Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Demonstrate the running of the following python code: import cv 2 import numpy as np from scipy.spatial.distance import cdist from skimage.transform import ProjectiveTransform, warp import
Demonstrate the running of the following python code:
import cv
import numpy as np
from scipy.spatial.distance import cdist
from skimage.transform import ProjectiveTransform, warp
import kornia.feature
import kornia.geometry.transform
image cvimreadpathtoyourfirstimage.jpg
image cvimreadpathtoyoursecondimage.jpg
gray cvcvtColorimage cvCOLORBGRGRAY
gray cvcvtColorimage cvCOLORBGRGRAY
sift cvSIFTcreate
keypoints descriptors sift.detectAndComputegray None
keypoints descriptors sift.detectAndComputegray None
distances cdistdescriptors descriptors 'sqeuclidean'
threshold
matches
# Iterate through distance matrix to select matches below the threshold
for i row in enumeratedistances:
for j distance in enumeraterow:
if distance threshold:
matches.appendi j
import numpy as np
import cv
def estimateaffinetransformmatchedpoints matchedpoints:
Estimate an affine transformation using a set of matched points.
# Using cvgetAffineTransform requires exactly points.
# The points need to be reshaped as
if matchedpointsshape or matchedpointsshape:
raise ValueErrorExactly points are required"
transform cvgetAffineTransformnpfloatmatchedpoints npfloatmatchedpoints
return transform
def ransackeypoints keypoints matches, numiterations distancethreshold:
Perform RANSAC to find the best affine transformation.
:param keypoints: Keypoints from the first image.
:param keypoints: Keypoints from the second image.
:param matches: Matched pairs of keypoints indices.
:param numiterations: Number of RANSAC iterations.
:param distancethreshold: Threshold to consider a point as an inlier.
:return: The best affine transformation and the mask of inliers.
maxinliers
besttransform None
for in rangenumiterations:
# Randomly select matches
sampledmatches nprandom.choicematches replaceFalse
# Extract the matched keypoints' coordinates
pts npfloatkeypointsmpt for m in sampledmatches
pts npfloatkeypointsmpt for m in sampledmatches
# Estimate affine transform from pairs of matched points
M estimateaffinetransformpts pts
# Transform all keypoints from the first image to the second image's coordinate system
ptstransformed cvtransformnparraykeypointsmpt for m in matchesreshape M
# Calculate distances between actual and transformed points
distances npsqrtnpsumptstransformed nparraykeypointsmpt for m in matchesreshape axisflatten
# Determine inliers where distance is below threshold
inliers distances distancethreshold
# Update best transformation if current one has more inliers
if suminliers lenmaxinliers:
maxinliers inliers
besttransform M
return besttransform, maxinliers
mosaic:height :width image
mosaic:height :width npmaximummosaic:height :width warpedimage
# Display the color mosaic result
cvimshowMosaic mosaic
Make whatever changes needed preferably minor to run it and show said changes in the code.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
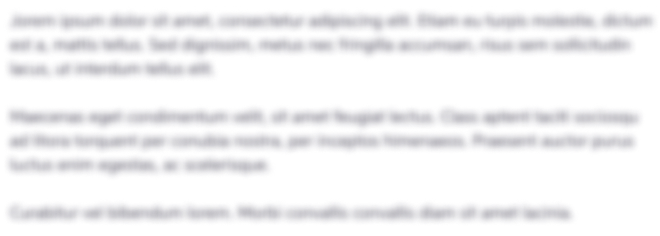
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started