Question
Create a new main for the classes CatRecord, DogRecord and BirdRecord which inherit from (extend) the PetRecord class (see code below). In this main, create
Create a new main for the classes CatRecord, DogRecord and BirdRecord which inherit from (extend) the PetRecord class (see code below). In this main, create an array of 3 PetRecord objects. Fill one slot of the array with a CatRecord, one with a DogRecord, and one with a BirdRecord, and fill each with appropriate data. Call print on each and observe that the version from the specific animal type is used, not the generic type in PetRecord this is due to polymorphism.
Here's my code:
PetRecord class:
public class PetRecord {
private String name;
private int age;
private double weight;
public String toString() {
return ("Name: " + name + " Age: " + age + " years" + " Weight: " + weight + " pounds");
}
public PetRecord(String initialName, int initialAge, double initialWeight) {
name = initialName;
if ((initialAge < 0) || (initialWeight < 0)) {
System.out.println("Error: Negative age or weight.");
System.exit(0);
} else {
age = initialAge;
weight = initialWeight;
}
}
public void set(String newName, int newAge, double newWeight) {
name = newName;
if ((newAge < 0) || (newWeight < 0)) {
System.out.println("Error: Negative age or weight.");
System.exit(0);
} else {
age = newAge;
weight = newWeight;
}
}
public PetRecord(String initialName) {
name = initialName;
age = 0;
weight = 0;
}
public void setName(String newName) {
name = newName;
}
public PetRecord(int initialAge) {
name = "No name yet.";
weight = 0;
if (initialAge < 0) {
System.out.println("Error: Negative age.");
System.exit(0);
} else
age = initialAge;
}
public void setAge(int newAge) {
if (newAge < 0) {
System.out.println("Error: Negative age.");
System.exit(0);
} else
age = newAge;
}
public PetRecord(double initialWeight) {
name = "No name yet";
age = 0;
if (initialWeight < 0) {
System.out.println("Error: Negative weight.");
System.exit(0);
} else
weight = initialWeight;
}
public void setWeight(double newWeight) {
if (newWeight < 0) {
System.out.println("Error: Negative weight.");
System.exit(0);
} else
weight = newWeight;
}
public PetRecord() {
name = "No name yet.";
age = 0;
weight = 0;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public double getWeight() {
return weight;
}
public void print() {
System.out.println("Name: " + name + " Age: " + age + " years" + " Weight: " + weight + " pounds");
}
}
DogRecord class:
public class DogRecord extends PetRecord
{
private boolean hasLongHair;
public DogRecord()
{
}
public DogRecord(String initialName, double initialWeight, int intitialAge, boolean hasLongHair)
{
super(initialName, intitialAge, initialWeight);
this.hasLongHair = hasLongHair;
}
public void setHasLongHair(boolean hasLongHair)
{
hasLongHair = hasLongHair;
}
public boolean getHasLongHair()
{
return hasLongHair;
}
public void print()
{
super.print();
System.out.println("hasLongHair: " + hasLongHair);
}
}
CatRecord class:
public class CatRecord extends PetRecord
{
private boolean hasLongHair;
public CatRecord()
{
}
public CatRecord(String initialName, double initialWeight, int intitialAge, boolean hasLongHair)
{
super(initialName, intitialAge, initialWeight);
this.hasLongHair = hasLongHair;
}
public void sethasLongHair(boolean hasLongHair)
{
hasLongHair = hasLongHair;
}
public boolean gethasLongHair()
{
return hasLongHair;
}
public void print()
{
super.print();
System.out.println("hasLongHair: " + hasLongHair);
}
}
Heres my BirdRecord class:
public class BirdRecord extends PetRecord
{
private int numFeathers;
public BirdRecord()
{
}
public BirdRecord(String initialName, double initialWeight, int intitialAge, int numFeathers)
{
super(initialName, intitialAge, initialWeight);
this.numFeathers = numFeathers;
}
public void setnumFeathers(int numFeathers)
{
numFeathers = numFeathers;
}
public int getnumFeathers()
{
return numFeathers;
}
public void print()
{
super.print();
System.out.println("numFeathers: " + numFeathers);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
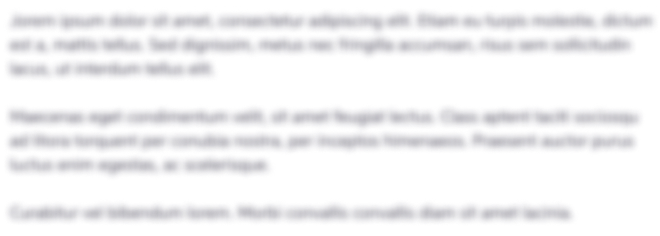
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started