Question
Develop a high-quality, object-oriented C++ program that performs a simulation using a heap implementation of a priority queue. A simulation creates a model of a
Develop a high-quality, object-oriented C++ program that performs a simulation using a heap implementation of a priority queue.
A simulation creates a model of a real-world situation, allowing us to introduce a variety of conditions and observe their effects. For instance, a flight simulator challenges a pilot to respond to varying conditions and measures how well the pilot responds. Simulation is frequently used to measure current business practices, such as the number of checkout lines in a grocery store or the number of tellers in a bank, so that management can determine the fewest number of employees required to meet customer needs.
Airlines have been experimenting with different boarding procedures to shorten the entire boarding time, keep the flights on-time, reduce aisle congestion, and make the experience more pleasant for passengers and crew. A late-departing flight can cause a domino effect:
the departure gate is tied up and cannot be used by other landing or departing flights,
passengers on board the late flight may miss connecting flights and require rebooking and possibly overnight arrangements (meals and lodging), etc., and
passengers complain about being late, and/or about having to rearrange their plans.
Thus, late flights have a huge operational impact.
For this project, we will simulate boarding procedures for Airworthy Airlines. The Airline's current procedure is as follows:
pre-board in the following order:
families with young children or people who need help (e.g., wheelchair)
first class and/or business class passengers
elite passengers (frequent fliers) and those passengers seated in exit rows
conduct general boarding in reverse, from the back of the plane to the front in the following order:
rows 23-26
rows 17-22
rows 11-16
rows 5-10
Airworthy is considering revising their boarding procedure such that general boarding is done randomly, meaning the first passenger in line for general boarding is the first passenger to board (i.e., general boarding passengers all have the same priority). Airworthy suspects this random general boarding method will improve the flow of passengers, getting them on board and seated more quickly. It is also less labor-intensive for Airworthy's customer service agents because it significantly reduces the number of boarding announcements required and eliminates confrontations with customers trying to board "out of turn." Note that the revision is to general boarding only. The pre-boarding procedures will not be changed.
Develop an object-oriented C++ program that simulates these two boarding procedures using a heap implementation of a priority queue. Assume that all passengers are already checked in and at the gate area when boarding begins for both scenarios. The simulation should model an Airbus A320-200 plane, which is configured as follows:
Rows 1 through 4 are first class seats (4 seats in each row)
Rows 5 through 26 are coach class seats (6 seats in each row)
Rows 10 and 11 are exit rows
Your program must consist of the Heap_PriorityQueue class from the textbook (which inherits from PriorityQueueInterface and uses the ArrayMaxHeap and HeapInterface classes, which are also provided), the PrecondViolatedExcep class from the textbook, the Passenger class and Airworthy class that you create, and a separate file named SimulationProject.cpp that contains the main() method. When designing and implementing the program, apply good software engineering principles. Create a makefile for the program. Be sure to follow the style guide and be sure to use Javadoc style comments appropriately.
Start the analysis and design process by drawing a complete UML class diagram for the program that includes the application and all the classes that are contained in the program, including the classes provided by the textbook and the classes that you create. After you have completed the program, update the UML class diagram to reflect the completed implementation.
The files for the Heap_PriorityQueue, the PriorityQueueInterface, the ArrayMaxHeap, the HeapInterface, and the PrecondViolatedExcep classes (Heap_PriorityQueue.h, Heap_PriorityQueue.cpp, PriorityQueueInterface.h, ArrayMaxHeap.h, ArrayMaxHeap.cpp, HeapInterface.h, PrecondViolatedExcep.h, and PrecondViolatedExcep.cpp) are provided in a zipped folder, along with input files for the program. Note that the priority queue must store Passenger objects, and the > and < operators must be defined for that class because the ArrayMaxHeap class uses those overloaded operators.
Develop a Passenger class that stores the following data for an Airworthy Airlines passenger:
key - the priority value for the PriorityQueue
passenger's last name
passenger type, a character, where 'H' is a child or passenger who needs help in boarding, 'E' is an elite passenger (frequent flyer), and 'G' is a general boarding passenger
row where passenger is seated; must be a number between 1 and 26, where rows 1-4 are first class and rows 10 and 11 are exit rows
Include a constructor and accessors and mutators for each attribute. In addition, you MUST include methods that overload the < and > operators. You may include other methods, if needed.
