Question
Create code that implements the following: add creative element. There should be two main parts to this: Decide on a theme for the game. FInd
Create code that implements the following:
add creative element. There should be two main parts to this:
Decide on a theme for the game. FInd images to support this theme. Use them for the player, enemies, and powerups.
Think of a fun variation on the basic game to make it more interesting to play. This should not be a one line change (like change the number in the wait to make it go faster). My guess is that you should have between 10-100 lines of code to do something worthwhile, but that is just a rough guide. It should show insight and caring about the impact on the game play. Partner projects should expect to do a little more here. I am deliberately not giving much guidance here so as to not influence your creative choices
import pygame, sys, math, random
# Test if two sprite masks overlap def pixel_collision(mask1, rect1, mask2, rect2): offset_x = rect2[0] - rect1[0] offset_y = rect2[1] - rect1[1] # See if the two masks at the offset are overlapping. overlap = mask1.overlap(mask2, (offset_x, offset_y)) return overlap
# A basic Sprite class that can draw itself, move, and test collisions class Sprite: def __init__(self, image): self.image = image self.rectangle = image.get_rect() self.mask = pygame.mask.from_surface(image)
def set_position(self, new_position): self.rectangle.center = new_position
def draw(self, screen): screen.blit(self.image, self.rectangle)
def is_colliding(self, other_sprite): return pixel_collision(self.mask, self.rectangle, other_sprite.mask, other_sprite.rectangle)
class Enemy: def __init__(self, image, width, height): self.image = image self.mask = pygame.mask.from_surface(image) self.rectangle = image.get_rect()
# Add code to # 1. Set the rectangle center to a random x and y based # on the screen width and height # 2. Set a speed instance variable that holds a tuple (vx, vy) # which specifies how much the rectangle moves each time. # vx means "velocity in x".
def move(self): print("need to implement move!") # Add code to move the rectangle instance variable in x by # the speed vx and in y by speed vy. The vx and vy are the # components of the speed instance variable tuple. # A useful method of rectangle is pygame's move_ip method. # Research how to use it for this task.
def bounce(self, width, height): print("need to implement bounce!") # This method makes the enemy bounce off of the top/left/right/bottom # of the screen. For example, if you want to check if the object is # hitting the left side, you can test # if self.rectangle.left < 0: # The rectangle.left tests the left side of the rectangle. You will # want to use .right .top .bottom for the other sides. # The height and width parameters gives the screen boundaries. # If a hit of the edge of the screen is detected on the top or bottom # you want to negate (multiply by -1) the vy component of the speed instance # variable. If a hit is detected on the left or right of the screen, you # want to negate the vx component of the speed. # Make sure the speed instance variable is updated as needed.
def draw(self, screen): # Same draw as Sprite screen.blit(self.image, self.rectangle)
class PowerUp: def __init__(self, image, width, height): # Set the PowerUp position randomly like is done for the Enemy class. # There is no speed for this object as it does not move. self.image = image self.mask = pygame.mask.from_surface(image) self.rectangle = image.get_rect()
def draw(self, screen): # Same as Sprite screen.blit(self.image, self.rectangle)
def main(): # Setup pygame pygame.init()
# Get a font for printing the lives left on the screen. myfont = pygame.font.SysFont('monospace', 24)
# Define the screen width, height = 600, 400 size = width, height screen = pygame.display.set_mode((width, height))
# Load image assets # Choose your own image enemy = pygame.image.load("GolfBall.png").convert_alpha() # Here is an example of scaling it to fit a 50x50 pixel size. enemy_image = pygame.transform.smoothscale(enemy, (50, 50))
enemy_sprites = [] # Make some number of enemies that will bounce around the screen. # Make a new Enemy instance each loop and add it to enemy_sprites.
# This is the character you control. Choose your image. player_image = pygame.image.load("Wizard.gif").convert_alpha() player_sprite = Sprite(player_image) life = 3
# This is the powerup image. Choose your image. powerup_image = pygame.image.load("Knight.gif").convert_alpha() # Start with an empty list of powerups and add them as the game runs. powerups = []
# Main part of the game is_playing = True # while loop while is_playing:# while is_playing is True, repeat # Modify the loop to stop when life is <= to 0.
# Check for events for event in pygame.event.get(): # Stop loop if click on window close button if event.type == pygame.QUIT: is_playing = False
# Make the player follow the mouse pos = pygame.mouse.get_pos() player_sprite.set_position(pos)
# Loop over the enemy sprites. If the player sprite is # colliding with an enemy, deduct from the life variable. # A player is likely to overlap an enemy for a few iterations # of the game loop - experiment to find a small value to deduct that # makes the game challenging but not frustrating.
# Loop over the powerups. If the player sprite is colliding, add # 1 to the life.
# Make a list comprehension that removes powerups that are colliding with # the player sprite.
# Loop over the enemy_sprites. Each enemy should call move and bounce.
# Choose a random number. Use the random number to decide to add a new # powerup to the powerups list. Experiment to make them appear not too # often, so the game is challenging.
# Erase the screen with a background color screen.fill((0,100,50)) # fill the window with a color
# Draw the characters for enemy_sprite in enemy_sprites: enemy_sprite.draw(screen) for powerup_sprite in powerups: powerup_sprite.draw(screen)
player_sprite.draw(screen)
# Write the life to the screen. text = "Life: " + str('%.1f'%life) label = myfont.render(text, True, (255, 255, 0)) screen.blit(label, (20, 20))
# Bring all the changes to the screen into view pygame.display.update() # Pause for a few milliseconds pygame.time.wait(20)
# Once the game loop is done, pause, close the window and quit. # Pause for a few seconds pygame.time.wait(2000) pygame.quit() sys.exit()
if __name__ == "__main__": main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
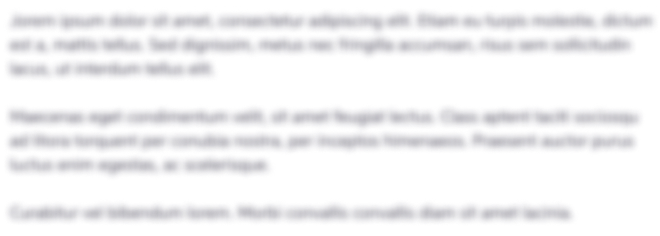
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started