Question
Develop a well ADT class which utilizes operator overloading Use dynamically allocated arrays Problem The Apache web server produces information detailing web page accesses, this
- Develop a well ADT class which utilizes operator overloading
- Use dynamically allocated arrays
Problem
- The Apache web server produces information detailing web page accesses, this information is stored in a log file in the Apache Common log file format. The assignment is to develop a program to process this log file and keep track of the different types of log entries.
Requirements
-
Program must compile and run correctly in Visual Studio
-
You CANNOT use the C++ standard string or any other libraries for this assignment
-
using namespace std; is strictly forbbiden. As are any global using statements
- Iteration 1 - 40 points - Rubric
- Implementation:
-
Re-implement your String class to use a dynamically allocated array for storage. It will be a NULL terminating character array.
-
Use the provided header file (string.hpp).
-
This dynamic version of the String will only allocate exactly the amount of memory necessary to store the characters. That is, the length will be the same as the capacity. However, the size of the dynamic array needs to have an extra char for the NULL terminator.
-
You will need to re-write the constructors to allocate the correct amount of memory.
-
The default constructor allocates an array of size 1 for the empty string. The other constructors will allocate memory as needed. For example for String str(abc); str.capacity() == 3, str.length() == 3, and str.array_size == 4.
-
Implement a destructor, copy constructor, constant time swap, and assignment operator for your ADT.
-
You will need to re-implement capacity(). length() remains the same.
-
You will also have to update concat (+ and +=) to return the properly sized string result.
-
You will have to reimplement operator>> to create a proper sized string result.
- Implement the private constructors String(int) and String(int, const char *) and a private method reset_capacity.
- String(int) constructs the object as the empty string with int capacity.
- String(int, const char *) works the same as String(const char *) but allocates int capacity.
- Implement a private method reset_capacity to change the capacity of your string while keeping the contents intact. That is, create a new array and copy contents over to the new array, making sure to clean up memory (this may grow or shrink the array).
-
The private constructors and method may be useful for re-implementing + and +=.
-
Note: part of the reason these are private is they violate the length == capacity invariant. This is temporary as where they are used will reestablish the invariant.
-
- Testing:
- A testing oracle of several test files is provided.
- Some are commented out in the CMakeLists.txt file so the code compiles before edits. Uncomment them once implemented.
- Implementation:
- Iteration 2 (30 pts) - Rubric
- Implementation:
- Implement method String String::substr(int start_pos, int count) const;. This will return the specified substring starting at start_pos and consisting of count characters (there will be less than count if it extends past the end of the string).
- Implement method int String::find(char ch, int start_pos) const; which gives the first location starting at start_pos of the character ch or -1 if not found.
- Implement method int String::find(const String & s, int start_pos) const; which gives the first location starting at start_pos of the substring s or -1 if not found.
- Implement a method std::vector
String::split(char) const; This method will return a vector of String split up based on a supplied character. For example given s = abc ef gh, the call s.split( ) will return a vector with three strings: abc, ef, and gh.
- Testing:
- A testing oracle is provided for you. The methods are commented out in the CMakeLists.txt file. Uncomment them once implemented.
