Question
CS 2050 Programming Project # 1 In this project, you create two classes for tracking students. One class is the Student class that holds student
CS 2050 Programming Project # 1
In this project, you create two classes for tracking students. One class is the Student class that holds student data; the other is the GradeItem class that holds data about grades that students earned in various courses.
Note: the next three projects build on this one. To keep pace in the course, you should complete this project in full by the due date. Ask questions if youre stuck!
Use Javadoc parameters @author, @version, @param and @return, as needed.
Problem Scenario
State University needs a Java programmer to assist it with creating a grade book application. You have graciously volunteered to help. The university wants to track their students and their grades in various courses. For each student, the university wants to track this information:
- Student id (String a unique value for each student)
- Student first name (String)
- Student last name (String)
- Student email address (String a unique value for each student)
The university wants to make sure that they have a correct email address for each student. They want you to verify there is an @ in the email address.
For each grade item, the university wants to track this information:
- Student id (String should match a student in the student list)
- GradeItem id (Integer a unique value for each grade item)
- Course id (String)
- Item type (String must be one of the following: HW, Quiz, Class Work, Test, Final)
- Date (String format yyyymmdd)
- Maximum score (Integer must be greater than 0)
- Actual score (Integer must be in the range of 0 to maximum score)
Program Requirements
Create two classes for the problem scenario given above. The classes are:
- A Student class
- A GradeItem class
These two classes are used in subsequent projects to instantiate the Student and GradeItem objects, and do not contain a main method or any input/output code. After declaring your instance variables, your Student and GradeItem classes should each contain these methods in this order:
- Constructor
- Get methods for each private variable (getters)
- An equals method to determine whether two instances of an object contain exactly the same data
- A toString method to display an instance of an object
Note: We assume that our objects are immutable (unchanged once an object is instantiated) so we dont need any set (setter) methods.
Constructors in each class should validate the data as follows:
- In the Student class
- Ensure all values for String fields are not blank. These are the student id, first name, last name and email address.
- Ensure the student email address contains the @ character. Hint: Use the contains method in the String class.
- In the GradeItem class
- Ensure all values for String fields are not blank. These are the student id, course id, item type and date.
- All grade item types must be one of the following: HW, Quiz, Class Work, Test, or Final. Use an array to store the types and search that array for a valid type. The entries are casesensitive (HW does not equal Hw). Do not hard-code the grade item types.
- GradeItem class: Maximum score must be greater than 0.
- GradeItem class: Actual score must be in the range of 0 to maximum score.
If any of the data in either class is invalid, the constructor in which the error was detected must throw the IllegalArgumentException exception with a message that is meaningful to a non-technical user.
Note: The data required to initialize the fields is passed by a method (defined in a subsequent project) to the constructor using arguments. In the Student and GradeItem classes, do not display any error messages on the console or in a window, or use the Scanner class to ask users for input.
Testing
We will write code to test the data in the Student and GradeItem classes in Programming Project # 2. Other than the testing described above, there is no additional testing now.
What to Submit
Submit printed copies of the Student class on top of the GradeItem class and staple them together. Write your name and Project #1 in the upper right-hand corner of the first page. Resubmitting? Place the earlier submission(s) at the end and indicate on the top page of the new submission that you are resubmitting.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
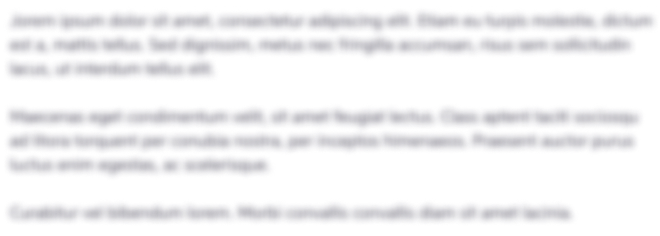
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started