Answered step by step
Verified Expert Solution
Question
1 Approved Answer
//************************************************ // Dog.java // // Represents a dog. // //************************************************ import java.util.Scanner; public class Dog { private static int Dog_Count = 0; // Instance variables
//************************************************ // Dog.java // // Represents a dog. // //************************************************ import java.util.Scanner; public class Dog { private static int Dog_Count = 0; // Instance variables private String name; private String breed; private int age; //----------------------------------------------------- // Constructor - sets up a dog object by initializing // the name, the breed, and the age. //----------------------------------------------------- public Dog(String name, String breed, int age) { this.name = name; this.breed = breed; this.age = age; Dog_Count++; } //----------------------------------------------------- // Constructor - sets up a dog object by initializing // the name, the breed, and the age from a file. //----------------------------------------------------- public Dog(Scanner dogScanner) { this.name = dogScanner.nextLine(); this.breed = dogScanner.nextLine(); this.age = dogScanner.nextInt(); dogScanner.nextLine(); Dog_Count++; } //-------------------------------------------------------------- // Method ageInPersonYears that takes no parameter. The method // should compute and return the age of the dog in person years // (seven times the dog's age). //-------------------------------------------------------------- public int ageInPersonYears() { int personAge = age*7; return personAge; } //------------------------------------------------------ // Returns a string representation of a dog. //------------------------------------------------------ public String toString() { return name + "\t"+ breed + "\t" + age + " age in person years:" + ageInPersonYears(); } public static int Get_Dog_Count() { return Dog_Count; } }
//************************************************** // DogsFromFile.java // A test driver for class Dog. //************************************************** import java.util.Scanner; import java.io.File; import java.io.FileNotFoundException; public class DogsFromFile { //----------------------------------------------- // Creates an array of Dog objects and then outputs // the attributes of all of the dogs in the array. //----------------------------------------------- public static void main (String[] args) { Dog[] dogs = new Dog[100]; int count = 0; String filename = ""; Boolean ok = false; System.out.println("This is program DogsFromFile."); System.out.println(); while (!ok) { // Get file name from the user System.out.print("Please enter file name: "); Scanner keyboardScanner = new Scanner(System.in); filename = keyboardScanner.nextLine(); try { File file = new File(filename); Scanner fileScanner = new Scanner(file); // Get dogs from the file. while (fileScanner.hasNext()) // Test for end of file { Dog dog1 = new Dog(fileScanner); dogs[count++] = dog1; } System.out.println("End of file reached"); System.out.println(); // Output the dogs from the array. for (int i = 0; i
Step by Step Solution
There are 3 Steps involved in it
Step: 1
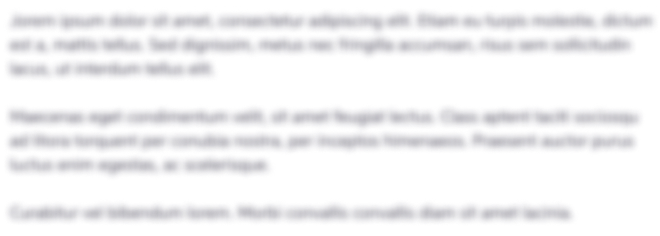
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started