Question
Design class Student with lastName, gpa, and age of data type String, double, and int respectively. The constructor initializes instance variables with data passed via
Design class Student with lastName, gpa, and age of data type String, double, and int respectively. The constructor initializes instance variables with data passed via parameter list. Design the following methods:
int compareTo(Student s) // returns -1 if this student has lower gpa than s, returns 0 if this
// student has the same gpa as s, otherwise, returns 1.
String toString() // returns string representation of student object ( data separated by
// tab and all data for each individual student in one line)
double getGPA() // returns gpa
Design private class Node with two public instance variables: data of Student type, and next of Node type. Class Node has constructor with parameter s of Student type. Constructor creates a Node with variables data and next being assigned s and null, respectively. Class Node is inner class of class LLStudent.
Class LLStudent has one private instance variable list of Node type. It has constructor that creates an empty list. It assigns value null to variable list. Design the following methods which should work properly for any linked list of Student objects:
void addFront(Student s) // inserts a new node with s in it at the front of the linked list.
void addTail(Student s) // inserts a new node with s in it at the tail of the linked list.
Student worstSudent() // returns a Student object with lowest gpa in entire linked list.
void printLinkedList() // traverses linked list and prints each student as separate line.
Node getList() // returns reference to the beginning of the linked list
EXTRA CREDIT:
Design the recursive methods:
Student bestStudentRec(Node first) // returns a student with highest gpa among all students in the linked list. Reference first refers to the first node in the linked list. Must use compareTo in the code.
boolean isSortedRec(Node first) // returns true if students are sorted in the linked list by their gpa.
// Must use compareTo in the code.
Class TestList has main method only. In the main method instantiate variables s1, s2, s3, s4 and s5 as the following five student objects:
Adams 3.6 26
Jones 4.0 29
Marcus 4.0 53
Smith 2.2 20
Zee 3.6 36
Create the two linked lists: list1, list2. Add the above five students in the order provided above to each linked list. When adding to list1 use only method addFront for each of the five students. When adding to list2 use only method addTail for each of the five students.
For each linked list perform the following five steps
create an empty linked list,
add five students and
print list by using printLinkedList method.
invoke method worstStudent and display the worst student.
Invoke bestStudentRec for both lists if you do EXTRA CREDIT.
Invoke isSortedRec for both lists if you do EXTRA CREDIT.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
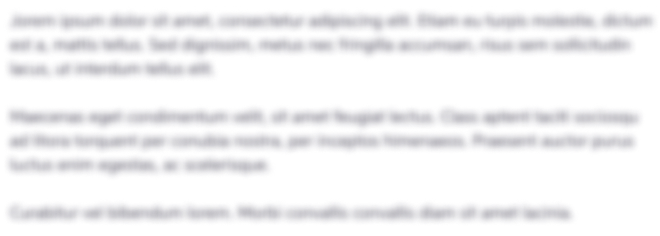
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started