Develop an Airworthy class that contains the following data members:
exactly one priority queue where the priority is set using Airworthy's previous boarding procedure
exactly one priority queue where the priority is set using Airworthy's new random boarding procedure
the amount of time, in seconds, required to board a plane using Airworthy's previous boarding procedure
the amount of time, in seconds, required to board a plane using Airworthy's new random boarding procedure
You may use additional attributes, as needed. The Airworthy class must include methods to support the following functions of the class:
a constructor
read the data from the input file
load the two priority queues
run the simulation
Be sure to incorporate adequate error checking for all files and for the priority queue, providing error messages as needed. When setting the priority (the key) for a passenger, keep the pre-boarding procedure described above in mind.
When running the simulation, assume that:
A passenger who is not blocked by another passenger requires one second to board.
A passenger is blocked when the previous passenger is sitting in the same row or a row closer to the front of the plane. A blocked passenger requires 25 seconds to board.
For example,
Passenger 1 seated in row 5 - not blocked (no one in front) - add 1 Passenger 2 seated in row 3 - not blocked (previous passenger's row is 5) - add 1 Passenger 3 seated in row 10 - blocked (previous passenger's row is 3) - add 25 Passenger 4 seated in row 15 - blocked (previous passenger's row is 10) - add 25 Passenger 5 seated in row 9 - not blocked (previous passenger's row is 15) - add 1 Passenger 6 seated in row 7 - not blocked (previous passenger's row is 9) - add 1 Passenger 7 seated in row 9 - blocked (previous passenger's row is 7) - add 25
The SimulationProject.cpp file contains a main() method and a method named description() that provides a detailed description for the user, explaining what the program is doing, how it works, and the location of all output files. Note that the description() method does NOT substitute for Javadoc comments. The audience for the description() method consists of non-technical users who have no information at all about the program or the assignment. The main() method must support the following:
call the description() method
call methods of the Airworthy class to load the priority queues and run the simulation using both the previous boarding procedure and the new random boarding procedure for each of 3 different input files. Each input file contains, on each line, the last name of the passenger, the type of passenger, and the row number in which the passenger is seated. The input files are:
airworthy100.txt which loads the plane at 100% of capacity
airworthy85.txt, which loads the plane at 85% of capacity
airworthy70.txt, which loads the plane at 70% of capacity
call methods of the Airworthy class to create 3 different output files named results100.txt, results85.txt, and results70.txt. Each output file should contain the data related to its similarly named input file. Be sure to title each part of the output and label all data so it is easy to identify. Each file should contain:
a list of each passenger read from the input file, showing the passenger's name, type, and row
a list of each passenger in order as they are removed from the priority queue when running the simulation using the current boarding procedure, showing the passenger's name, type, row, and priority value (the key)
the total number of minutes (shown with 2 decimal places) required to board the plane using the current boarding procedure,
a list of each passenger in order as they are removed from the priority queue when running the simulation for using the new random boarding procedure, showing the passenger's name, type, row, and priority value (the key), and
the total number of minutes (shown with 2 decimal places) required to board the plane using the new random boarding procedure.
Be sure to follow the Project Guidelines & Evaluation Criteria, since the project will be evaluated using this criteria. In addition, be sure to use javadoc-style comments appropriately. When creating javadoc-style comments, keep in mind that the comment will eventually become part of an html file that will be used by other programmers on your programming team, and by maintenance programmers. Remember also that maintenance programmers have not seen the assignment (the specification), so the information you are providing here must provide all of the detailed information another programmer will need to completely understand the program and to maintain the code. This program is worth 10% of your overall course grade, and it will require a major time investment.
Output Examples (Assume 30 passengers):
(When the program reads an external file and runs, it should generate the results as the following example, and the results are saved into another output file.)
