Question
/* Description: * 1. Complete the Box file * a. Complete the constructors * b. Write down the set and get methods for variables *
/* Description:
* 1. Complete the Box file
* a. Complete the constructors
* b. Write down the set and get methods for variables
* 2. Read the boxfile.txt using scanner
* -. First line of the file contains how many boxes are there
* -. Following N rows contains length, width, height of each boxes
* 3. Create Box class Array
* 4. Store all the rows in the Box class array using get set method or Constructor
*
* 5. Find the box with maximum length
* 6. Find the box with minimum length
* 7. Compute volume of each boxes and print that
* 8. Find the box with max volume
* 9. Find the box with min volume
*/
BoxFile.txt
4 2 3 4 1 10 2.3 1.5 2 3 3 4 7
Runner.java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class Runner {
public static void main(String args[]) throws FileNotFoundException {
File file = new File("/Users/afarhan/eclipse-workspace/CS2401_LAB3/src/boxfile.txt");
//Creating Scanner instnace to read File
Scanner scnr = new Scanner(file);
// First line of text file that give us the number of boxes
String n_str = scnr.nextLine();
int n = Integer.parseInt(n_str);
// Box class Array
Box boxes[] = new Box[n];
// Scanning each row of the text file and making boxes with the len,width,height values
int i = 0;
while(i String line = scnr.nextLine(); Box box = new Box(); String items[] = line.split(" "); // This line will not acceptable for your lab // You are supposed to write a set method to set value of each variable of the box box.length=Double.parseDouble(items[0]); box.width=Double.parseDouble(items[1]); box.height=Double.parseDouble(items[2]); boxes[i]=box; i++; } // You need to use get method to access the variables for(int j=0; j System.out.println("Box number "+(j+1)+ " length: "+boxes[j].length+ " width "+boxes[j].width+ " height "+boxes[j].height+ " volume "+boxes[j].getVolume()); } } public static Box getMaxLength(Box[] boxes) { int res=0; return boxes[res]; } public static Box getMinLength(Box[] boxes) { int res=0; return boxes[res]; } public static Box getMaxVolume(Box[] boxes) { int res=0; return boxes[res]; } public static Box getMinVolume(Box[] boxes) { int res=0; return boxes[res]; } } Box.java public class Box { // Convert the public variables to private public double length; public double width; public double height; //Complete the bellow constructors public Box() { } public Box(double length, double width, double height) { } // Write down the set and get methods for all the variables public void setLength(double length) { } public void setWidth(double width) { } public void setHeight(double width) { } public double getLength() { return 0; } public double getWidth() { return 0; } public double getHeight() { return 0; } // Complete the get volume method public double getVolume(){ return length*width*height; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
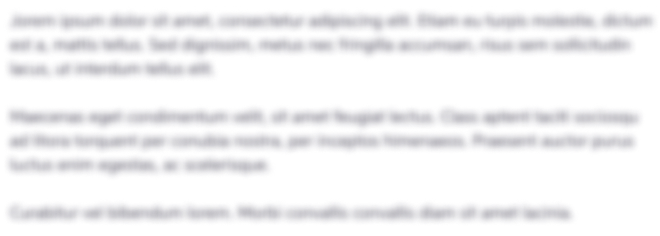
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started