Question
Create a program that prints a class roll sheet in alphabetical order. The user inputs the students' first name and last names (separately!), and presses
Create a program that prints a class roll sheet in alphabetical order. The user inputs the students' first name and last names (separately!), and presses enter on the first name when done. The program prints out the roster like this...
HATFIELD ELIZABETH,
HEIDI KAISER,
RUSSELL LIPSHUTZ,
HOWARD PENKERT,
DAWN WRIGHT,
Before starting, carefully study sort_str(), mod_str(), and format(). Make a copy of sort_str() and rename it roll(). Also, change stsrt() to stsrt2(). Compile and make sure it works from main(). Change limit[] to represent first name. The input should work for 30 students and first name should be 15 characters. Change prompts as needed. Compile and test. Make changes to convert the first name to all upper case using a function from mod_str(). Add another array and get input for last name which will also be an array of 30 with 15 characters. Combine last and first into an third array. You may use sprintf() for this one. There are other ways.
these are the programs that i am referring to when i am saying "sort_str(), mod_str(), and format()"...
/* mod_str.c -- modifies a string */ #include #include #include // need the ctype header file because it is part of toupper #define LIMIT 81 void ToUpper(char *); int PunctCount(const char *);
void mod_str(void) { char line[LIMIT]; char * find; puts("Please enter a line:"); fgets(line, LIMIT, stdin); find = strchr(line, ' '); // look for newline if (find) // if the address is not NULL, *find = '\0'; // place a null character there ToUpper(line); puts(line); printf("That line has %d punctuation characters. ", PunctCount(line)); }
void ToUpper(char * str) { while (*str) //it is checking for the location for the first character. { *str = toupper(*str); str++; } }
int PunctCount(const char * str) { int ct = 0; while (*str) { if (ispunct(*str)) ct++; str++; } return ct; }
/* format.c -- format a string */ #include #define MAX 20 char * s_gets(char * st, int n);
void format(void) { char first[MAX]; char last[MAX]; char formal[2 * MAX + 10]; double prize; puts("Enter your first name:"); s_gets(first, MAX); puts("Enter your last name:"); s_gets(last, MAX); puts("Enter your prize money:"); scanf("%lf", &prize); sprintf(formal, "%s, %-19s: $%6.2f ", last, first, prize); puts(formal); } /* char * s_gets(char * st, int n) { char * ret_val; int i = 0; ret_val = fgets(st, n, stdin); if (ret_val) { while (st[i] != ' ' && st[i] != '\0') i++; if (st[i] == ' ') st[i] = '\0'; else // must have words[i] == '\0' while (getchar() != ' ') continue; } return ret_val; } */
/* sort_str.c -- reads in strings and sorts them */ #include #include #define SIZE 15 /* string length limit, including \0 */ #define LIM 30 /* maximum number of lines to be read */ #define HALT "" /* null string to stop input */ void stsrt(char *strings[], int num);/* string-sort function */ char * s_gets(char * st, int n);
void sort_str(void) { char input[LIM][SIZE]; /* array to store input */ char *ptstr[LIM]; /* array of pointer variables */ int ct = 0; /* input count */ int k; /* output count */ printf("Input up to %d lines, and I will sort them. ",LIM); printf("To stop, press the Enter key at a line's start. "); while (ct < LIM && s_gets(input[ct], SIZE) != NULL && input[ct][0] != '\0') { ptstr[ct] = input[ct]; /* set ptrs to strings */ ct++; } stsrt(ptstr, ct); /* string sorter */ puts(" Here's the sorted list: "); for (k = 0; k < ct; k++) puts(ptstr[k]) ; /* sorted pointers */ }
/* string-pointer-sorting function */ void stsrt(char *strings[], int num) //DO NOT TOUCH THIS CODE!! { char *temp; int top, seek; for (top = 0; top < num-1; top++) for (seek = top + 1; seek < num; seek++) if (strcmp(strings[top],strings[seek]) > 0) { temp = strings[top]; strings[top] = strings[seek]; strings[seek] = temp; } } /* char * s_gets(char * st, int n) { char * ret_val; int i = 0; ret_val = fgets(st, n, stdin); if (ret_val) { while (st[i] != ' ' && st[i] != '\0') i++; if (st[i] == ' ') st[i] = '\0'; else // must have words[i] == '\0' while (getchar() != ' ') continue; } return ret_val; } */
/*
* Chapter11: chapter11b.c - Second half of chapter 11
* Julie Luscomb - Oct 1, 2017, 1:32:47 PM
*/
#include
char * s_gets(char * st, int n); // function included at end of this file
int menu(void); //prototype definition
void test_fit(void);
void str_cat(void); //first occurrence of s_gets()
void join_chk(void);
void nogo(void);
void compare(void);
void compback(void);
void quit_chk(void);
void starsrch(void);
void copy1(void);
void copy2(void);
void copy3(void);
void format(void);
void sort_str(void);
void mod_str(void);
//void repeat(void);
//void hello(void);
void strcnvt(void);
int main(void)
{
int selection = menu(); //variable declaration and initialization via call to menu()
//The line above also serves as the 'priming' read for the following while loop
while(selection != 99) {
// The switch statement is similar to a chained if-else except conditions fall through in C!
