Question
Create a UML Diagram for the following code import java.util.*; import java.util.Queue; // Class for Min Queue Data Structure class MinQueue { Queue Q =
Create a UML Diagram for the following code
import java.util.*;
import java.util.Queue;
// Class for Min Queue Data Structure
class MinQueue {
Queue
Deque
public void enque_element(int element) { // Function to push element into the queue
if(Q.size() == 0) { // Check if there is no element in the queue
Q.add(element);
D.add(element);
}
else {
Q.add(element);
while(D.size() != 0 && D.peekLast() > element) { //Remove the elements out until the element at back is greater than current element
D.removeLast(); // Remove from last of the Deque
}
D.add(element);
}
}
public void deque_element() { // Function to remove the element out from the queue
if(Q.peek() == D.peekFirst()) { // Check if the Minimum element is the element at the front of the Deque
Q.remove();
D.removeFirst(); // remove from head of the Deque
}
else {
Q.remove();
}
}
public int getMin() { // Function to get the minimum element from the queue
return D.peekFirst(); // Insert in the front
}
}
public class MainClass {
public static void main(String args[]) {
MinQueue k = new MinQueue();
int[] arr = new int[]{ -2, 1,2,4,677,0, 18};
for(int i=0; i<7; i++) { // Loop to enque element
k.enque_element(arr[i]);
}
System.out.println("Min element: " + k.getMin());
System.out.println("Initial Queue " + k.Q);
k.deque_element();
System.out.println("After 1 iteration-> Queue " + k.Q);
System.out.println("Min element: " + k.getMin());
System.out.println("SIZE of the queue is " + k.Q.size());
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
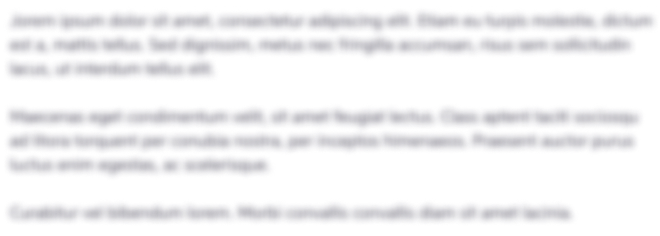
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started