Question
// did the snake eat itself? // end the game if the snake collides with itself public void detectDeath() { Block current = head.next; while
// did the snake eat itself? // end the game if the snake collides with itself public void detectDeath() { Block current = head.next; while (current != null) { if (head.x == current.x && head.y == current.y) { System.exit(0); } current = current.next; } }
// redraw the board with snake and apple updated positions public void paint() { for (int i = 0; i < gridColumns; ++i) { for (int j = 0; j < gridRows; ++j) { if (i % 2 == j % 2) { setBGColor(j, i, bg); } else { setBGColor(j, i, bc); } } }
setBGColor(head.y, head.x, hc);
drawSymbol(apple.y, apple.x, NamedSymbol.apple, ac); drawSymbol(bomb.y, bomb.x, NamedSymbol.bomb, ac);
Block current = head.next; while (current != null) { setBGColor(current.y, current.x, fg); current = current.next; } }
// Set up the first state of the game grid //TODO: Init a queue of blocks that represent the snake. //the head and tail pointers are currently not initialized. //The head variable can represent the first block in the linked list queue and the tail can represent the last block public void initialize() { System.out.println("initialize"); for (int i = 0; i < gridColumns; ++i) { for (int j = 0; j < gridRows; ++j) { if (i % 2 == j % 2) { setBGColor(j, i, bg); } else { setBGColor(j, i, bc); } } }
//TODO: START LINKNED LIST QUEUE HERE BY ASSIGNING HEAD AND TAIL VARIABLES for (int i = 0; i < startLength; ++i) { setBGColor(startY, startX - i, fg); if (i > 0) { //TODO: BASED ON THE SIZE OF THE STARTING SNAKE, ENQUEUE A NEW BLOCK TO THE LINKED LIST } }
frameTime = System.nanoTime(); nextFrameTime = frameTime + FRAMERATE; dir = Direction.EAST; lastDir = dir; apple = new Block(); bomb = new Block(); plantApple(); plantBomb(); }
// Game loop will run many times per second. // handle input, check if apple was detected, update position, redraw, // detect if snake ate itself public void gameLoop() { handleInput(); if (System.nanoTime() > nextFrameTime) { frameTime = System.nanoTime(); nextFrameTime = frameTime + FRAMERATE;
lastDir = dir;
detectApple(); detectBomb();
updatePosition();
paint();
detectDeath();
} }
public static void main(String args[]) { SnakeScaffold game = new SnakeScaffold(22, "test", "137842425086", gridColumns, gridRows); game.setTitle("snake"); game.setDescription("Snake: Eat the food, not yourself!");
game.start(); }
//TODO: Handle enqueue of a new block to the linked list, the tail is the last position in the linked list, //next is the new block to enqueue. //Return the tail of the list. public static Block enqueue(Block tail, Block next) { return tail; }
//TODO: Handle dequeue of a new block to the linked list, the head is the first position in the linked list, //Return the head of the list. public static Block dequeue(Block head) {
return head; } }
enum Direction { NORTH, SOUTH, EAST, WEST }
class Block {
public Block next; public int x; public int y;
public Block() { this(-1, -1, null); }
public Block(int x, int y) { this(x, y, null); }
public Block(int x, int y, Block next) { this.x = x; this.y = y; this.next = next; } } please I just need the java coding for the TODOs, *code the TODOs*, please answer correctly please. thank you.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
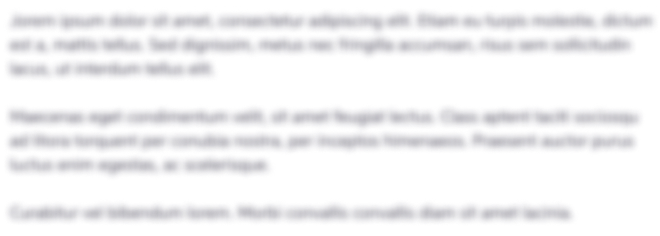
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started