Question
DO IT IN JAVA Counting Game Pieces of the CountingGame class already exist and are in CountingGame.java. Take a look at that code now if
DO IT IN JAVA
Counting Game Pieces of the CountingGame class already exist and are in CountingGame.java. Take a look at that code now if you have not done so already. Also before you start, make sure you are familiar with the methods available to you in the AList class (check ListInterface.html).
Step 1. Compile the classes CountingGame and AList. Run the main method in CountingGame. Checkpoint: If all has gone well, the program will run and accept input. It will then generate a null pointer exception. The goal now is to create the list of players.
Step 2. Create a new Alist and assign it to players.
Step 3. Using a loop, add new objects of type Integer to the players list. Checkpoint: Compile and run the program. Enter 3 for the number of players. The program should print out { } for the players list. The next goal is to do one round of the game. It will be encapsulated in the method doRhyme().
Step 4. Complete the doRhyme() method. Use the following algorithm. For each word in the rhyme Print the word in the rhyme and the player that says it. Print the name of the player to be removed. Remove that player from the list. Return the index of the player that will start the next round.
Step 5. Call doRhyme(players, rhyme, position) in main after the call to getRhyme().
Step 6. Print out the new player list. 170 Lab 12 List Client Checkpoint: Compile and run the program. Enter 6 for the number of players. Enter A B C for the rhyme. It should print out something similar to Player 1: A Player 2: B Player 3: C Removing player 3 The players list is { } Enter 5 for the number of players. Enter A B C D E F for the rhyme. Compare your result with your answers in the pre-lab. Reconcile any differences. The final goal is to do multiple rounds.
Step 7. Wrap the lines of code from the previous two steps in a while loop that continues as long as there is more than one player left. Final checkpoint: Compile and run the program. Enter 6 for the number of players. Enter A B C for the rhyme. The players should be removed in the order 3, 6, 4, 2, 5. The winner should be player 1. Enter 5 for the number of players. Enter A B C D E F for the rhyme. Compare your result with your answers in the pre-lab exercises. Reconcile any differences.
import java.io.*;
import java.util.*;
public class CountingGame
{
public static void main(String args[])
{
ListInterface
ListInterface
int max;
int position = 1; // always start with the first player
System.out.println("Please enter the number of players.");
max = getInt(" It should be an integer value greater than or equal to 2.");
System.out.println("Constructing list of players");
// ADD CODE HERE TO CREATE THE LIST OF PLAYERS
System.out.println("The players list is " + players);
rhyme = getRhyme();
// ADD CODE HERE TO PLAY THE GAME
System.out.println("The winner is " + players.getEntry(1));
}
/**
* Do the rhyme with the players in the list and remove the selected
* player.
*
* @param players A list holding the players.
* @param rhyme A list holding the words of the rhyme.
* @param startAt A position to start the rhyme at.
*
* @return The position of the player eliminated.
*/
public static int doRhyme(ListInterface
{
// COMPLETE THIS METHOD
return -1;
}
/**
* Get an integer value.
*
* @return An integer.
*/
private static int getInt(String rangePrompt)
{
Scanner input;
int result = 10; //Default value is 10
try
{
input = new Scanner(System.in);
System.out.println(rangePrompt);
result = input.nextInt();
}
catch(NumberFormatException e)
{
System.out.println("Could not convert input to an integer");
System.out.println(e.getMessage());
System.out.println("Will use 10 as the default value");
}
catch(Exception e)
{
System.out.println("There was an error with System.in");
System.out.println(e.getMessage());
System.out.println("Will use 10 as the default value");
}
return result;
}
/**
* getRhyme - Get the rhyme.
*
* @return A list of words that is the rhyme.
*/
private static ListInterface
{
Scanner input;
String inString = "";
ListInterface
try
{
input = new Scanner( System.in );
System.out.println("Please enter a rhyme");
inString = input.nextLine().trim();
Scanner rhymeWords = new Scanner(inString);
while(rhymeWords.hasNext())
{
rhyme.add(rhymeWords.next());
}
}
catch(Exception e)
{
System.out.println("There was an error with System.in");
System.out.println(e.getMessage());
System.out.println("Will use a rhyme of size one");
}
// Make sure there is at least one word in the rhyme
if(rhyme.getLength() < 1)
rhyme.add("Default");
return (ListInterface
}
}
-If need anything else just comment also I realize ihavent posted AList and Listerinterface because the questions is to long, but im not sure there any other way to send it to you cause i can't use a 3rd party website. Chegg gave me warning when i used a cite called codeshare to share my code. Not sure if im allowed to use google drive to share my code.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
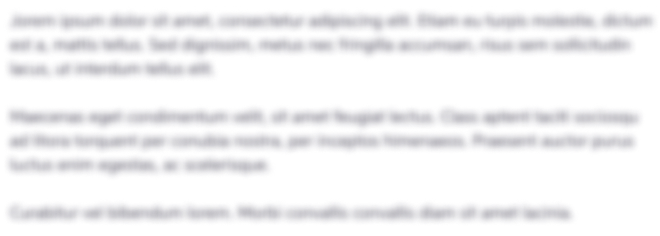
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started