Question
Due date/Time: Monday, Feb. 19, 2018 at 5:30pm What this Lab Is About: Exercise on while, for and do..while loops Familiar with menu-driven program Cotinue
Due date/Time: Monday, Feb. 19, 2018 at 5:30pm
What this Lab Is About:
Exercise on while, for and do..while loops
Familiar with menu-driven program
Cotinue exercise on switch statement
Coding Guidelines for All Labs/Assignments (You will be graded on this)
Give identifiers semantic meaning and make them easy to read (examples numStudents, grossPay, etc).
Keep identifiers to a reasonably short length.
Use upper case for constants. Use title case (first letter is upper case) for classes. Use lower case with uppercase word separators for all other identifiers (variables, methods, objects).
Use tabs or spaces to indent code within blocks (code surrounded by braces). This includes classes, methods, and code associated with ifs, switches and loops. Be consistent with the number of spaces or tabs that you use to indent.
Use white space to make your program more readable.
Use comments properly before or after the ending brace of classes, methods, and blocks to identify to which block it belongs.
1. Lab Description
For this Lab your program must perform three different arithmetic operations based on a users input. To do this, you must show the user the menu, ask the user for their choice, perform the appropriate computation, and then repeat until the user exits. The menu is as follows:
1) Calculate the sum of integers from 1 to m. 2) Calculate the factorial of a given number. 3) Find the first digit of a given number. 4) Quit.
Please choose from above menu:
1.1 Step 1: Getting Started
Similar as what you've done in previous labs, create a C++ source code called Lab6.cpp, use the same setup for setting up your class and main method as you did in previous labs and assignments. Be sure to name your file Lab6.cpp (again, no empty spaces and special characters are allowed, follow the naming conventions).
For documentation purpose, at the beginning of each programming lab/assignment, you must have a comment block with the following information inside:
//----------------------------------------------------------- // FILENAME: Lab6.cpp // AUTHOR: your name // ASU ID: your 10 digits ASU ID // SPECIFICATION: short description of the program //-----------------------------------------------------------
1.2 Step 2: Beginning the loop and declaring variables
The first part of the problem is to show the user the menu and repeat the menu until the user selects the exit option. For this, we need to declare a variable choice to store the user choice.
int choice;
Next, we must select the appropriate loop for the main body of the program. In this case, since we know that the program should execute at least once, a do..while loop is most appropriate. Inside the loop, print the menu using cout statements and then request the menu option from the user. A skeleton do-while loop follows.
do{ //1) Calculate the sum of integers 1 to m. //2) Calculate the factorial of a given number. //3) Find the first digit of a given number. //4) Quit.
//Please choose your choice from following menu
} while(/* termination condition*/)
Remember every loop should have a termination condition. Here the loop should run until user chooses option 4. So the termination condition would be (choice != 4)
1.3 Step 3: Performing arithmetic operations
Based on the choice entered by the user, we need to do some arithmetic operations. Inside the do..while loop, but after the menu, initialize a switch statement to perform the operations. The switchstatement depends on the choice variable, which should now have an integer value. An example switch statement follows.
switch(//----) //switch base on 'choice' { case 1: //code for case #1 break; case 2: //code for case #2 break; case 3: //code for case #3 break; default: break; }
1.4 Step 4: Write codes for Case #1 (Write a counter-controlled while loop)
If the user chooses option one, they wish to compute the sum of integers from one to a target number, for example, m. Thus, we must request this target number from the user and sum up the integers from 1 to that number. First, we declare two new integer variables, one for the target number m and one for the sum. It is important to initialize the value of sum as 0, else the sum variable may start with an incorrect value.
//declare two variables, m and sum, also initialize sum to be 0 //----
Next, show a user prompt message on screen and ask the user to enter a number and then use cin to get the number, store it inside variable m.
To perform the operation, we need an intermediate variable that goes from 1 to m in a loop. For that, declare and initialize a variable. Note: you can view this variable as a counter or an index that typically start at i and proceed in order of i, i+1, i+2, ... . for this case, till m.
//declare a variable i and intialize it to be 1. i.e counter starts from 1 //----
Begin a while loop using i as the counter and until its value is less or equals to m and inside the loop update the value of the sum:
while (//as long as counter i is less or equals to m) { //update sum. sum should be its previous value plus i //---- //increase counter i by 1 //----
}
After above while loop terminate, you will need to print the value of sum so that user can see it.(see our output test cases)
cout << //----;
1.5 Step 5: Write codes for Case #2 (Write a for loop)
User chooses option 2 in order to compute the factorial of a given integer. For calculating the value of the factorial, we need to have two variables: n, which is the integer entered by the user, and fact, which is the value of the factorial.
//declare two variables of type int, one is n, another one is fact //make sure you intialize fact to be 1 //----
Ask the user to enter a number by using cout to show a user prompt message on screen, then use cin to get the number and store it inside variable n. Next, to compute n!, which is 1*2*3*...* (n-2) * (n-1) * n, we can write a for loop as follows:
for(int i = 1; //----counter i less or equals to n; //----increase i by 1) { fact = fact*i; }
After above for loop terminate, you will need to print the value of fact so that user can see it. (see our output test cases)
cout << //----;
1.6 Step 6: Write codes for Case #3 (Write a while loop)
The user chooses option 3 in order to get the first digit of a given number. To calculate this, we need to have two variables, num, which is the integer entered by the user, and rem, which stores the value of reminder when num is divided by 10. Ask the user to enter a number using cin and store the value entered by the user inside variable num using a cin. The logic is that we will divide the number until the quotient becomes 0 and store the reminder at every iteration. At the end of each iteration, the reminder will have the 1st integer from the right from the previous iteration. We need to use a while loop to perform the operation, since we dont know how big the number is ahead of times and the terminating condition would be num = 0.
while(//as long as num is not equal to 0) { //compute rem, it should equal to num modulo 10 (num%10) //---- //change num, it should equal to itself divided by 10 //---- } //at every iteration rem will have the 1st digit from left for an integer */
After the while loop terminate, print the value of rem as the 1st integer from left using cout.
cout << //----;
2. Sample Run of the Program
Below is an example of what your output should roughly look like when this lab is completed. Note: user input is bold 1. Calculate the sum of 1 to m integers 2. Calculate the factorial of given number 3. Find the first digit of a given number 4. Quit
Please choose from above menu: 1 Enter the number: 4 The sum of 1 to 4 is 10
1. Calculate the sum of 1 to m integers 2. Calculate the factorial of given number 3. Find the first digit of a given number 4. Quit
Please choose from above menu: 2 Enter the number: 5
The factorial of 5 is 120 1. Calculate the sum of 1 to m integers 2. Calculate the factorial of given number 3. Find the first digit of a given number 4. Quit
Please choose from above menu: 3 Enter the number: 987654321
The leftmost digit is 9 1. Calculate the sum of 1 to m integers 2. Calculate the factorial of given number 3. Find the first digit of a given number 4. Quit
Please choose from above menu: 4
Step by Step Solution
There are 3 Steps involved in it
Step: 1
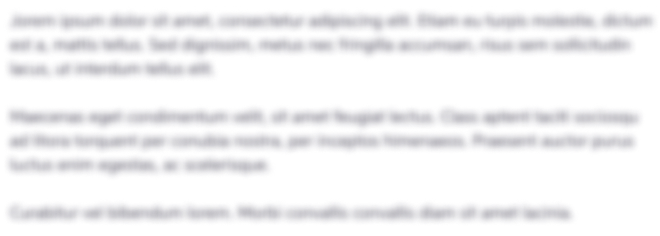
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started