Question
Develop this in C++ Develop a program that implements a student information database. Start by implementing a class and name it Student. The class shall
Develop this in C++
Develop a program that implements a student information database. Start by implementing a class and name it Student. The class shall have a few data members; first_name, last_name (both of type string), studentID (of type integer), and scores[NUM_TESTS], where scores is an integer array and NUM_TESTS is a macro that you should define as 5 for now. A count data member should also exist to hold the number of valid scores (i.e. the ones that have been entered). Add any other data or function members as you see fit. Your constructor should initialize all strings to (i.e. empty) and all scores to zeros. The count should also be initialized to zero since initially the database user hasnt added any valid scores. You may want to add a function member add_score, that adds a test score (and hence it needs to also increment the count)
Create the class Records, which contains an array of type Student (i.e. use class aggregation), and whose size is equal to MAX_NUM_STUDENTS which is a macro that you should define as 30 for now (i.e. 30 students). This class should also contain a data member (count) that keeps track of the number of students whose information is valid. In addition, it should have member functions for adding new students and for adding scores. Note that the function for adding scores should first identify the students object within the student array of 30 objects/students and then invoke its corresponding Student::add_score member function (Hint: you will need to do string comparison). Also it should overload the operator [] and have it return a reference to the Student whose index is passed as the array operators index. Hence the prototype should look like this:
Student& operator[](int idx);
Your main routine should go through a while loop that asks the user to enter a command. For now implement the following commands;
addstudent firstname lastname (creates a new student) addscore firstname lastname score (This should append a new score to an already created student) print (This should print all valid students, each with its valid scores, one student per line) quit (exits the program)
If the user enters any other command, then the loop repeats by asking the user to enter a valid command. Commands should be case-insensitive. It may be a good idea to convert all words/strings to lower case. Heres a quick way to convert a string whose name is mystr to lowercase:
for(int i=0;i The Following is the Sample of what the records.h file should look start to look like #ifndef RECORDS.H #define RECORDS.H #define NUM_SCORES 5 #define NUM_STUDENTS 30 #include class student { public: int count; string firstname; string lastname; float scores[NUM_SCORES]; Student(); ~Student(); bool add_score(float score); }; class records { Student student[NUM_STUDENTS]; int count; public: Records(); ~Records(); bool add_student(string, string); bool add_score(string, string, float); int get_count() { return count; } Student& operator[](int); }; #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
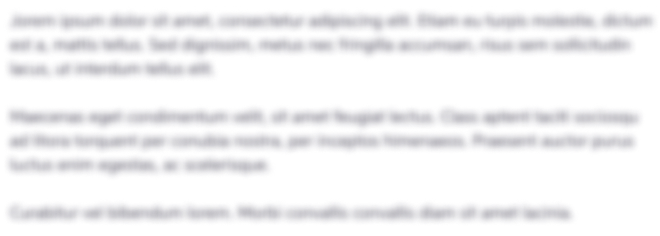
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started