Question
----- ElectionLab4 java import java.util.ArrayList; public class ElectionLab4 { public static void main(String args[]) throws CandidateNotFoundException,MinimumAgeException,MissingCandidatesException{ ArrayList candids = new ArrayList(3); Candidate candid; try{ candid
-----
ElectionLab4 java
import java.util.ArrayList;
public class ElectionLab4 { public static void main(String args[]) throws CandidateNotFoundException,MinimumAgeException,MissingCandidatesException{ ArrayList candids = new ArrayList(3); Candidate candid; try{ candid = new Candidate(55, 'M', "Tommy", "Scott", "Non-Affiliate"); candids.add(candid); candid = new Candidate(60, 'M', "King", "Pound", "Democrat"); candids.add(candid); candid = new Candidate(75, 'F', "Sally", "Adams", "Republican"); candids.add(candid); } catch(MinimumAgeException e){ System.out.println(e); } VotingMachine vm = new VotingMachine(candids); Voter vot[] = new Voter[10]; try{ vot[0] = new Voter(1, 30, 'M', "John", "Smith", "Non-Affiliate"); vot[1] = new Voter(1, 42, 'F', "Adams", "Evan", "Republican"); vot[2] = new Voter(1, 65, 'F', "Jim", "Smith", "Republican"); vot[3] = new Voter(1, 70, 'M', "Alice", "Zens", "Non-Affiliate"); vot[4] = new Voter(1, 29, 'F', "Boss", "Tony", "Republican"); vot[5] = new Voter(1, 33, 'M', "Tommy", "Bright", "Non-Affiliate"); vot[6] = new Voter(1, 38, 'F', "Thomas", "Sams", "Democrat"); vot[7] = new Voter(1, 46, 'M', "Black", "Mills", "Democrat"); vot[8] = new Voter(1, 28, 'M', "William", "Hall", "Democrat"); vot[9] = new Voter(1, 31, 'F', "Paul", "Barn", "Non-Affiliate"); } catch(MinimumAgeException e){ System.out.println(e); } vm.vote(vot[0],candids.get(0)); vm.vote(vot[1],candids.get(0)); vm.vote(vot[2],candids.get(2)); vm.vote(vot[3],candids.get(1)); vm.vote(vot[4],candids.get(1)); vm.vote(vot[5]); vm.vote(vot[6],candids.get(0)); vm.vote(vot[7],candids.get(1)); vm.vote(vot[8]); vm.vote(vot[9],candids.get(1)); vm.tally(); } } ------
Lab 3 code
//Program to evaluate the concept of inheritance as the candidate class and voter class are child classes so they can access the methods of parent class package CJ;
abstract class Person {
private int age; private char gender; protected String firstName; protected String lastName; protected String politicalParty;
//Default constructor public Person() { // TODO Auto-generated constructor stub } //constructor public Person(int age, char gender, String firstName, String lastName, String politicalParty) { this.age = age; this.gender = gender; this.firstName = firstName; this.lastName = lastName; this.politicalParty = politicalParty; } //getter methods public int getAge() { return age; }
public char getGender() { return gender; } public abstract String getFullName();
} class Candidate extends Person {
private int voteCount = 0;
public Candidate() { // TODO Auto-generated constructor stub } public Candidate(int age, char gender, String firstName, String lastName, String politicalParty) { super(age, gender, firstName, lastName, politicalParty); }
public int getVoteCount() { return voteCount; }
public void incrementVoteCOunt() { voteCount++; } //override the abstract method @Override public String getFullName() { String name = firstName + " " + lastName; name += (politicalParty.equals("Democrat") || politicalParty.equals("Republican")) ? "-" + politicalParty.charAt(0) : "-NA"; return name; }
} class Voter extends Person {
private int voterId; private boolean voted;
public Voter() { // TODO Auto-generated constructor stub }
public Voter(int age, char gender, String firstName, String lastName, String politicalParty) { super(age, gender, firstName, lastName, politicalParty); }
public boolean hasVoted() { return voted; }
public void voted() { this.voted = true; } public int getVoterId() { return voterId; }
@Override public String getFullName() { return firstName + " " + lastName; }
} class VotingMachine {
public Candidate[] candidates;
public VotingMachine(Candidate[] candidates) { this.candidates = candidates; }
public void vote(Voter v, Candidate c) { c.incrementVoteCOunt(); v.voted(); }
public void vote(Voter v) { v.voted(); }
public void tally() { for (Candidate c : candidates) { System.out.println(c.getFullName() + " has " + c.getVoteCount() + " votes."); } int winner = 0; for (int i = 1; i
System.out.println( candidates[winner].getFullName() + " won with " + candidates[winner].getVoteCount() + " votes."); } }
public class LabProgram {
