Question
EmpBinaryTree.h #ifndef EMPBINARYTREE_H #define EMPBINARYTREE_H #include Employee.h #include class EmpBinaryTree { private : struct TreeNode { Employee e; TreeNode *left; TreeNode *right; }; TreeNode *root;
EmpBinaryTree.h
#ifndef EMPBINARYTREE_H #define EMPBINARYTREE_H #include "Employee.h" #includeclass EmpBinaryTree { private: struct TreeNode { Employee e; TreeNode *left; TreeNode *right; }; TreeNode *root; void insert(TreeNode *&, TreeNode *&); void destroySubTree(TreeNode *); void deleteNode(Employee, TreeNode *&); void makeDeletion(TreeNode *&); void displayInOrder(TreeNode *) const; void displayPreOrder(TreeNode *) const; void displayPostOrder(TreeNode *) const; public: // Constructor EmpBinaryTree() { root = nullptr; } // Destructor ~EmpBinaryTree() { destroySubTree(root); } // Binary tree operations void insertEmployee(Employee); bool searchEmployee(Employee); Employee getEmployee(string); void remove(Employee); void displayInOrder() const { displayInOrder(root); } void displayPreOrder() const { displayPreOrder(root); } void displayPostOrder() const { displayPostOrder(root); } }; #endif
Employ.h
// Specification file for the Employee class #ifndef EMPLOYEE_H #define EMPLOYEE_H #includeusing namespace std; // Exception class class InvalidEmployeeNumber {}; class Employee { private: string name; // Employee name string number; // Employee number string hireDate; // Hire date public: // Default constructor Employee() { name = ""; number = ""; hireDate = ""; } // Constructor Employee(string aName, string aNumber, string aDate) { // Test aNumber... int num = atoi(aNumber.data()); if (num >= 0 && num <= 9999) { // aNumber is valid. name = aName; number = aNumber; hireDate = aDate; } else throw InvalidEmployeeNumber(); } // Mutators void setName(string n) { name = n; } void setNumber(string aNumber) { int num = atoi(aNumber.data()); if (num >= 0 && num <= 9999) number = num; else throw InvalidEmployeeNumber(); } void setHireDate(string date) { hireDate = date; } // Accessors string getName() const { return name; } string getNumber() const { return number; } string getHireDate() const { return hireDate; } }; #endif /* EMPLOYEE_H_ */
EmployeeDB.h
#ifndef EMPLOYEEDB_H_ #define EMPLOYEEDB_H_ #include "EmpBinaryTree.h" #includeclass EmployeeDB{ private: EmpBinaryTree db; public: EmployeeDB(); void insertEmployee(Employee); bool deleteEmployee(Employee); Employee searchEmployee(string); void displayRecords(); }; #endif /* EMPLOYEEDB_H_ */
main.cpp
#include
#include
#include "EmployeeDB.h"
using namespace std;
int main() {
EmployeeDB db;
for (int i=0;i<100;i++){
Employee e;
std::string name = "Employee" + to_string(i);
e.setName(name);
std::cout << "Inserting: " << e.getName() << std::endl;
db.insertEmployee(e);
}
std::cout << " Display Records" << std::endl;
db.displayRecords();
Employee temp = db.searchEmployee("Employee99");
std::cout << "Deleting: Employee99" << std::endl;
db.deleteEmployee(temp);
std::cout << " Display Records" << std::endl;
db.displayRecords();
return 0;
}
employee database to store employee records.
The EmployeeDB class will store employee records using a binary search tree.
The EmployeeDB will have the following methods:
- insertEmployee: This method takes a single employee as an argument and inserts the
employee into the BST.
-deleteEmployee: This method takesa (temp) employee as an argument then deletes the Employee from the BST with a matching name.
- searchEmployee: This method accepts a string as an argument, then searches the BST for an Employee with a name matching this string argument.
- displayRecords: This method prints the name of every Employee using an InOrder BST traversal.
See the provided Employee Class in Employee.h.
No changes required.
See the provided EmpBinaryTree specification
Complete the class in the separate Employee.cpp file.
See the provided EmployeeDB specification.
Complete the class in the separate EmployeeDB.cpp file
See the provided driver in main.cpp. This is only an example. Write own driver.
Create driver to do the following:
Provide a looping menu with the following options:
- Insert New employee. This creates a new employee, prompts for employee info, updates the employee, inserts this employee into the database.
- Delete Employee: This prompts the user for the name of the employee to search for then deletes the Employee with this name.
- Search for an employee: Prompt the user for a name to search for. If the Employee exists, print out all of the information about this Employee. Otherwise: Print error message.
- Display all of the employee records: Display all of the records to the screen using the displayInOrder() method from the BST.
The provided Employee.h, EmpBinaryTree.h, EmployeeDB.h may not be modified.
Complete the EmpBinaryTree class, then complete the EmployeeDB class, then create useful driver application.
Test and demonstrate your application and provide useful screenshots.
Submit all source files and screenshots to Blackboard before the deadline.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
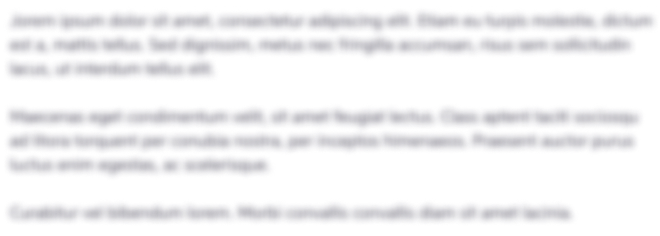
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started