Question
create an index for the complete works of William Shakespeare (pg100.txt), although you can use it on other files. Please check below for instructions and
create an index for the complete works of William Shakespeare (pg100.txt), although you can use it on other files. Please check below for instructions and follow them. And use JAVA to code this.
We will create an IndexTree, a special type of Binary Search Tree. The IndexTree does a bit more than your standard tree, as we will use it to build an index of a le. This will be much like the index you nd at the end of a textbook, where each topic is listed in alphabetical order with the pages it is found on. Since les don't have traditional pages to work o of, we will instead use line numbers. Furthermore, rather than building an index of only a few select topics, we will build an index over all the words in the le. To do this, we use a special type of node. The IndexTree is made up of special IndexNodes. Rather than using generics, each IndexNode stores a word, the count of occurrences of that word, and a list of all lines that word appeared on (this means that each IndexNode will hold their own list). Nodes in the tree will be sorted by the String. Use an IndexTree object to store an index of all the words that are in the provided text le, then display the index by performing an inorder traversal of the tree.
A main object template has already made to read the file. Choose any text file is OK. There is also template for other parts. So get the file reading working first, then work on IndexTree.
Here is the template.
public class IndexNode { // The word for this entry String word; // The number of occurrences for this word int occurences; // A list of line numbers for this word. List
// Your class. Notice how it has no generics. // This is because we use generics when we have no idea what kind of data we are getting // Here we know we are getting two pieces of data: a string and a line number public class IndexTree { // This is your root // again, your root does not use generics because you know your nodes // hold strings, an int, and a list of integers private IndexNode root; // Make your constructor // It doesn't need to do anything // complete the methods below // this is your wrapper method // it takes in two pieces of data rather than one // call your recursive add method public void add(String word, int lineNumber){ } // your recursive method for add // Think about how this is slightly different the the regular add method // When you add the word to the index, if it already exists, // you want to add it to the IndexNode that already exists // otherwise make a new indexNode private IndexNode add(IndexNode root, String word, int lineNumber){ return null; } // returns true if the word is in the index public boolean contains(String word){ return false; } // call your recursive method // use book as guide public void delete(String word){ }
// your recursive case // remove the word and all the entries for the word // This should be no different than the regular technique. private IndexNode delete(IndexNode root, String word){ return null; } // prints all the words in the index in inorder order // To successfully print it out // this should print out each word followed by the number of occurrences and the list of all occurrences // each word and its data gets its own line public void printIndex(){ } public static void main(String[] args){ IndexTree index = new IndexTree(); // add all the words to the tree // print out the index // test removing a word from the index } }
import java.io.*; import java.util.Scanner; public class ReadingFromFileExample { public static void main(String [] args) { String fileName = "pg2240.txt"; try { Scanner scanner = new Scanner(new File(fileName)); while(scanner.hasNextLine()){ String line = scanner.nextLine(); System.out.println(line); //String[] words = line.split("\\s+"); //for(String word : words){ // word = word.replaceAll(":", ""); // word = word.replaceAll(",", ""); // System.out.println(word); //} } scanner.close(); } catch (FileNotFoundException e1) { // TODO Auto-generated catch block e1.printStackTrace(); } } }
Please be sure create the index and read the file based on the templates and requirement.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
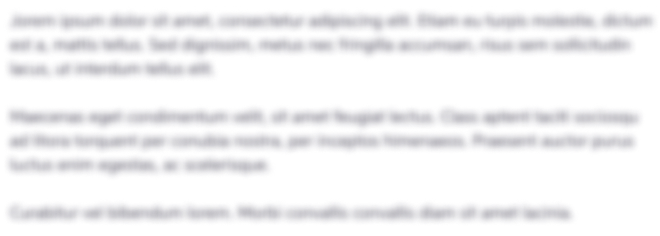
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started