public static void main(String[] args) { //three candidate objects Candidate c1 = new Candidate(45, 'M', "Sam", "Smith", "Republican"); Candidate c2 = new Candidate(40, 'M', "Joe", "Dean", "Democrat"); Candidate c3 = new Candidate(47, 'M', "Jane", "Doe", "Non-Affliate"); Candidate[] candidates = { c1, c2, c3 }; //Voting Machine Object VotingMachine votingMachine = new VotingMachine(candidates); //votes votingMachine.vote(new Voter(), c1); votingMachine.vote(new Voter(), c2); votingMachine.vote(new Voter(), c1); votingMachine.vote(new Voter(), c1); votingMachine.vote(new Voter(), c3); votingMachine.vote(new Voter(), c3); votingMachine.vote(new Voter(), c3); votingMachine.vote(new Voter(), c1); votingMachine.vote(new Voter(), c3); votingMachine.vote(new Voter(), c1); votingMachine.vote(new Voter(), c3); votingMachine.vote(new Voter(), c3); votingMachine.vote(new Voter(), c2); votingMachine.vote(new Voter(), c3); votingMachine.tally();
}
}
Lab 4 - Exceptions Handling Introduction This project is an extension of Lab 3 which was about designing an object-oriented voting system, written in java, that will illustrate the principles of object-oriented programming. With this lab we will illustrate the use of exceptions. Classes We will be enhancing the classes which we created before (changes in highlighted in yellow), as well as adding new classes for exceptions (this will hold the custom exceptions we defined). Person (No changes) This will be an abstract class. In this class we have used the concept of inheritance as the candidate class and voter class are child classes so they can access the methods of parent class. Must have the following variables: age (int) - private gender (char) - private, will hold values like M, F, T, U firstName (string) - protected lastName (string) - protected politicalParty (string) - protected, will hold values like Democrat, Republican, Non-Affiliate Must have the defined constructor: Person (). Person (int age, char gender, String firstName, String lastName, String political Party) Which will set the passed properties to our defined variables Will implement the following methods: .getAge() - returns int getGender() - returns char Will define the following abstract methods: getFullName() - returns string Candidate This class holds the information about the candidate it will extend the class Person. Must have the following variables: voteCount (int) - private, when the class is instantiated this should default to 0 Must have the defined constructor: Candidate() Candidate (int age, char gender, String firstName, String lastName, String political Party) Which will set the passed properties to our defined variables and super class Throws exception MinimumAgeException if candidate's age is less than 25 The message is Candidate's age cannot be less than 25" Which will set the passed properties to our defined variables and super class Will implement the following public methods: getVoteCount() - returns int incrementVoteCount() - void, increments the vote Count .getFullName() - returns string o If the candidates firstName = "John and lastName = "Smith and political party is Democrat" then it returns "John Smith - D o If the candidates firstName = "John" and lastName = "Smith and political party is Republican" then it returns John Smith - R o If the candidates firstName = John and lastName = "Smith and political party is Non-Affiliate then it returns John Smith - NA o Otherwise "John Smith" Voter This class will gather the information about the voter it will extend the class Person. Must have the following variables:pub voterld (int) - private voted (boolean) - private, when the class is instantiated this should default to false Must have the defined constructor: Voter () Voter (int voterld, int age, char gender, String firstName, String lastName, String politicalParty) Throws exception MinimumAgeException if voter's age is less than 18 The message is Voter's age cannot be less than 18 Which will set the passed properties to our defined variables and super class Will implement the following public methods: getVoterld() - returns int hasVoted() - returns boolean voted() - void, sets the voted flag to true getFullName() - returns string - e.g. firstName = John" and lastName = "Smith then it returns "John Smith" Voting Machine This class is used to manipulate and print the results (i.e. who is the winner and how many votes he/she gained). This class has crucial role in whole system Must have the following variables: candidates (Candidate[]) - public, array of all candidates in the race candidates (ArrayListStep by Step Solution
There are 3 Steps involved in it
Step: 1
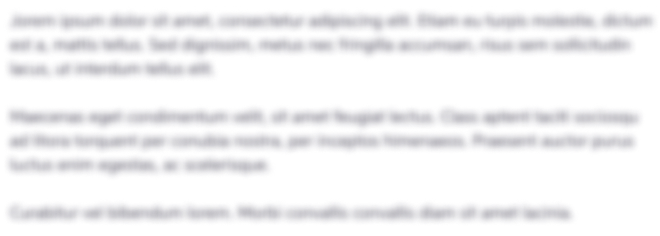
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started