Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Define an interface, BarcodeIO, that contains these method signatures. Any class that implements BarcodeIO is expected to store some version of an image and some
Define an interface, BarcodeIO, that contains these method signatures. Any class that implements BarcodeIO is expected to store some version of an image and some version of the text associated with that image.
public boolean scanBarcodeImage bc;
public boolean readTextString text;
public boolean generateImageFromText;
public boolean translateImageToText;
public void displayTextToConsole;
public void displayImageToConsole;
Now, as I said, this is an interface. So you should be able to do this part of the assignment in less than seconds. I'll time you. Go
Here are the descriptions of what these will do when implemented in the InfoBox class, however, descriptions in an interface don't pack any punch in practice.
public boolean scan BarcodeImage bc accepts some image, represented as a BarcodeImage object to be described below, and stores a copy of this image. Depending on the sophistication of the implementing class, the internally stored image might be an exact clone of the parameter, or a refined, cleaned and processed image. Technically, there is no requirement that an implementing class use a BarcodeImage object internally, although we will do so For the basic InfoBox option, it will be an exact clone. Also, no translation is done here ie any text string that might be part of an implementing class is not touched, updated or defined during the scan.
public boolean readText String text accepts a text string to be eventually encoded in an image. No translation is done here ie any BarcodeImage that might be part of an implementing class is not touched, updated or defined during the reading of the text.
public boolean generateImageFromText Not technically an IO operation, this method looks at the internal text stored in the implementing class and produces a companion BarcodeImage, internally or an image in whatever format the implementing class uses After this is called, we expect the implementing object to contain a fullydefined image and text that are in agreement with each other.
public boolean translateImageToText Not technically an IO operation, this method looks at the internal image stored in the implementing class, and produces a companion text string, internally. After this is called, we expect the implementing object to contain a fully defined image and text that are in agreement with each other.
public void displayTextToConsole prints out the text string to the console.
void displayImageToConsole prints out the image to the console. In our implementation, we will do this in the form of a dotmatrix of blanks and asterisks, eg
Phase : BarcodeImage
This class will realize all the essential data and methods associated with a D pattern, thought of conceptually as an image of a square or rectangular bar code. Here are the essential ingredients. This class has very little "smarts" in it except for the parameterized constructor. It mostly just stores and retrieves D data.
Remember: BarcodeImage implements Cloneable.
DATA
public static final int MAXHEIGHT ; public static final int MAXWIDTH ; The exact internal dimensions of D data.
private boolean imageData This is where to store your image. If the incoming data is smaller than the max, instantiate memory anyway, but leave it blank white This data will be false for elements that are white, and true for elements that are black.
METHODS
Constructors. Two minimum, but you could have others:
Default Constructor instantiates a D array MAXHEIGHT x MAXWIDTH and stuffs it all with blanks false
BarcodeImageString strDatatakes a D array of Strings and converts it to the internal D array of booleans.
HINT This constructor is a little tricky because the incoming image is not the necessarily same size as the internal matrix. So you have to pack it into the lowerleft corner of the double array, causing a bit of stress if you don't like D counting. This is good D array exercise. The InfoBox class will make sure that there is no extra space below or left of the image so this constructor can put it into the lowerleft corner of the array.
Accessor and mutator for each bit in the image: boolean getPixelint row, int col and boolean setPixelint row, int col, boolean value; For the getPixel you can use the return value for both the actual data and also the error condition so that we don't "create a scene" if there is an error; we just return false.
Optional A private utility method is highly recommended, but not required: checkSizeString data It does the job of checking the incoming data for every conceivable size or null error. Smaller is okay. Bigger or null is not.
Optional A displayToConsole method that is useful for debugging this class, but not very useful for the assignment at large.
A clone method that overrides the method of that name in Cloneable inter
Step by Step Solution
There are 3 Steps involved in it
Step: 1
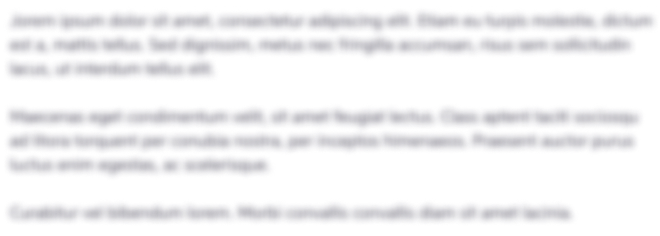
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started