Question
Create an AVL Tree as a C++ class called AVLTree. Implement smart pointers weak_ptr and shared_ptr, which are built to help to manage memory. Abide
Create an AVL Tree as a C++ class called AVLTree. Implement smart pointers weak_ptr and shared_ptr, which are built to help to manage memory.
Abide by the following rules if you wish to modify my BST tree to implement your AVL tree in C++:
If v is a parent of u, then u has a std::weak_ptr that points to v.
If w is a child of u, then u has a std::shared_ptr that points to w.
Use std::shared_ptr to modify what is being pointed to. In order to do this for a parent, you must convert a std::weak_ptr to a std::shared_ptr using lock.
To check for an empty smart pointer, compare a std::shared_ptr to nullptr.
A std::weak_ptr may not be assigned nullptr; instead, use reset to have a std::weak_ptr "point to null".
Implement the following as well:
- Insert Implement an Insert function that utilizes the rotations as described at Geeks- ForGeeks: https://www.geeksforgeeks.org/?p=17679
- Deletion Implement a Delete function as described at GeeksForGeeks: https://www.geeksforgeeks.org/avl-tree-set-2-deletion/ Note that the article links to BST deletion: https://www.geeksforgeeks.org/binary-search-tree-set-2-delete/ When a node is deleted in a BST, if it has two children, either the inorder successor or the inorder predecessor is copied. Either choice is fine for this programming assignment.
- DeleteMin Implement a DeleteMin function which deletes the minimum key in the AVL tree. Any implementation that runs in O(log n) time is acceptable.
The input will be in the form of a JSON file, and output should be printed to the screen.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
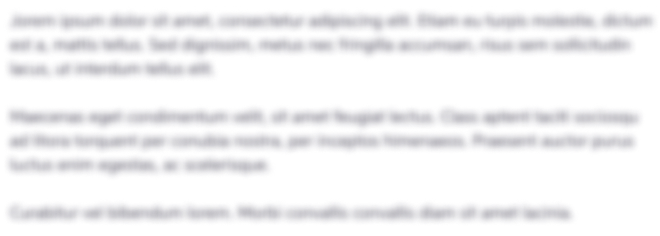
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started