- Implementation:
String.hpp
Test oracles are supplied for you so you must use this interface and namings. * You must also have all of these methods and functions defined for your string class. * * You can NOT add any attributes/members. If you do the test oracles will not work. * * You will need to uncomment and implment the commented out methods for Iteration 1. * You will have to add the methods to the header for Iteration 2. */
#ifndef INCLUDED_STRING_HPP #define INCLUDED_STRING_HPP
#include
// size of the array. const int STRING_SIZE = 256;
/** * @invariant str[length()] == 0 * && length() == capacity() * && capacity() == array_capacity - 1 */ class String { private: // helper constructors and methods String(int); String(int, const char *); void reset_capacity (int);
// change to: char * str; char * str[STRING_SIZE];
// capacity includes NULL terminator int array_capacity;
public:
// constructor: empty string, String('x'), and String("abcd") String(); String(char); String(const char *);
// copy ctor, destructor, constant time swap, and assignment String(const String &); ~String(); void swap (String &); String & operator= (String);
// subscript: accessor/modifier and accessor char & operator[](int); char operator[](int) const;
// max chars that can be stored (not including null terminator) int capacity() const;
// number of char in string int length() const;
// concatenation // += by value for self assignment String operator+ (const String &) const; String & operator+=(String);
// relational methods bool operator==(const String &) const; bool operator< (const String &) const;
// i/o friend std::ostream & operator<<(std::ostream &, const String &); friend std::istream & operator>>(std::istream &, String &);
};
// free functions for concatenation and relational String operator+ (const char *, const String &); String operator+ (char, const String &); bool operator== (const char *, const String &); bool operator== (char, const String &); bool operator< (const char *, const String &); bool operator< (char, const String &); bool operator<= (const String &, const String &); bool operator!= (const String &, const String &); bool operator>= (const String &, const String &); bool operator> (const String &, const String &);
#endif
String.cpp
String::String() { str[0] = '\0'; }
String::String(char ch) { str[0] = ch; str[1] = '\0'; }
String::String(const char cString[]) { int len = 0; while (cString[len] != '\0') { ++len; } for (int i = 0; i < len; ++i) { str[i] = cString[i]; }
str[len] = '\0'; }
int String::capacity() const { return STRING_SIZE - 1; }
int String::length() const { int len = 0; while(str[len] != '\0') { ++len; } return len; }
bool String::operator==(const String& rhs) const { int index = 0; while (str[index] != '\0' && rhs.str[index] != '\0') { if (str[index] != rhs.str[index]) { return false; } ++index; }
return str[index] == rhs.str[index]; }
bool String::operator< (const String& rhs) const { int index = 0; while (str[index] != '\0' && rhs.str[index] != '\0' && str[index] == rhs.str[index]) { ++index; }
return str[index] < rhs.str[index]; }
String String::operator+ (const String& rhs) const { String result(str); int index = 0; int offset = length(); while (rhs.str[index] != '\0') { result.str[offset] = rhs.str[index]; ++index; ++offset; } result.str[offset] = rhs.str[index]; return result; }
String& String::operator+=(String rhs) { int offset = length();
int index = 0; while(rhs.str[index] != '\0') { str[offset] = rhs.str[index]; ++offset; ++index; }
str[offset] = rhs.str[index]; return *this; }
char& String::operator[](int index) { return str[index]; }
char String::operator[](int index) const { return str[index]; }
std::ostream& operator<<(std::ostream& out, const String& data) { for (int i = 0; i < data.length(); ++i) { out << data[i]; } return out; }
std::istream& operator>>(std::istream& in, String& word) { word = ""; char raw_data[256]; if (in >> raw_data) { word = String(raw_data); } return in; }
String operator+ (const char* lhs, const String& rhs) { return String(lhs) + rhs; }
String operator+ (char lhs, const String& rhs) { return String(lhs) + rhs; }
bool operator== (const char* lhs, const String& rhs) { return String(lhs) == rhs; }
bool operator== (char lhs, const String& rhs) { return String(lhs) == rhs; }
bool operator< (const char* lhs, const String& rhs) { return String(lhs) < rhs; }
bool operator< (char lhs, const String& rhs) { return String(lhs) < rhs; }
bool operator<= (const String& lhs, const String& rhs) { if (lhs == rhs) { return true; }
if (lhs < rhs) { return true; } return false; }
bool operator!= (const String& lhs, const String& rhs) { return (!(lhs == rhs)); }
bool operator>= (const String& lhs, const String& rhs) { return (!(lhs < rhs)); }
bool operator> (const String& lhs, const String& rhs) { return (!(lhs <= rhs)); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
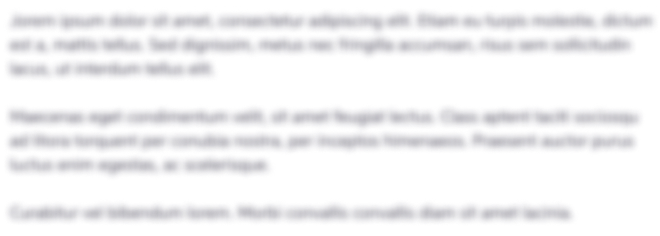
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started