switch(selection) {
case 1:
//test_fit();
break;
case 2:
// str_cat();
break;
case 3:
//join_chk();
break;
case 4:
//nogo();
break;
case 5:
// compare();
break;
case 6:
//compback();
break;
case 7:
//quit_chk();
break;
case 8:
//starsrch();
break;
case 9:
//copy1();
break;
case 10:
//copy2();
break;
case 11:
//copy3();
break;
case 12:
//format();
break;
case 13:
sort_str();
break;
case 14:
//mod_str();
break;
case 15:
//repeat(); new project, compile and run from command line
break;
case 16:
//hello(); new project, type program, compile and run from command line
break;
case 17:
//strcnvt();
break;
case 18:
break;
case 19:
break;
default: /* Optional - but a good idea expecially if you have no other error catching! */
printf("Please enter a valid selection. ");
}
selection = menu(); // get the next menu selection, otherwise, you have an eternal loop!
}
return 0;
}
int menu(void) {
int choice = 99;
printf("*************************** ");
printf(" 1. test_fit ");
printf(" 2. str_cat ");
printf(" 3. join_chk ");
printf(" 4. nogo ");
printf(" 5. compare ");
printf(" 6. compback ");
printf(" 7. quit_chk ");
printf(" 8. starsrch ");
printf(" 9. copy1 ");
printf("10. copy2 ");
printf("11. copy3 ");
printf("12. format ");
printf("13. sort_str ");
printf("14. mod_str ");
printf("15. Repeat: Use new project, compile and run from prompt! ");
printf("16. Hello: Use new project, compile and run from prompt! ");
printf("17. strcnvt ");
printf("18. ");
printf("19. ");
printf("99. Exit ");
printf("Please select number and press enter: ");
printf("*************************** ");
scanf("%d", &choice);
getchar();
return choice;
}
/*Put this function ONE place and then include the prototype for each file
It is better to use header files - but this approach taken to make things more clear. */
char * s_gets(char * st, int n)
{
char * ret_val;
int i = 0;
ret_val = fgets(st, n, stdin);
if (ret_val)
{
while (st[i] != ' ' && st[i] != '\0')
i++;
if (st[i] == ' ')
st[i] = '\0';
else // must have words[i] == '\0'
while (getchar() != ' ')
continue;
}
return ret_val;
}
i hope this is enough information!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
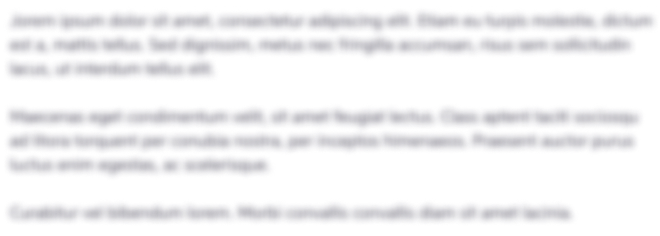
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started