Question
Create a new project in either BlueJ or NetBeans then add two classes, TasksPart2.java and TasksPart2Tests.java. (The linked files have starter code for you included
Create a new project in either BlueJ or NetBeans then add two classes, TasksPart2.java and TasksPart2Tests.java. (The linked files have starter code for you included in them.) Complete the implementation of the methods listed below as part of the TasksPart2 class; do NOT modify code in TasksPart2Tests except to uncomment lines. Add documentation at the method level using JavaDoc syntax. You should use the TasksPart2Tests.java file (which includes the main method) to test the code you've written. Please include the name of both people in your group in the class level documentation. If not checked off in class by the instructor or TA, you should each submit the lab by attaching a zip file of your completed BlueJ or NetBeans project.
- course()
- void return type.
- No parameters.
- Displays (prints) a truthful compliment about CIS 2087.
- inOrder()
- boolean return type.
- Three double parameters respectively x, y, and z.
- Returns whether x y z.
- mp3Sizer()
- int return type.
- Three int parameters named songs, videos, and photos.
- Returns an estimate in GB of the size of an MP3 player that can store the indicated media amounts. Note, if the estimate is fractional, round up.
- Assume that the amount of storage needed for a song is 3.04 MB, a video is 89.3 MB, and a photo is 1.72 MB.
- count()
- int return type.
- Two double parameters and one int parameter named respectively a, b, and mL. (mL represents milliliters)
- Returns an estimate of the number jelly beans in a jar with volume mL where a jelly bean has length a and height b (in cm).
- The volume of a jelly bean is estimated to be 5 a b2 / 24
- Assume the jelly bean loading capacity of a jar is 69.8%.
- The number of jelly beans would be calculated as v c / j where v is the volume of the jar, c is the loading capacity, and j is the estimate size of a jelly bean
You may use TasksPart2.java as a starting point for completing the implementation of the methods. The methods can be tested by uncommenting statements from the method main() of program TasksPart2Tests.java. A run of the program could produce the following output. (Note that you need to save TasksPart2.java and TasksPart2Tests.java in the same directory and should not change any code in TasksPart2Tests.java except to uncomment lines.) Trying course() course(): CIS 2087 is a cool class. course(): CIS 2087 is a cool class. Trying inOrder() TasksPart2.inOrder( 3.0, 1.5, 5.5 ): false TasksPart2.inOrder( 3.5, 4.5, 7.5 ): true Trying mp3Sizer() TasksPart2.mp3Sizer( 2000, 25, 1500 ): 11 TasksPart2.mp3Sizer( 1350, 50, 4000 ): 16 Trying count() TasksPart2.count( 1.5, 0.9, 500 ): 439 beans TasksPart2.count( 2.0, 1.0, 25 ): 14 beans
Taskspart2.java
/** * Add your JavaDoc class description here. Include names and date along with the description. * @author Your Name * * @since Today's Date */ public class TasksPart2 {
/** * Prints a truthful compliment about CIS 2087 */ public static void course() { //add code here }
// method inOrder(): returns whether its parameters are in sorted order // add JavaDoc style comments for the method // write the method signature and code yourself
// method mp3Sizer(): estimates the number of gB needed to store media // add JavaDoc style comments for the method // write the method signature and code yourself
// method count(): returns estimated number of beans in jar // add JavaDoc style comments for the method // write the method signature and code yourself
}
TaskPart2Tests.java
// purpose: experience the thrill of using methods // Change to JavaDoc style comments.
public class TasksPart2Tests {
public static void main( String[] args ) {
System.out.println( " Trying course() " );
// ****** uncomment next four lines after completing course() // System.out.print( "course(): " ); // TasksPart2Tests.course(); // System.out.print( "course(): " ); // TasksPart2Tests.course();
System.out.println( " Trying inOrder() " );
double x1 = 3.0; double x2 = 3.5; double y1 = 1.5; double y2 = 4.5; double z1 = 5.5; double z2 = 7.5;
// boolean b3 = TasksPart2Tests.inOrder( x1, y1, z1 ); // boolean b4 = TasksPart2Tests.inOrder( x2, y2, z2 );
// System.out.println( "TasksPart2Tests.inOrder( " + x1 + ", " + y1 + ", " + z1 // + " ): " + b3 ); // System.out.println( "TasksPart2Tests.inOrder( " + x2 + ", " + y2 + ", " + z2 // + " ): " + b4 );
System.out.println( " Trying mp3Sizer() " );
int s1 = 2000; int s2 = 1350; int m1 = 25; int m2 = 50; int j1 = 1500; int j2 = 4000;
// int g1 = TasksPart2Tests.mp3Sizer( s1, m1, j1 ); // int g2 = TasksPart2Tests.mp3Sizer( s2, m2, j2 );
// System.out.println( "TasksPart2Tests.mp3Sizer( " + s1 + ", " + m1 + ", " + j1 // + " ): " + g1 ); // System.out.println( "TasksPart2Tests.mp3Sizer( " + s2 + ", " + m2 + ", " + j2 // + " ): " + g2 );
System.out.println( " Trying count() " );
// uncomment next four lines after completing count() // int b1 = TasksPart2Tests.count( 1.5, 0.9, 500 ); // int b2 = TasksPart2Tests.count( 2.0, 1.0, 25 ); // System.out.println( "TasksPart2Tests.count( 1.5, 0.9, 500 ): " + b1 + " beans" ); // System.out.println( "TasksPart2Tests.count( 2.0, 1.0, 25 ): " + b2 + " beans" );
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
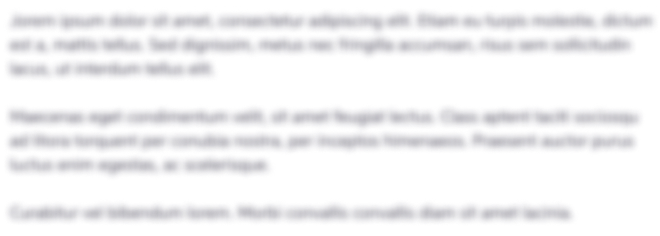
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started