Question
Employee Class Write a class named Employee that has the following fields: name. The name field is a String object that holds the employees name.
-
Employee Class
Write a class named Employee that has the following fields:
-
name. The name field is a String object that holds the employees name.
-
idNumber. The idNumber is an int variable that holds the employees ID number.
-
department. The department field is a String object that holds the name of the department where the employee works.
-
position. The position field is a String object that holds the employees job title.
Write appropriate mutator methods that store values in these fields, and accessor methods that return the values in these fields. Once you have written the class, write a separate program that creates three Employee objects to hold the following data:
Name ID Number Department Position Susan Meyers 47899 Accounting Vice President Mark Jones 39119 IT Programmer Joy Rogers 81774 Manufacturing Engineer The program should store this data in the three objects, then display the data for each employee on the screen.
-
Below is my coding:
class employee
{
private String name;
private int idNumber;
private String department;
private String position;
public employee()
{
this.name="Susan Meyers";
idNumber=47899;
department="Accounting";
position="Vice President";
}
public employee(String n,int id, String d, String p)
{
this.name=n;
this.idNumber=id;
this.department=d;
this.position=p;
}
public employee(employee other)
{
name=other.name;
idNumber=other.idNumber;
department=other.department;
position=other.position;
}
public void setName(String n)
{
this.name=n;
}
public void setID(int d)
{
this.idNumber=d;
}
public void setDepartment(String d)
{
this.department=d;
}
public void setPosition(String p)
{
this.position=p;
}
public String getName()
{
return this.name;
}
public int getID()
{
return this.idNumber;
}
public String getDepartment()
{
return this.department;
}
public String getPosition()
{
return this.position;
}
public String toString()
{
System.out.println("Employee: "+getName()+" ID: "+getID()+" Department: "+getDepartment()+" Position: "+getPosition());
return"Employee: "+name+" ID: "+idNumber+" Department: "+department+" Position: "+position;
}
}
import java.util.*;
import javax.swing.*;
class EmployeeDemo
{
static Scanner in = new Scanner(System.in);
public static void main(String args[])
{
String name,dept,position;
employee employee1=new employee();
employee employee2=new employee("Mark Jones",39119,"IT","Programmer");
employee employeetocopy=new employee("Joy Rogers",81774,"Manufacturing","Engineer");
employee employee3=new employee(employeetocopy);
employee1.toString();
employee2.toString();
employee3.toString();
}
}
Read the feedback:
Verify your code using the EmployeeDemo.java
The class name needs to be Employee not employee. Capital E in Employee.
Constructors. They are not required. If you do create them you need to create one that accepts not argumnent.
The method name must match. setIdNumber
Submission is also lacking comments thoug out the code.
Formatting issues with indentation.
**PLEASE MAKE NECESSARY CHANGES**
Step by Step Solution
There are 3 Steps involved in it
Step: 1
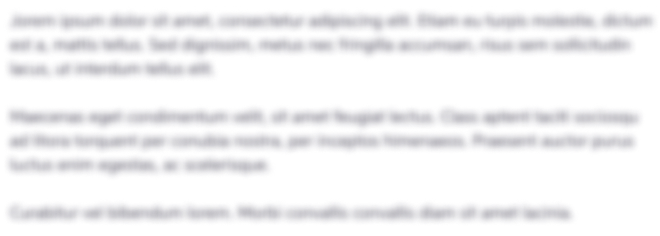
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started