Original List in the file: Vang G 23 Velazquez G 5 Langley G 17 Buckner G 14 Barlow G 18 Guthrie G 11 Hyde G 21 Delacruz H 22 Foreman G 7 Hewitt G 12 Downs G 15 Franks G 7 Hendrix G 8 Finch E 10 Mcleod G 10 Workman G 21 Byers G 15 Mcfadden E 13 Goff G 14 Madden G 5 Burris G 2 Talley G 24 Tyson E 20 Lancaster G 18 Burks E 11 Hahn G 8 Clements E 24 Holden G 23 Witt G 6 Snider E 22
Boarding in Previous Procedure ... Last Name Type Row Key Delacruz H 22 30 Burris G 2 29 Guthrie G 11 28 Finch E 10 27 Mcleod G 10 26 Mcfadden E 13 25 Tyson E 20 24 Burks E 11 23 Clements E 24 22 Snider E 22 21 Vang G 23 20 Talley G 24 19 Holden G 23 18 Langley G 17 17 Barlow G 18 16 Hyde G 21 15 Workman G 21 14 Lancaster G 18 13 Buckner G 14 12 Hewitt G 12 11 Downs G 15 10 Byers G 15 9 Goff G 14 8 Velazquez G 5 7 Foreman G 7 6 Franks G 7 5 Hendrix G 8 4 Madden G 5 3 Hahn G 8 2 Witt G 6 1 Total boarding time: 6 minutes and 54 seconds. Boarding in Random Procedure ... Last Name Type Row Key Delacruz H 22 30 Burris G 2 29 Guthrie G 11 28 Finch E 10 27 Mcleod G 10 26 Mcfadden E 13 25 Tyson E 20 24 Burks E 11 23 Clements E 24 22 Snider E 22 21 Vang G 23 20 Velazquez G 5 19 Langley G 17 18 Buckner G 14 17 Barlow G 18 16 Hyde G 21 15 Foreman G 7 14 Hewitt G 12 13 Downs G 15 12 Franks G 7 11 Hendrix G 8 10 Workman G 21 9 Byers G 15 8 Goff G 14 7 Madden G 5 6 Talley G 24 5 Lancaster G 18 4 Hahn G 8 3 Holden G 23 2 Witt G 6 1 Total boarding time: 6 minutes and 30 seconds.
****************************** PriorityQueueInterface.h ******************************
#ifndef _PRIORITY_QUEUE_INTERFACE #define _PRIORITY_QUEUE_INTERFACE
template
{ public: /** Sees whether this priority queue is empty. @return True if the priority queue is empty, or false if not. */ virtual bool isEmpty() const = 0; /** Adds a new entry to this priority queue. @post If the operation was successful, newEntry is in the priority queue. @param newEntry The object to be added as a new entry. @return True if the addition is successful or false if not. */ virtual bool add(const ItemType& newEntry) = 0; /** Removes from this priority queue the entry having the highest priority. @post If the operation was successful, the highest priority entry has been removed. @return True if the removal is successful or false if not. */ virtual bool remove() = 0; /** Returns the highest-priority entry in this priority queue. @pre The priority queue is not empty. @post The highest-priority entry has been returned, and the priority queue is unchanged. @return The highest-priority entry. */ virtual ItemType peek() const = 0; }; // end PriorityQueueInterface #endif
****************************** HeapInterface.h ******************************
#ifndef _HEAP_INTERFACE #define _HEAP_INTERFACE
template
****************************** ArrayMaxHeap.h ******************************
#ifndef _ARRAY_MAX_HEAP #define _ARRAY_MAX_HEAP
#include "HeapInterface.h" #include "PrecondViolatedExcep.h"
template
****************************** ArrayMaxHeap.cpp ******************************
#include
template
template
template
template
template
/* // Another version of rebuild, but does not follow book's pseudocode template
template
//****************************************************************** // // Public methods start here // //******************************************************************
template
template
template
template
template
template
template
template
template
bool inPlace = false; int newDataIndex = itemCount; while ((newDataIndex > 0) && !inPlace) { int parentIndex = getParentIndex(newDataIndex); if (items[newDataIndex] < items[parentIndex]) { inPlace = true; } else { swap(items[newDataIndex], items[parentIndex]); newDataIndex = parentIndex; } // end if } // end while
itemCount++; isSuccessful = true; } // end if return isSuccessful; } // end add
template
****************************** Heap_PriorityQueue.h ******************************
#ifndef _HEAP_PRIORITY_QUEUE #define _HEAP_PRIORITY_QUEUE
#include "ArrayMaxHeap.h" #include "PriorityQueueInterface.h"
template
#include "Heap_PriorityQueue.cpp" #endif
****************************** Heap_PriorityQueue.cpp ******************************
#include "Heap_PriorityQueue.h"
template
template
template
template
template
****************************** PrecondViolatedExcep.h ******************************
#ifndef _PRECOND_VIOLATED_EXCEPT #define _PRECOND_VIOLATED_EXCEPT
#include
using namespace std;
class PrecondViolatedExcep : public logic_error { public: PrecondViolatedExcep(const string& message = ""); }; // end PrecondViolatedExcep #endif
****************************** PrecondViolatedExcep.cpp ******************************
#include "PrecondViolatedExcep.h"
PrecondViolatedExcep::PrecondViolatedExcep(const string& message): logic_error("Precondition Violated Exception: " + message) { } // end constructor
// End of implementation file.
