Question
Develop a high-quality, menu-driven object-oriented C++ program that creates a small database, using a binary search tree structure to store and process the data. The
Develop a high-quality, menu-driven object-oriented C++ program that creates a small database, using a binary search tree structure to store and process the data. The database will contain the top 100 highest grossing films of 2015.
The C++ object-oriented program must use the BinarySearchTree class from the textbook (along with the BinaryNode, BinaryNodeTree, BinaryTreeInterface, NotFoundException,and PrecondViolatedExcep classes it requires) to fulfill the requirements of this project. When designing and implementing the program, apply good software engineering principles. Create a makefile for the program that allows compilation and execution of the program from within jGRASP using the Windows operating system. Start the analysis and design process by drawing a complete UML class diagram for the program that includes all the classes that are contained in the program, including the classes provided by the textbook and the classes that you create. The UML class diagram will assist you in understanding the components of the project.
Your program must include:
a Film class that stores all the data for a Film and provides appropriate methods to support good software engineering principles,
a FilmDatabase class that stores the binary search tree and provides appropriate methods to support good software engineering principles,
a Menu class that contains, as a minimum, a method for each menu used in the program that displays the menu and responds to all menu choices made by the user, calling appropriate methods in the FilmDatabase class as necessary, and
a file named Project1.cpp that contains the main() method.
You may also include other classes, as needed. Note that the binary search tree must store Film objects, and the >, <, and == operators must be defined for that class because the BinarySearchTree class uses those overloaded operators.
The downloaded files include the Student class (Student.h and Student.cpp), the StudentDatabase class (StudentDatabase.h and StudentDatabase.cpp), BSTTest.cpp, and makefile for you to use as an example to help you begin your program. The program compiles, links, and executes if you Make it using jGRASP/Windows.
Data Details:
The database contains data pertaining to the 100 highest grossing films of 2015. A comma delimited file named Films2015.csv contains the initial data. Each record is stored on one line of the file in the following format:
Data Data type
Rank int Film Title (key) string Studio string Total Gross double Total Theaters int Opening Gross double Opening Theaters int Opening Date string
Each of the data fields is separated in the file using the comma (,) character as a delimiter. There is no comma (,) character after the last field on the line. The data is considered clean; There are no errors in the input file.
When storing the data in the binary search tree, use the data types shown above. The Film Title will serve as the key field.
Menu Details:
Your program must begin by inputting the text data from the Films2015.csv text file and building a binary search tree for the Films, in order by the key (the film title). Then the program must display the following Main Menu:
MAIN MENU D - Describe the Program R - Reports S - Search the Database X - Exit the Program
Enter Selection ->
All menu choices are selected by typing the number of the choice.
D - Describe the Program
If the user chooses Describe the Program, the program provides a detailed description for the user, explaining what the program is doing and how it works. Note that this method does NOT substitute for javadoc-style comments. The audience for this method consists of non-technical users that have no information at all about the assignment. After providing the description, display the MAIN MENU again. Do NOT use recursion to do this; use a loop.
R - Reports
If the user chooses Reports from the MAIN MENU, the program displays the following menu:
REPORTS MENU T - Order by Film Title report R - Order by Rank report X - Return to main menu
Enter Selection ->
If the user chooses Order by Film Title report from the REPORTS MENU, the program should do the following:
Display a report in order by Film Title that contains all the data for each record stored in the binary search tree.
Identify the report and label all the data displayed appropriately.
If the user chooses Order by Rank report from the REPORTS MENU, the program should do the following:
Display a report sorted in increasing order by earnings rank that contains all the data for each record stored in the binary search tree. Note that this does not involve a simple tree traversal. You will need to write code that specifically performs this function. This is not a simple method and it will require some thought on your part. You may assume that the rank is never less than 1 and never greater than 100. Do NOT copy the data into another binary search tree, into an array, into a linked list, or into any other type of data structure. Retrieve the data directly from the binary search tree which is stored in order by the Film Title.
Identify the report and label all the data displayed appropriately.
If the user chooses Return to main menu from the REPORTS MENU, then display the MAIN MENU again. Do NOT use recursion to do this; use a loop.
S - Search the Database
If the user chooses Search the database, the program should display another menu, as follows:
Search MENU T - Search by Title K - Search by Keyword(s) S - Search by Studio M - Search by month of release X - Return to main menu
Enter Selection ->
If the user chooses Search by Title, the program should do the following:
Request a film title from the user.
Search the database for the title given. This will be an exact match of the film title and at most, only 1 record will match. A case insensitive search should be performed.
If the film title is found, display the record stored in the binary search tree. Otherwise report that the requested film title was not found.
Identify the output and label all the data displayed appropriately.
If the user chooses Search by Keyword(s), the program should do the following:
Request title keywords from the user.
Search the database for all titles that contain the keyword(s). The keywords given represent all or a portion of the title. When multiple keywords are given, only titles that contain the keywords in the order given will be selected. For example, if the user enters Star Wars, Star Wars: The Force Awakens will be selected as will The Making of Star Wars. However, One Star, Many Wars, Once Upon a Star, Wars and Wars with Stars will not be selected.
Multiple search keywords may be entered by separating the keywords with a comma. All titles containing one or more of the keywords will be selected. For example, if the user enters 'Cinderella,SpongeBob,Dinosaur', "Cinderella", "The Good Dinosaur", "In Search of Dinosaurs", "The SpongeBob Movie: Sponge Out of Water" as well as "Cinderella Loves Dinosaurs and SpongeBob!" will be selected.
Display all records stored in the binary search tree where the title contains the keyword(s) given by the user. If there are no records containing the keyword(s), report that the requested keyword(s) were not found.
Identify the output and label all the data displayed appropriately.
If the user chooses Search by Studio, the program should do the following:
Request the name of a Studio from the user.
Search the database for an exact match of the studio name.
Display all records stored in the binary search tree where the studio name matches the name given by the user. If there are no records with the studio name given, report that the requested studio was not found.
Identify the output and label all the data displayed appropriately.
If the user chooses Search by month of release, the program should do the following:
Request a month value between 1 and 12 from the user.
Search the database for all records where the release date contains the month requested. Note: the date is stored as a string.
Display all records stored in the binary search tree that match the month given by the user. If there are no records with the requested release month, report that the requested month was not found.
Identify the output and label all the data displayed appropriately.
If the user chooses Return to main menu, then display the MAIN MENU again. Do NOT use recursion to do this; use a loop.
X Exit the program
If the user chooses Exit the program from the MAIN MENU, the program ends.
Since the program is controlled and manipulated by a human user, be sure to perform adequate error checking on every aspect of the program.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
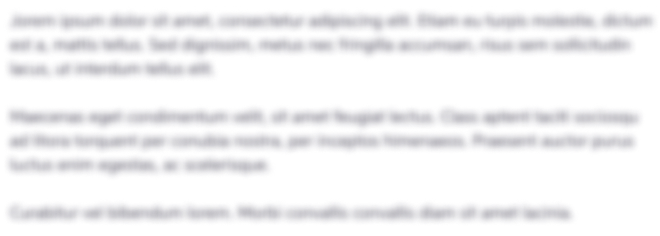
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started