Question
Each member in your group will have to implement two classes (a Location subclass and a Players ubclass). Your team is free to add more
Each member in your group will have to implement two classes (a Location subclass and a Players ubclass). Your team is free to add more to the game if you wish but that will not be necessary.
Assigned to **HOME (location) and HELPER (players)**.
Locations
The world that the game takes place in consists of different locations. A location can have zero or more players in it, zero or more peaches in it, and it may have some behaviour of its own.
Each member will implement a location subclass.
1) A Home is the home location. There is only one home in each world. This is where several of the players will begin the game. Is is a place for players to drop off (to stockpile) peaches. The home keeps track of how many peaches each player brings back.
The home will also create a new Helper player to go help another player in distress (low health). It will give the helper the location of the player in distress and some peaches to give to the distressed player to eat.
2) A PeachGrove has a bunch of peach trees, zero or more peaches (from the trees) and perhaps some bees (that may sting a player). When a player enters a peach grove for the n-th time then n bees might sting that person each with a 50% probability. Each sting will result in 5 health points being lost to the player. If the player doesn't die from bee stings then they can eat and take away as many peaches as they are able (if there are that many available). A grove does not grow more peaches as the game progresses.
3) A PeachPit is a location with a large hole. When a player enters (falls into) the pit, they lose 1/2 of their health points. (Just use integer division here.)
A peach pit also remembers who has already fallen into the pit. When a player falls in for the second (or more) time, they are instantly transported to the Home location, lose 1/2 their health points and their turn ends.
There may initially be several peaches in the pit. A player that falls in for the first time may take them (to eat or carry).
4) A BearsDen has a bear in it. If a player offers a bear two or more peaches then the bear will leave the
player alone (do nothing). If the player does not give two or more peaches to the bear then the bear will attack the player resulting in 25 health points being deducted. The bear will remember each player that gave it peaches and will not take peaches again or attack that player again. Players Each group member will implement a player subclass. 1) A PeachHunter searches for peaches to bring back to the home location. A peach hunter can carry lots of peaches (as many as 100). The peach hunter should return to home to deposit its peaches once they have 50 or more peaches. A peach hunter will remember where peach groves are when they find them and go back to them to get more peaches until the grove runs out. If a hunter's health is less than 50 then it can only carry 25 peaches. It must then put any excess peaches in the current location if this happens. 2) A PitFinder looks for peach pits and remembers where they are (locations). Each time a piut finder finds a new pit it will go back home and report this to the home (when the player enters home). When a pit finder and peach hunter interact, a finder will reveal the location of a pit to the finder in exchange of 5 peaches. A finder with health less then 30 can only carry 10 peaches. If their health drops down to this (if they fall in a pit or attacked by a bear for example) they must drop any excess peaches in the current location. 3) A SmartyPants just wanders around the world trying to sell advice to others. A smarty pants is given knowledge of all peach pits, bear dens and peach groves in the world. When it interacts with peach hunters they will reveal a grove's location for 7 peaches; when they interact with pit finders they will reveal a pit or bears den for 6 peaches; and when they interact with a thief they will steal all the theirs peaches (instead of the thief stealing theirs). There is only one smarty pants in the world. If a hunter or finder do not have enought peaches then no transaction is made. A smarty pants is fair to peach hunters and pit finders. They will not reveal the same location twice to the same player (unless they already revealed all of the locations). 4) A Helper is created and dispatched from the home location whenever a player (pit finder or peach hunter) asks for help. They bring a bunch of peaches to the player (giving them to the player when they interact with them). Once a helper helps someone they go back home (and do nothing for the rest of the game). A helper will not give any peaches to a bear. 5) A PeachThief that will try to steal peaches from other players, as well as pick up peaches it finds along the way. When it interacts with a hunter or pit finder, it will repeatedly steal peaches (with probability 75%) until it fails to steal one (or the other player runs out of peaches). A thief will immediately eat the first peach successfully stolen in any interaction. At any time (during the play method) a player can eat a peach they have. This will rejuvenate them by adding |
some health equal to the ripeness of the peach. So if a peach has ripeness 10 then eating it will give the player 10 health points. However, if the peach is bad, then it will deduct the value of the ripeness from their health instead.
If a player's health ever drops below 10 they can call home for help. The Home loction will dispatch aHelper player to send some peaches to eat.
Peaches Adventure
The provided PeachesGame program is a simple starting point for the game. Essentially, it lets each player in the world have their turn (play method) and repeats until the home location has accumulated enuogh peaches.
