Question
Download the attached file/s, copy and paste the code segment/s into your visual studio or any other C++ IDE and run it. You will have
Download the attached file/s, copy and paste the code segment/s into your visual studio or any other C++ IDE and run it. You will have to implement a small intentional bug in your program and post it for other students to debug.
To be able to receive your full discussion points, you need to submit the following.
Following is your check list and rubric
Attach your .cpp file/s with an implemented bug - 20pnts
Describe what the code does in detail - 20pnts
Debug at least one other program and submit your .cpp file - 40pnts (note what the bug was in comments)
After running the debugged program, attach the screen shot of your output- 20pnts
// This program demonstrates the binarySearch function, which // performs a binary search on an integer array. #includeusing namespace std; // Function prototype int binarySearch(const int [], int, int); const int SIZE = 20; int main() { // Array with employee IDs sorted in ascending order. int idNums[SIZE] = {101, 142, 147, 189, 199, 207, 222, 234, 289, 296, 310, 319, 388, 394, 417, 429, 447, 521, 536, 600}; int results; // To hold the search results int empID; // To hold an employee ID // Get an employee ID to search for. cout << "Enter the employee ID you wish to search for: "; cin >> empID; // Search for the ID. results = binarySearch(idNums, SIZE, empID); // If results contains -1 the ID was not found. if (results == -1) cout << "That number does not exist in the array. "; else { // Otherwise results contains the subscript of // the specified employee ID in the array. cout << "That ID is found at element " << results; cout << " in the array. "; } return 0; } //*************************************************************** // The binarySearch function performs a binary search on an * // integer array. array, which has a maximum of size elements, * // is searched for the number stored in value. If the number is * // found, its array subscript is returned. Otherwise, -1 is * // returned indicating the value was not in the array. * //*************************************************************** int binarySearch(const int array[], int size, int value) { int first = 0, // First array element last = size - 1, // Last array element middle, // Mid point of search position = -1; // Position of search value bool found = false; // Flag while (!found && first <= last) { middle = (first + last) / 2; // Calculate mid point if (array[middle] == value) // If value is found at mid { found = true; position = middle; } else if (array[middle] > value) // If value is in lower half last = middle - 1; else first = middle + 1; // If value is in upper half } return position; }
Above is the file we were given.
Can someone help me create a file that has an intentional bug in it? Then, can you tell me bug it is?
These are all the instructions I was given. This is in C++ if you missed it.
Thank you in advance.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
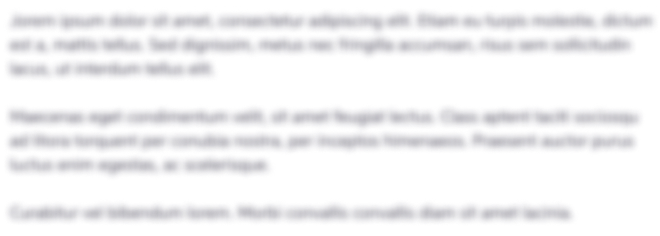
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started