Question
Define a struct that can hold information about accountsFirst: Each instance will hold the name for the account and an account number. We have created
Define a struct that can hold information about accountsFirst:
Each instance will hold the name for the account and an account number.
We have created an input file that has at least three accounts, called accounts.txt.
In main, open and read the file of account information, filling a vector of account objects.
Define local variables corresponding to each data item
Loop through the file, reading into these variables
Each time through the loop, define an instance of the account struct, assign the values that were read to the fields of the struct by name and push back the object onto your vector.
Close the file!
Display all of the objects. Just write this in main, dont worry about defining a function.
Second:
Clear the vector. (Thats the clear method. it sets the size to zero.)
Reopen the file (using the same stream variable, i.e. call the open method)
and repeat the above, except use curly braced initializers to initialize the instance. E.g. if we had a struct Thing with two fields, we could define and initialize an instance with:
Thing thingOne{valueForFirstField, valueForSecondField};
A user of Eclipse reported needing to specify c++14 in their build.
Define a class to hold accountsRedo the above steps from task 1.a that you did with the struct version.
Use the same stream variable!
Obviously, use the classs constructor to initialize the fields
Write getters to access the fields in the accounts when printing.
Add an output operator for your class.Do not make it a friend!
Note it will have to be written outside of the Account class.
And you will need getters for the fields you are displaying.
Repeat the print loop using this output operator
Now make the output operator a friend, by adding a friend prototype to your account class definition.
Do not put the definition of the output operator in the class.
Comment out the line where you are using getters. (Yes, I know we said you shouldnt comment out any code...)
Add a line in the output operator that prints the fields directly, i.e. without using the getters.
Redo your input but pass temporary instances to the push_back method.
This means that you will not define a variable to hold the account, but instead the vector push_back method will be passed a temporary object defined as the argument to push_back.
E.g. If I had a class Foo whose constructor took three ints, and a vector of Foos called vf, I could add an instance of Foo to the vector with: vf.push_back(Foo(2,4,6));
Note that we keep making objects and then adding copies of those objects onto the vector (since thats what push_back does). In this part, we will use the vectors emplace_back method. Instead of passing account objects to emplace_back, we just pass the arguments that we would pass to the accounts constructor and emplace_back will initialize an instance in the vector!
Repeat the filling of the vector using emplace_back and again display the contents.
Add transactions to your world.A transaction will be implemented as a class. Define the class outside of the account class. It will have
a field to indicate whether this is a deposit or a withdrawal.
a field to say how much is being deposited or withdrawn.
An output operator. Define the output operator outside of the transaction class.
The account class will keep track of transactions applied to it. Define a new account class for task 3. It will need
a vector to store this history of transactions.
a balance field to indicate how much is in the account.
How will we use this?The account will have methods deposit and withdrawal which will
be passed the amount
will add an appropriate transaction object to the history
and modify the balance as needed.
Output operators
Define the output operators for both the account class and the transaction class outside of the classes. (Yes, you can make them friends.)
The accounts output operator will need to also display (you format it) the history, i.e. the transactions.
Test the above code out by reading in a file (transactions.txt) that has commands such as
Account moe 6 Deposit 6 10 Account larry 17 Withdraw 6 100
Note that the number 6 in the deposit and withdraw commands refers to the account. You will have to locate the account in your collection of accounts.
Withdrawals should not be allowed to put the account in the red, so if there are insufficient funds in the account then generate an error message and go on.
Now nest the transaction class inside the account class. For convenience, put it in the public section. Yes, define new account and transaction classes for this task.What else will you need to do, besides just moving the Transaction class definition inside?
You should have a little difficulty when defining the output operator for the Transaction class.
Yes you are still defining it outside.
The compiler should complain that there is no such class as Transaction.
That will happen for
the second parameter to the Transactions output operator when you are defining it.
the use of the type Transaction inside the accounts output operator, at least if you are using the ranged for loop there.
Instead, you will have to qualify the name Transaction with the name of the account class it is defined in.
Notice you did not have to do that on the friend declaration inside the Transaction class!!!
Only do this task if you have time in lab
Finally nest the transaction class in the private section of the account class. And, yes, define a new account class for this task.
What else will you need to do, besides just moving the Transaction class from public to private?