****************************** Passenger.h ******************************
#ifndef PASSENGER_H
#define PASSENGER_H
#include
using namespace std;
class Passenger
{
private:
int key; //The priority value for the PriorityQueue
string lastName; //The passenger's last name
char type; //The type of passenger
int row; //Where the passengers are seated
bool priority;
public:
/**
* Default constructor
*/
Passenger();
/**
* Constructor is passed line from file, parses line and initializes data fields
*/
Passenger(string line, bool genSort);
//Gets the key
int getKey(void);
//Gets the last name
string getLastName(void);
//Gets the type
char getType(void);
//Gets the row
int getRow(void);
//Sets the key
void setKey(int k);
//Sets the last name
void setLastName(string n);
//Sets the type
void setType(char t);
//Sets the row
void setRow(int r);
/**
* Defines the > operator for a Passenger object.
*/
bool operator> (const Passenger &right);
/**
* Defines the < operator for a Pasenger object.
*/
bool operator< (const Passenger &right);
/**
* Defines the == operator for a Passenger object.
*/
bool operator== (const Passenger &right);
}; //end Passenger
#endif
****************************** Passenger.cpp ******************************
#include "Passenger.h"
#include
#include
#include
using namespace std;
/**
* Default Constructor
*/
Passenger::Passenger()
{
}
/**
* Constructor is passed line from file, parses line and initializes data fields
*/
Passenger::Passenger(string line, bool genSort)
{
stringstream ss(line);
//Assign variables from the line
ss >> lastName;
ss >> type;
ss >> row;
key = 1; //Default lowest key value
//Test for priority boarding, assign keys accordingly
if(type == 'H') //Test for child or passengers who need help
{
priority = true;
key = 7;
}
else if(row <= 4) //Test for 1st class
{
priority = true;
key = 6;
}
else if(row == 10 || row == 11 || type == 'E') //Test for frequent flyers
{
priority = true;
key = 5;
}
else
{
priority = false; //Others are general boarding
}
//Parameter flag indicates if non-priority get sorted
if(genSort && !priority) //If sorting by row
{
if(row <= 26 && row >= 23)
{
key = 4; //rows 23-26 board first in general boarding
}
else if(row <= 22 && row >= 17)
{
key = 3; //rows 17-22 board second in general boarding
}
else if(row <= 16 && row >= 11)
{
key = 2; //rows 11-16 board third in general boarding
}
else if(row <= 10 && row >= 5) //rows 10-11 are exit rows and we're already filled
{
key = 1; //rows 5-10 board last in general boarding
}
}
}//end constructor
//Used to access private data fields
int Passenger::getKey(void)
{
return key; //getKey
}
string Passenger::getLastName(void)
{
return lastName; //getLastName
}
char Passenger::getType(void)
{
return type; //getType
}
int Passenger::getRow(void)
{
return row; //getRow
}
//Used to set private data fields
void Passenger::setKey(int k)
{
key = k; //setKey
}
void Passenger::setLastName(string n)
{
lastName = n; //setLastName
}
void Passenger::setType(char t)
{
type = t; //setType
}
void Passenger::setRow(int r)
{
row = r; //setRow
}
bool Passenger::operator> (const Passenger &right)
{
return (key > right.key);
}
bool Passenger::operator< (const Passenger &right)
{
return (key < right.key);
}
bool Passenger::operator== (const Passenger &right)
{
return (key == right.key);
}
****************************** Airworthy.h ******************************
#ifndef AIRWORTHY_H
