Question
CS/SE 4348 Operating Systems A Very Simple File System File system is an integral part of any operating system. The goal of this project is
CS/SE 4348 Operating Systems
A Very Simple File System
File system is an integral part of any operating system. The goal of this project is to design and implement a very simple file system on a virtual disk. After successful implementation, you would not only have gained significant insight into the intricacies of a file system, but also have experienced building a moderately complex system.
To help you manage your time well, the project is broken down into two parts. Try to finish Part 1 in a weeks time.
Part 1: Virtual Disk
File systems are built on storage devices (disks). But UTD cannot afford to get everyone a disk for this project. So, in this part of the project, you will build a virtual disk on a Linux machine. You will emulate a disk using a very large file. You will divide the file into 4K byte blocks. All the blocks are stored sequentially in the file. So, block number 10 will begin at offset 4096 in the file.
Your virtual disk library should support the following interfaces.
int create_disk(char* filename, size_t nbytes);
This function creates the disk. To be specific, the file ( filename) of length specified by nbytes is created. On success, it returns 0. Otherwise returns -1.
int open_disk(char* filename);
This functions opens the file (specified by filename) for reading and writing. On success, returns the file descriptor of the opened file. Otherwise returns -1.
int read_block(int disk, int block_num, char *buf);
This function reads disk block block_num from the disk pointed to by disk (file descriptor returned by open_disk) into a buffer pointed to by buf. On success it returns 0. Otherwise it returns -1.
int write_block(int disk, int block_num, char *buf);
This function writes the data in buf to disk block block_num pointed to by disk. On success it returns 0. Otherwise returns -1.
int close_disk(int disk)
This function closes the disk. On success returns 0. Otherwise returns -1.
All the above functions should be in file disk.c(cpp) . Note that this source file should be compiled to a library disk.o. Use file disk.h to define relevant constants and data structures for this library.
Part 2: Simple File System (SFS)
SFS consists of two parts: management functions and access functions. The interfaces to management functions are defined next.
int make_sfs(char *disk_name)
This function creates the new file system on the virtual disk specified by disk_name. As part of this function, you should first invoke open_disk() to create a new disk. Then, create (and
initialize) the necessary on disk structures for your file system. The function returns 0 on success, and -1 on failure (when the disk could not be created, opened, or properly initialized.)
int mount_sfs(char *disk_name)
This function mounts the disk (disk_name). The on disk structures are read into memory to handle the file system operations. The function returns 0 on success, and -1 on failure.
int umount_sfs(char *disk_name)
This function unmounts the disk (disk_name) from your file system. All the meta data and file
system related data that are on memory should be wrote into appropriate on disk structures. Finally, it should call close_disk(). The function returns 0 on success, and -1 on failure.
Next, you need to implement the following access routines.
int sfs_open(char *file_name)
The file (file_name) is opened for reading and writing. This function returns the file descriptor
of the file on success and -1 on failure. Note that the same file may opened many times, and for each open call a different file descriptor should be returned. The maximum number of files that can remain opened at a time is specified by constant MAX_OPEN_FILES in the sfs.h header file.
int sfs_close(int fd)
The file descriptor fd is closed. The closed file descriptor can no longer be used to access the
corresponding file. However, the closed file descriptor should be reused during another sfs_open()call. This function returns 0 on success and -1 on failure.
int sfs_create(char *file_name)
The file specified by file_name is created. The file is created only if it is not already present.
The length of the file name is specified by the constant FNAME_LENGTH. The function returns 0 on success and -1 on failure. The number of files that are in a directory is restricted to MAX_FILES.
int sfs_delete(char *file_name)
The file specified by file_name is deleted. The file is deleted only if it has been already created.
On deletion all the data blocks, on disk and on memory data structures related to the file data and meta data are released. Also, the file can be deleted only if it is not open. This function returns 0 on success and -1 on failure.
int sfs_read(int fd, void *buf, size_t count)
sfs_read () attempts to read up to count bytes from file descriptor fd into the buffer starting at buf. The read operation begins at the current file offset, and the file offset is incremented by
the number of bytes read. If the current file offset is at or past the end of file, no bytes are read, and the call returns zero. On success, the number of bytes read is returned (zero indicates end of file), and the file position is advanced by this number. It is not an error if this number is smaller than the number of bytes requested; this may happen because fewer bytes are actually available right now (maybe the current file position is close to end of file). On error, -1 is returned.
int sfs_write(int fd, void *buf, size_t count)
sfs_write () attempts to writes up to count bytes from the buffer buf pointed to the file referred to by the file descriptor fd. The write operation begins at the current file offset, and the
file offset is incremented by the number of bytes written. If end of file is reached while writing,
the file is automatically extended. Upon successful completion, the number of bytes that were actually written is returned. This number could be smaller than count when the disk runs out of
space (when writing to a full disk, the function returns zero). On error, the function returns -1.
int sfs_seek(int fd, int offset)
The function sets the current offset of the file corresponding to file descriptor fd to the value specified by offset. This function returns 0 on success and -1 on failure. It is an error if offset
is larger than the file size.
All the functions should be in file sfs.c(cpp). Note that this source file should be compiled to a library sfs.o. Define relevant constants and data structures for this library in header file sfs.h.
Implementation
You can model your file system using FAT. This is easier to implement when compared to maintaining inode based file system.
SFS need to support only one directory which is the root directory.
The first block of the file system should be super block, and it should contain information pertaining to the file system (block size, number of data blocks, location of FAT, and location of root directory.)
For accessing files, you need to maintain in-memory data structures per process file table and system wide file table. The structure and usage of these tables will be discussed in the class.
The suggested values for the constants are:
BLOCK_SIZE 4096
MAX_BLOCKS 4096
MAX_DATA_BLOCKS 3500
MAX_FILES 64
MAX_OPEN_FILES 64
FNAME_LENGTH 16
Both the source files will not contain any main() function. You are implementing a file system library. Our test case file will have main() with calls to test the functions you have implemented. Of course, you need to write a main() function to test your library by yourself.
Grading Policy
Submit the source files and header files through elearning.
Implementation: 50%. Source code should be structured well with adequate comments clearly showing the different parts and functionalities implemented.
Correctness: 50%.
The TA will compile the code and test it in cs1.utdallas.edu. So, your program should run correctly in cs1.utdallas.edu. Add a note at the top of the source code in case you are using any special flags for compilers/linkers.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
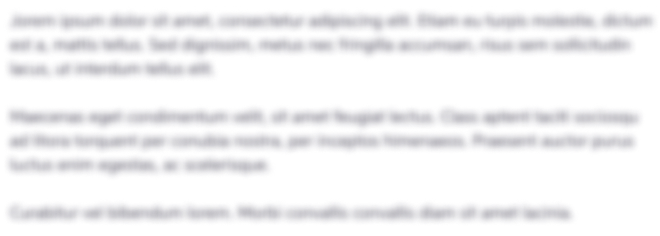
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started