Question
Create a new C++ project. Call OverloadedFunctionsDemo. Add standard includes and main function: #include #include using namespace std; int main() { return 0; } Add
- Create a new C++ project. Call OverloadedFunctionsDemo.
- Add standard includes and main function:
#include
#include
using namespace std;
int main()
{
return 0;
}
- Add Some Overloaded Functions
- Add the following functions:
void SayGoodnight()
{
cout << "Good night!" << endl;
}
void SayGoodnight(string name1)
{
cout << "Good night " << name1 << "!" << endl;
}
void SayGoodnight(string name1, string name2)
{
cout << "Good " << name1 << " and " << name2 << "!" << endl;
}
void SayGoodnight(int number)
{
for (int i = 0; i < number; ++i)
{
cout << "Good night! " << endl;
}
}
- Add function prototypes at top of program:
void SayGoodnight();
void SayGoodnight(string name1);
void SayGoodnight(string name1, string name2);
void SayGoodnight(int number);
- Call functions from main:
- Call the parameterless function:
SayGoodnight();
- Run with ctrl-F5
- Add a call to the one parameter function:
string name;
getline(cin, name);
SayGoodnight(name);
- Run with ctrl-F5
- Add a call to the two parameter function:
cout << "Name one: ";
string nameFirst;
getline(cin, nameFirst);
cout << "Name second: ";
string nameSecond;
getline(cin, nameSecond);
SayGoodnight(nameFirst,nameSecond);
- Run with ctrl-F5
- Add a call to the function that takes one int parameter:
cout << "Number: ";
int number;
cin >> number;
SayGoodnight(number);
- Run with ctrl-F5
Question: How does C++ differentiate between SayGoodnight(name); and SayGoodnight(number);?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
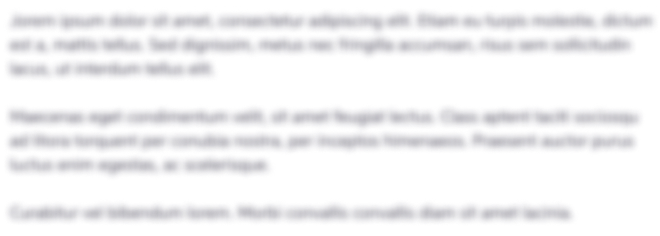
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started