }
/** * Test method for {@link edu.odu.cs.cs350.Duration#hashCode()}. */ @Test public final void testHashCode() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#Duration()}. */ @Test public final void testDuration() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#Duration(long)}. */ @Test public final void testDurationLong() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#Duration(int, int, int, int)}. */ @Test public final void testDurationIntIntIntInt() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#getTotalSeconds()}. */ @Test public final void testGetTotalSeconds() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#setTotalSeconds(long)}. */ @Test public final void testSetTotalSeconds() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#getDays()}. */ @Test public final void testGetDays() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#getHours()}. */ @Test public final void testGetHours() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#getMinutes()}. */ @Test public final void testGetMinutes() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#getSeconds()}. */ @Test public final void testGetSeconds() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#add(edu.odu.cs.cs350.Duration)}. */ @Test public final void testAdd() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#subtract(edu.odu.cs.cs350.Duration)}. */ @Test public final void testSubtract() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#scale(double)}. */ @Test public final void testScale() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#toString()}. */ @Test public final void testToString() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#equals(java.lang.Object)}. */ @Test public final void testEqualsObject() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link edu.odu.cs.cs350.Duration#clone()}. */ @Test public final void testClone() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link java.lang.Object#Object()}. */ @Test public final void testObject() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link java.lang.Object#getClass()}. */ @Test public final void testGetClass() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link java.lang.Object#equals(java.lang.Object)}. */ @Test public final void testEqualsObject1() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link java.lang.Object#clone()}. */ @Test public final void testClone1() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link java.lang.Object#toString()}. */ @Test public final void testToString1() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link java.lang.Object#notify()}. */ @Test public final void testNotify() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link java.lang.Object#notifyAll()}. */ @Test public final void testNotifyAll() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link java.lang.Object#wait(long)}. */ @Test public final void testWaitLong() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link java.lang.Object#wait(long, int)}. */ @Test public final void testWaitLongInt() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link java.lang.Object#wait()}. */ @Test public final void testWait() { fail("Not yet implemented"); // TODO }
/** * Test method for {@link java.lang.Object#finalize()}. */ @Test public final void testFinalize() { fail("Not yet implemented"); // TODO }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
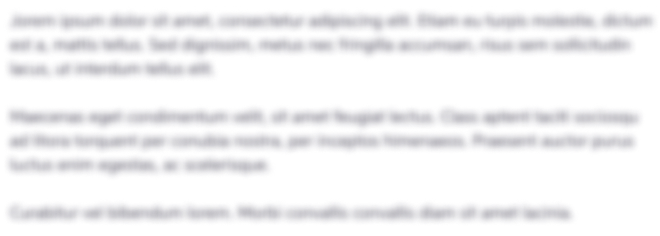
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started