Question
Define a class for rational numbers. A rational number is a number that can be represented as the quotient of two integers. For example, 1/2,
Define a class for rational numbers. A rational number is a number that can be represented as the quotient of two integers. For example, 1/2, 3/4, 64/2, and so forth are all rational numbers.
(By 1/2 we mean the everyday meaning of the fraction, not the integer division this expression would produce in a C++ program.) Represent rational numbers as two values of type int, one for the numerator and one for the denominator. Call the class Rational.
Include a constructor with two arguments that can be used to set the member variables of an object to any legitimate values. Also include a constructor that has only a single parameter of type int; call this single parameter wholeNumber and define the constructor so that the object will be initialized to the rational number wholeNumber/1.
Also include a default constructor that initializes an object to 0 (that is, 0/1).
Overload the relational operators ==, <, <=, >, >=, and != so that they correctly apply to type Rational.
Also write a test program to test your class.
Hints: Two rational numbers a/b and c/d are equal if a*d equals c*b. If b and d are positive rational numbers then a/b is less than c/d provided a*d is less than c*b.
use the header file Rational.h as a starting point
A function to standardize the values stored so that the denominator is always positive should be included. (An additional exercise would be to include a function to reduce the rational number so the numerator and denominator are as small as possible.)
Rational.h:
#ifndef RATIONAL_H
#define RATIONAL_H
#include
using namespace std;
class Rational
{
public:
Rational();
// Initializes the rational number to 0
Rational(int wholeNumber);
// Initializes the rational number to wholeNumber/1
Rational(int m, int n);
// Initializes the rational number to m/n if n is not 0;
// the sign of the rational is stored in the numerator
// (the denominator is always positive);
// exits if n = 0 (invalid rational number)
void output();
// Precondition: The rational number is defined.
// Postcondition: The rational number has been displayed on
// the screen in the form m/n.
friend bool operator ==(const Rational& r1, const Rational& r2);
// Precondition: r1 and r2 are valid rational numbers
// Returns true if r1 equals r2; false otherwise.
friend bool operator <(const Rational& r1, const Rational& r2);
// Precondition: r1 and r2 are valid rational numbers
// Returns true if r1 is less than r2; false otherwise.
friend bool operator >(const Rational& r1, const Rational& r2);
// Precondition: r1 and r2 are valid rational numbers
// Returns true if r1 is greater than r2; false otherwise.
friend bool operator <=(const Rational& r1, const Rational& r2);
// Precondition: r1 and r2 are valid rational numbers
// Returns true if r1 is less than or equal to r2; false otherwise.
friend bool operator >=(const Rational& r1, const Rational& r2);
// Precondition: r1 and r2 are valid rational numbers
// Returns true if r1 is greater than or equal to r2; false otherwise.
friend bool operator !=(const Rational& r1, const Rational& r2);
// Precondition: r1 and r2 are valid rational numbers
// Returns true if r1 is not equal to r2; false otherwise.
private:
int num; // the numerator of the number
int denom; // the denominator of the number
void standardize();
// Precondition: num and denom have values
// Postcondition: if denom is 0 the program is terminated;
// otherwise the rational number is standardized so that
// denom is positive.
};
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
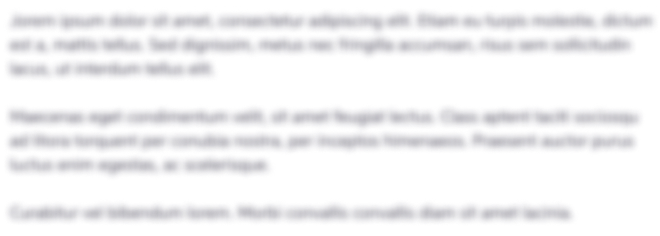
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started