Classes
*Directions.java*
public class Directions{ public static final int UP = 0; public static final int DOWN = 1; public static final int LEFT = 2; public static final int RIGHT = 3; }
*EmptyLocation.java*
import java.util.ArrayList;
public class EmptyLocation extends Location{ public EmptyLocation(Position p, String description){ super(p, description, new ArrayList
*Location.java*
import java.util.List;
public class Location{ protected Position position; protected String description = "Nothing special about this location."; protected List
*Peach.java*
public class Peach implements Comparable
public Peach(int ripeness, boolean bad){ this.ripeness = ripeness; this.bad = bad; } public Peach(int ripeness){ this(ripeness, false); } public int getRipeness(){ return ripeness; } /** ages a peach in some way */ public void age(){} @Override public int compareTo(Peach other){ return 0; } }
*PeachesGame.java*
import java.util.ArrayList;
public class PeachesGame{ public static void main(String[] args){ World w = new World(); Player p = new Player(w, "cat", w.home, new ArrayList
*Player.java*
import java.util.List;
/** A Player in the game * * Each member of your team should implement their own * unique Player subtype. Your group should also have a human player. */
public class Player{ protected World world; // world that player lives in protected String name; // name of player protected Location location; // where in the world the player is protected List
/** Getter for a player's name */ public String getName(){ return name; } /** Getter for a player's location */ public Location getLocation(){ return location; } /** Getter for a player's peach */ public Peach getPeach(){ return peaches == null ? null : peaches.remove(0); } /** Getter for a player's health */ public int getHealth(){ return health; } /** This is the logic of the player. * It defines what they should do when given a chance to do somerthing */ public void play(){ if( health < 10 ){ getHelp(); return; } } /** Moves a player from one location to a new location * * @param newLocation is the new location that the player will be moved to * @return true if the move was successful and false otherwise (e.g. when trying to move from one * location to another that are not connected) */ public boolean move(int direction){ // move from current location to new location (if possible) world.move(this, direction); return false; } /** sets a player's current location * * @param location is the Location to set for the player */ public void setLocation(Location location){ this.location = location; }
/** Setter for a player's health * * @param h is the new health of the player */ public void setHealth(int h){ this.health = h; } /** Allows for interaction with this player and another player * (presumably called from within the play method) * * @param p is a player that is interacting with this player */ public void interact(Player p){ // allows for some interaction with a player } /** ask for help when they need it */ public void getHelp(){ world.getHome().callForHelp(this, location); } @Override public String toString(){ return name; } /** Two players are the same if they have the same name, location and health. */ @Override public boolean equals(Object o){ if( o instanceof Player){ return this.name.equals( ((Player)o).name ) && this.location.equals( ((Player)o).location ) && this.health == ((Player)o).health; }else{ return false; } }
}
*Position.java*
public class Position{ protected int x; protected int y; public Position(int x, int y){ this.x = x; this.y = y; } public int getX(){ return x; } public int getY(){ return y; } @Override public String toString(){ return "["+x+","+y+"]"; } }
*RGB.java*
public class RGB{ public static RGB RED = new RGB(255,0,0); public static RGB GREEN = new RGB(0,255,0); public static RGB BLUE = new RGB(0,0,255); public static RGB YELLOW = new RGB(255,255,0); public static RGB MAGENTA = new RGB(255,0,255); public static RGB CYAN = new RGB(0,255,255); protected int red; protected int green; protected int blue; public RGB(int red, int green, int blue){ this.red = red; this.green = green; this.blue = blue; } public RGB(int gray){ this(gray,gray,gray); } }
*World.java*
import java.util.List; import java.util.ArrayList; import java.util.Arrays;
public class World{ protected Location[][] locations; protected Location home; protected List
Step by Step Solution
There are 3 Steps involved in it
Step: 1
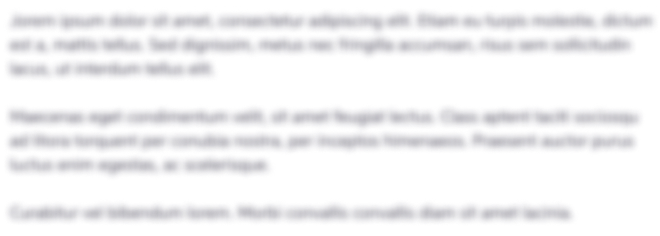
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started