#define AIRWORTHY_H
#include "Heap_PriorityQueue.h"
#include "Passenger.h"
#include
using namespace std;
class Airworthy
{
private:
//Queue where priority is set using Airworthy's preboarding boarding procedure
Heap_PriorityQueue
//Queue where priority is set using Airworthy's new random boarding procedure
Heap_PriorityQueue
//The amount of time, in seconds, required to board a plane using Airworthy's preboarding procedure
int boardingTime;
//The amount of time, in seconds, required to board a plane using Airworthy's random boarding procedure
int rdmBoardingTime;
//Reads data from input file, loads queues, writes contents of queues to output file
void readInData(ifstream& inFile);
//Empties queue, writes data to output file, and increments boardTime based on the last item's position
void loadPriorityQueue(ifstream& inFile, ostream& outFile);
public:
/**
* Default Constructor
*/
Airworthy();
/**
* Opens output file and writes simulation results of both queues to it
*/
void runSimulation(ostream& outFile);
}; //end Airworthy
#endif
****************************** Airworthy.cpp ******************************
#include
#include
#include
#include
#include "Airworthy.h"
#include "Passenger.h"
#include "Heap_PriorityQueue.h"
using namespace std;
const int NOT_BLOCKED = 1, BLOCKED = 25;
Airworthy::Airworthy()
{
boardingTime = 0;
rdmBoardingTime = 0;
}
void Airworthy::readInData(ifstream& inFile)
{
string tempLastName;
string tempRow;
string strType;
char tempType;
int tempIntRow;
//Reads in the last name of the passenger
getline(inFile, tempLastName, ' ');
myPassenger.setLastName(tempLastName);
//Reads in the passenger type and converts it to a char
getline(inFile, strType, ' ');
tempType = strType[0];
myPassenger.setPassengerType(tempType);
//Reads in the row and converts it to an int
getline(inFile, tempRow);
tempIntRow = atoi (tempRow.c_str());
myPassenger.setRow(tempIntRow);
}//end readInData
void Airworthy::loadPriorityQueue(ifstream& inFile, ostream& outFile)
{
readInData(inFile);
while(inFile)
{
//Previous priority queue loading
preboardConditions();
previousBoarding();
outFile << "PREVIOUS CONDITIONS PRIORITY QUEUE" << endl;
outFile << "Priority Queue:" << endl
<< "Name: " << myPassenger.getLastName() << endl
<< "Type: " << myPassenger.getPassengerType() << endl
<< "Row: " << myPassenger.getRow() << endl << endl;
ppq.add(myPassenger);
//Random priority queue loading
preboardConditions();
randomBoarding();
outFile << "RANDOM CONDITIONS PRIORITY QUEUE" << endl;
outFile << "Priority Queue:" << myPassenger.getKey() << endl
<< "Name: " << myPassenger.getLastName() << endl
<< "Type: " << myPassenger.getPassengerType() << endl
<< "Row: " << myPassenger.getRow() << endl << endl;
rpq.add(myPassenger);
readInData(inFile);
}
}
void Airworthy::runSimulation(ostream& outFile)
{
int passengerOne = 0;
int rdmPassengerOne = 0;
//Previous boarding simulation
//Special consideration needed for first passenger on plane
//First passenger will never be blocked so must be handled
//outside of while loop
outFile << "PREVIOUS CONDITIONS BOARDING" << endl;
ppq.remove();
passengerOne = myPassenger.getRow();
boardingTime += NOT_BLOCKED;
outFile << "Boarding Plane:" << endl
<< "Key: " << myPassenger.getKey() << endl
<< "Name: " << myPassenger.getLastName() << endl
<< "Type: " << myPassenger.getPassengerType() << endl
<< "Row: " << myPassenger.getRow() << endl << endl;
