Question
Create a new class called Box which is a very short helper class to display the name/assignment number box that youve done in each program
Create a new class called Box which is a very short helper class to display the name/assignment number box that youve done in each program so far
Declare an integer instance variable (global scope) called projectNum.
Since this is a helper class, Box will have no main method
Create the constructor of Box to accept one integer parameter, the project number called pnumb. Remember that a constructor is a method and therefore needs a method header; the method name for a constructor will always be the same as the class name, and there is no return type.
Following the method header, the constructor, like all methods surrounds its code with { and }
Inside the code of the constructor, the constructor assigns pnumb to the instance variable projectNum; this method should only be one line long.
In the class Box, create a second method called toString. The header for toString should take no parameters, but should return a String object.
toString should return ONE string containing the usual box with your name, CSC 2014, your section number, and the Project number. It will use the project number that was passed in when the Box class was instantiated and stored in projectNum. Since we want this string to print over multiple lines, we must use the correct escape characters. Remember that multiple strings can be appended together using the + operator
NOTE: The toString method returns a string to the driver (the program that called it). The driver will print out the string that it gets back from the toString method. When printed, this string will look like the box with our name and project number that we did earlier labs
Save Box class as Box.java
Make a second class, called TestBox, in its own file. This class should have a main method, and that main method should contain code to create an instance of the Box class using the number of this assignment, and then print out that Box using println(). It should look something like this:
public class TestBox {
public static void main(String[] args) {
Box myBox = new Box(5);
System.out.println(myBox.toString());
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
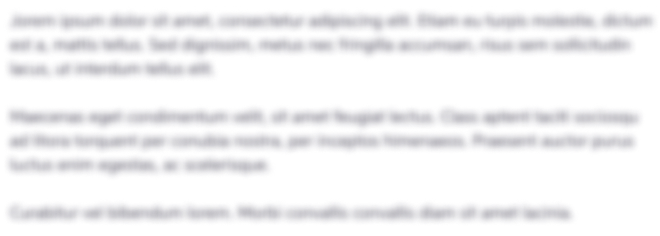
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started