Question
Create a program in Java Language: Program 2: Restaurant Bill In this assignment you will be calculating the final bill at a restaurant. External Class
Create a program in Java Language:
Program 2: Restaurant Bill
In this assignment you will be calculating the final bill at a restaurant.
External Class Requirements:
Create an External Class and name it ComputeBill.
Add the following global instance data:
Create a constant and name it: TAX_RATE.
The constant will be a double.
Initialize the constant 7.5 percent.
See Module 2 Lecture 2 on how to create a constant.
Create another constant and name it: TIP_RATE.
The constant will be a double.
Initialize the constant at 18 percent.
Create a global variable for the tip amount.
This will be a double.
Name it: tip.
Make this variable private.
Create a global variable for the tax amount.
This will be a double.
Name it: tax.
Make this variable private.
Create a global variable for the cost of the meal.
This will be a double.
Name it: mealCost.
Make this variable private.
Create a global variable for the total of the bill.
This will be a double.
Name it: totalBill.
Make this variable private.
Create a Constructor that will initialize the initial cost of the meal.
There is no datatype nor is it void for a constructor.
The constructor receives the cost of the meal through the parameter list.
The constructor will take the initial cost of the meal sent through the parameter list and assign it to the global variable mealCost.
See Module 4 Lecture 5 on how to do this.
Create a method that will calculate the tip amount (before taxes).
Use the constant TIP_RATE that you created.
The datatype of the method will be void. It will not return the tip. It will assign the tip to the global variable tip.
This method will use the global variable mealCost that was initialized by the constructor to compute the tip.
Create a method that will calculate the amount of taxes.
Use the constant TAX_RATE that you created.
The datatype of the method will be void. It will not return the tax. It will assign the tax to the global variable tax.
This method will use the global variable mealCost that was initialized by the constructor to compute the tax.
Create a method that will calculate the total of the bill (includes the taxes, the tip, and the cost of the meal).
Use the variables tax and tip and mealCost to compute the total bill.
Assign the result to the global variable totalBill.
The datatype of the method will be void.
Do not return the total bill through the method name.
7. Create a get and set method for the tip, tax, mealCost, and totalBill.
Driver Class Requirements:
Create a Driver Class and name it RestaurantBill.
This Class will contain the main method.
Create a new object of Class ComputeBill.
Name this object dinner1.
Send 45.00 as the cost of the meal through the parameter list of the constructor.
Hard-code the 45.00 in the parameter list.
Add the following global instance data:Create a variable to hold the cost of the meal.
This variable will be a double.
Name this variable mealPrice.
Create a variable to hold the tax.
This variable will be a double.
Name this variable mealTax.
Create a variable to hold the tip.
This variable will be a double.
Name this variable mealTip.
Call the method to compute the tip.
Use the name of the object: dinner1
Remember, the method will not return anything.
Remember, the cost of the meal has already been sent to the object dinner1 when you created it.
Call the method to compute the tax.
Use the name of the object: dinner1
Remember, the method will not return anything.
Remember, the cost of the meal has already been sent to the object dinner1 when you created it.
Call the method to compute the total cost of the meal.
Use the name of the object: dinner1
Remember, the method will not return anything.
Remember, the cost of the meal has already been sent to the object dinner1 when you created it.
Display the initial cost of the meal using the get method.
Display the amount of the tip using the get method.
Display the amount of the taxes using the get method.
Display the total cost of the meal using the get method.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
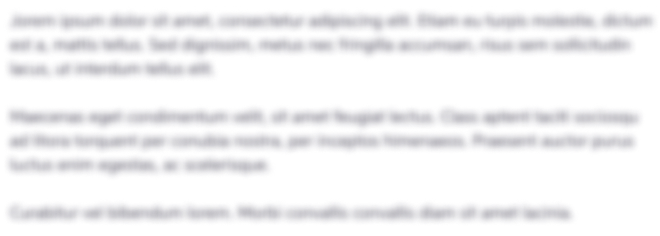
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started