while(!ppq.isEmpty())
{
if(passengerOne >= myPassenger.getRow())
{
cout << "value for passengerOne= " << passengerOne << endl;
boardingTime += BLOCKED;
}
else
boardingTime += NOT_BLOCKED;
passengerOne = myPassenger.getRow();
ppq.remove();
outFile << "PREVIOUS CONDITIONS BOARDING" << endl
<< "Key: " << myPassenger.getKey() << endl
<< "Name: " << myPassenger.getLastName() << endl
<< "Type: " << myPassenger.getPassengerType() << endl
<< "Row: " << myPassenger.getRow() << endl << endl;
}//end while
//Random Boarding simulation
//Special consideration needed for first passenger on plane
//First passenger will never be blocked so must be handled
//outside of while loop
outFile << "RANDOM CONDITIONS BOARDING" << endl;
rpq.remove();
rdmPassengerOne = myPassenger.getRow();
rdmBoardingTime += NOT_BLOCKED;
outFile << "Boarding Plane:" << endl
<< "Key: " << myPassenger.getKey() << endl
<< "Name: " << myPassenger.getLastName() << endl
<< "Type: " << myPassenger.getPassengerType() << endl
<< "Row: " << myPassenger.getRow() << endl << endl;
while(!rpq.isEmpty())
{
if(rdmPassengerOne >= myPassenger.getRow())
rdmBoardingTime += BLOCKED;
else
rdmBoardingTime += NOT_BLOCKED;
rdmPassengerOne = myPassenger.getRow();
rpq.remove();
outFile << "RANDOM CONDITIONS BOARDING" << endl;
outFile << "Boarding Plane:" << endl
<< "Key: " << myPassenger.getKey() << endl
<< "Name: " << myPassenger.getLastName() << endl
<< "Type: " << myPassenger.getPassengerType() << endl
<< "Row: " << myPassenger.getRow() << endl << endl;
}//end while
//Calculations for the final boarding times in minutes
double finalBoardingTime = boardingTime/60;
outFile << fixed;
outFile << setprecision(2) << "Previous Boarding Time = " << finalBoardingTime << " minutes" < double finalRdmBoardingTime = rdmBoardingTime/60; outFile << fixed; outFile << setprecision(2) << "Random Boarding Time = " << finalRdmBoardingTime << " minutes" < } void Airworthy::preboardConditions() { //Comparisons for special boarding conditions if (myPassenger.getPassengerType() == 'H') { myPassenger.setKey(7); } else if (myPassenger.getRow() >= 1 && myPassenger.getRow() <=4) { myPassenger.setKey(6); } else if (myPassenger.getPassengerType() == 'E' || myPassenger.getRow() >= 10 && myPassenger.getRow() <=11) { myPassenger.setKey(5); } } void Airworthy::previousBoarding() { //Comparisons for previous general boarding conditions if (myPassenger.getPassengerType() == 'G' && myPassenger.getRow() >= 23 && myPassenger.getRow() <= 26) { myPassenger.setKey(4); } else if (myPassenger.getPassengerType() == 'G' && myPassenger.getRow() >= 17 && myPassenger.getRow() <= 22) { myPassenger.setKey(3); } else if (myPassenger.getPassengerType() == 'G' && myPassenger.getRow() >= 12 && myPassenger.getRow() <= 16) { myPassenger.setKey(2); } else if (myPassenger.getPassengerType() == 'G' && myPassenger.getRow() >= 5 && myPassenger.getRow() <=9) { myPassenger.setKey(1); } } void Airworthy::randomBoarding() { //Comparison for the random general boarding conditions if (myPassenger.getPassengerType() == 'G' && myPassenger.getRow() >= 5 && myPassenger.getRow() <=9) myPassenger.setKey(4); else if(myPassenger.getPassengerType() == 'G' && myPassenger.getRow() >= 12 && myPassenger.getRow() <=26) myPassenger.setKey(4); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
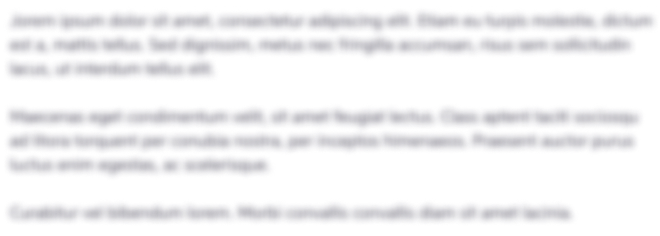
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started