Question
CS 201 Excerise 10 Part 1 Build a class dynListType based on dynamic array. Its declaration is provided below: // no more MAX class dynListType
CS 201 Excerise 10
Part 1
Build a class dynListType based on dynamic array. Its declaration is provided below:
// no more MAX class dynListType { public: private: int* dataPtr; int size; int maxSize; }; |
Follow example in Unit 6 PPT 1 (ch12-ch13 Classes with Dynamic Data), add:
a (default) constructor with an int param (default value 5) and
a destructor.
Add those 10 functions from HW9 into dynListType. Be sure to change each occurrence of dataArr (HW9) into dataPtr. Make additional necessary changes.
getSize()
getMaxSize()
isEmpty()
search()
at()
two inserts
remove()
operator +
operator
At this point your program should be able to compile, but may crash during execution. Thats because copy constructor and overloaded assignment are required to handle dynamic data members.
Now add:
Copy constructor
Overloaded assignment operator =
Your program should work perfectly now. dynListType provides the same functionality listType (HW9) does, except that dynListType doesnt have any capacity cap (i.e. there is no MAX for maxSize).
Test your class thoroughly before proceeding to Part 2.
Part 2
Modify your dynListType program from Part 1.
Overload == operator (comparison operator, as in if (obj1 == obj2) ): return true if two list objects contain the same data items in a same order. Values of maxSize dont matter. For example, given obj1 {maxSize 10, } and obj2 {maxSize 5, }, the comparison should return true. Given obj3 {maxSize 5, }, (obj1 == obj3) should be false.
Modify the two insert functions so a list will never be full, like how Java ArrayList and the STL vector class work. Whenever an insertion reaches the maxSize cap, double maxSize, and reallocate storage space. Here is the suggested pseudo code:
If size before insertion is equal to maxSize // current maxSize reached maxSize allocate a new dynamic array with maxSize slots copy data pointed by dataPtr over to new array delete the dynamic array currently pointed by dataPtr let dataPtr point to the newly allocated dynamic array End if Insert as normal |
Modify your driver accordingly to test the new/modified functions. Here is the output of my sample driver. I hard-coded data in the driver and generate some data using loops. You may do it differently, as long as it tests EACH new/modified function
Organize your program into header file (interface), implementation file, and driver file. Use #include guard with the header file.
LISTYPE HW9
const int MAX = 100; // max capacity for all listType objects class listType { public: listType(int max = 5); // constructor // Post: maxSize MAX, MAX will be used // size int getSize() const { return size; } // return # of elements actually stored int getMaxSize() const { return maxSize; } // return capacity bool isEmpty() const { return size == 0; } bool isFull() const { return size == maxSize; } int search(int element) const; // look for an item. return index of first occurrence int at(int index) const; // return element at a specific location void print() const; // print content of list on screen in format of [a, b, c] (like what ArrayList in Java does) bool insert(int element); // append/insert an element at the end bool insert(int index, int element); // insert an element into location index. // Shifts the element currently at that index (if any) and any subsequent elements to the right bool remove(int index); // remove element at the specified location private: int dataArr[MAX]; // static array storing data items int size; // actual # of elements stored. size int maxSize; // The capacity of this listType obj. 0 }; |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
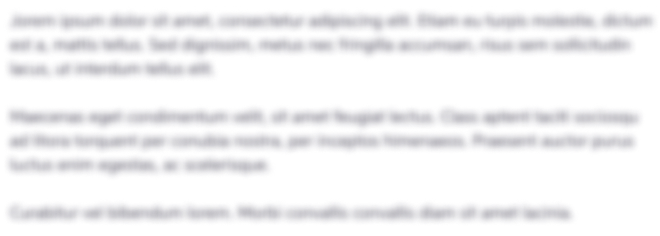
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started