Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Need Help with C++ Project. NEED HELP CREATING AND IMPLEMENTING CLASSES Develop C++ classes that could be used to create a room simulator. Create two
Need Help with C++ Project. NEED HELP CREATING AND IMPLEMENTING CLASSES
Develop C++ classes that could be used to create a room simulator. Create two classes: one to represent a seat and the other to represent a room. These classes can be used to create a room simulator, which will not be created as part of this assignment.
Requirements:
provided with a test suite implementation must pass all tests provided in this test suite:
- The test suites are:
- test-seat - This tests the functions of a seat object.
- test-room1 - This tests some functions of a room object.
- test-room2 - This tests some other functions of a room object. Room test suite is broken into two sets for grading purposes.
- Running all of these tests must be possible by running make test-all.
- Your project will lose points if memory leaks exist.
UML of the classes
Make File:
CXX = g++ | |
CXXFLAGS = -g -std=c++11 -Wall | |
test-all: test-seat test-room1 test-room2 | |
test-seat: test/test-seat.o seat.cpp | |
$(CXX) $(CXXFLAGS) -o test/$@ $^ | |
test/$@ --success | |
test-room1: test/test-room1.o room.cpp seat.cpp | |
$(CXX) $(CXXFLAGS) -o test/$@ $^ | |
test/$@ --success | |
test-room2: test/test-room2.o room.cpp seat.cpp | |
$(CXX) $(CXXFLAGS) -o test/$@ $^ | |
test/$@ --success | |
clean: | |
rm -rf *.dSYM | |
$(RM) *.o *.gc* test/*.o test/test-seat test/test-room1 test/test-room2 core main | |
Test_Room_1.cpp
#define CATCH_CONFIG_MAIN | |
#include "catch/catch.hpp" | |
#include "../room.hpp" | |
#include | |
TEST_CASE("Testing constructors") | |
{ | |
Room r1; // defualt should create 3x3 room | |
std::ostringstream s; | |
s << r1; | |
CHECK("o o o o o o o o o " == s.str()); | |
s.str(""); | |
Room r2(2,4); | |
s << r2; | |
CHECK ("o o o o o o o o " == s.str()); | |
} | |
TEST_CASE("Testing Room object") | |
{ | |
Room r1; | |
std::ostringstream s; | |
bool ret = false; | |
ret = r1.BookSeat(1,1); // book seat within range | |
CHECK(ret); | |
s << r1; | |
CHECK ("o o o o x o o o o " == s.str()); | |
ret = r1.BookSeat (4,0); // book seat outside range; | |
CHECK(!ret); | |
ret = r1.BookSeat (-1,2); // book seat with negative index | |
CHECK(!ret); | |
ret = r1.FreeSeat(1,1); // 1,1 has been booked before | |
CHECK(ret); | |
ret = r1.FreeSeat (-1,0); | |
CHECK(!ret); | |
} |
Test_Room_2.cpp
#define CATCH_CONFIG_MAIN | |
#include "catch/catch.hpp" | |
#include "../room.hpp" | |
#include | |
TEST_CASE("Testing Room object") | |
{ | |
Room r1; | |
std::ostringstream s; | |
bool ret = false; | |
ret = r1.ShrinkRoom(4,3); // provide bigger dimensions. | |
CHECK(!ret); | |
Room r2; | |
ret = r2.ShrinkRoom(2,2); | |
CHECK (ret); | |
s.str(""); | |
s << r2; | |
CHECK("o o o o " == s.str()); | |
Room r3(2,5); | |
CHECK(Approx(0.0) == r3.Occupancy()); | |
r3.BookSeat(0,0); | |
r3.BookSeat(0,1); | |
CHECK(Approx(0.2) == r3.Occupancy()); | |
r3.BookSeat(0,0); // book an already booked seat | |
CHECK(Approx(0.2) == r3.Occupancy()); | |
r3.BookSeat (1,0); | |
CHECK(Approx(0.3) == r3.Occupancy()); | |
} |
Test_Seat.cpp
#define CATCH_CONFIG_MAIN | |
#include "catch/catch.hpp" | |
#include "../seat.hpp" | |
TEST_CASE("Testing constructors") | |
{ | |
Seat s1; | |
CHECK(!s1.GetStatus()); | |
Seat s2 (true); | |
CHECK(s2.GetStatus()); | |
Seat s3(false); | |
CHECK(!s3.GetStatus()); | |
} | |
TEST_CASE("Testing Seat object") | |
{ | |
Seat s(false); | |
bool ret; | |
ret = s.Free(); // freeing an already free seat! | |
CHECK(!ret); | |
ret = s.Book(); // booking a free seat | |
CHECK(ret); | |
CHECK(s.GetStatus()); | |
ret = s.Book(); // booking a booked seat! | |
CHECK(!ret); | |
ret = s.Free(); // freeing a booked seat! | |
CHECK(ret); | |
CHECK(!s.GetStatus()); | |
} | |
Step by Step Solution
★★★★★
3.49 Rating (149 Votes )
There are 3 Steps involved in it
Step: 1
The room simulator creating two classes 1 Room 2 Seat Then list the functions or methods you want to ...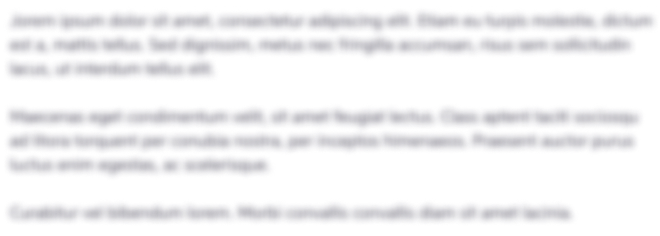
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started