Now the compiler will refuse to recognize the qualified transaction parameter for the transactions output operator.
We could move the transactions output operator definition inside the transaction class. Yeah, in some ways its not as clean but it works. We won't do this.
Instead we will mark the Transaction's output operator as a friend of the Account class. Now the definition of the output operator can appear outside of the class definitions, just as in the previous task.
And here is the format that you have to follow:
/*
rec03_start.cpp
*/
// Provided
#include
#include
#include
#include
using namespace std;
// Task 1
// Define an Account struct
// Task 2
// Define an Account class (use a different name than in Task 1)
// Task 3
// Define an Account (use a different name than in Task 1&2) and
// Transaction classes
// Task 4
// Define an Account with a nested public Transaction class
// (use different names than in the previous Tasks)
// Task 5
// Define an Account with a nested private Transaction class
// (use different names than in the previous Tasks)
int main() {
// Task 1: account as struct
// 1a
cout << "Task1a: ";
// 1b
cout << "Task1b: ";
cout << "Filling vector of struct objects, using {} initialization: ";
//==================================
// Task 2: account as class
// 2a
cout << "============== ";
cout << " Task2a:";
cout << " Filling vector of class objects, using local class object: ";
cout << " Task2b: ";
cout << "output using output operator with getters ";
cout << " Task2c: ";
cout << "output using output operator as friend without getters ";
cout << " Task2d: ";
cout << "Filling vector of class objects, using temporary class object: ";
cout << " Task2e: ";
cout << "Filling vector of class objects, using emplace_back: ";
cout << "============== ";
cout << " Task 3: Accounts and Transaction: ";
cout << "============== ";
cout << " Task 4: Transaction nested in public section of Account: ";
cout << "============== ";
cout << " Task 5: Transaction nested in private section of Account: ";
}
//account.txt
moe 6 larry 28 curly 42 //output.txt
Task1a: Filling vector of struct objects, define a local struct instance and set fields explicitly: moe 6 larry 28 curly 42 Task1b: Filling vector of struct objects, using {} initialization: moe 6 larry 28 curly 42 ==============
Task2a: Filling vector of class objects, using local class object: moe 6 larry 28 curly 42 ---
Task2b: output using output operator with getters moe 6 larry 28 curly 42
Task2c: output using output operator as friend without getters moe 6 larry 28 curly 42
Task2d: Filling vector of class objects, using temporary class object: moe 6 larry 28 curly 42
Task2e: Filling vector of class objects, using emplace_back: moe 6 larry 28 curly 42 ==============
Task 3: Accounts and Transaction: Account# 6 has only 10. Insufficient for withdrawal of 100. Account# 28 has only 0. Insufficient for withdrawal of 100. moe 6: deposit 10
larry 28: deposit 10 deposit 10 withdrawal 5
curly 42:
==============
Task 4: Transaction nested in Account: Account# 6 has only 10. Insufficient for withdrawal of 100. Account# 28 has only 0. Insufficient for withdrawal of 100. moe 6: deposit 10
larry 28: deposit 10 deposit 10 withdrawal 5
curly 42:
==============
Task 5: Transaction nested in Account: Account# 6 has only 10. Insufficient for withdrawal of 100. Account# 28 has only 0. Insufficient for withdrawal of 100. moe 6: deposit 10
larry 28: deposit 10 deposit 10 withdrawal 5
curly 42:
//transaction.txt
Account moe 6 Deposit 6 10 Withdraw 6 100
Account larry 28 Withdraw 28 100 Deposit 28 10 Deposit 28 10 Withdraw 28 5
Account curly 42
Step by Step Solution
There are 3 Steps involved in it
Step: 1
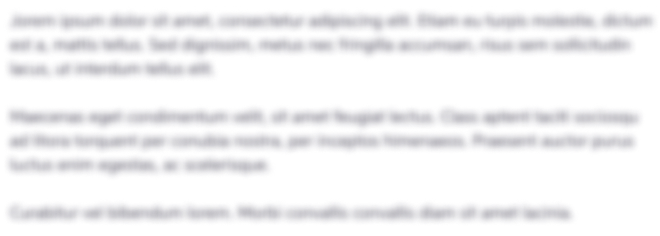
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started