Question
estimating efficiency of backtracking sudoku solver using monte carlo estimate here is a link to a textbook talking about backtracking and how to estimate the
estimating efficiency of backtracking sudoku solver using monte carlo estimate
here is a link to a textbook talking about backtracking and how to estimate the efficiency of backtracking algorithms using monte carlo estimation:
http://apprize.info/science/algorithms/5.html
here is the python code for a backtracking sudoku puzzle solver(just the main algorithm):
def isFull(board): for x in range(0, 9): for y in range(0, 9): if board[x][y] == 0: return False return True # function to find all of the possible numbers # which can be put at the specifies location by # checking the horizontal and vertical and the # three by three square in which the numbers are # housed def possibleEntries(board, i, j): possibilityArray = {} for x in range(1, 10): possibilityArray[x] = 0 # For horizontal entries for y in range(0, 9): if not board[i][y] == 0: possibilityArray[board[i][y]] = 1 # For vertical entries for x in range(0, 9): if not board[x][j] == 0: possibilityArray[board[x][j]] = 1 # For squares of three x three k = 3 * floor(i / 3) l = 3 * floor(j / 3) for x in range(k, k + 3): for y in range(l, l + 3): if not board[x][y] == 0: possibilityArray[board[x][y]] = 1 for x in range(1, 10): if possibilityArray[x] == 0: possibilityArray[x] = x else: possibilityArray[x] = 0 return possibilityArray # recursive function which solved the board and # prints it. def sudokuSolver(board): i = 0 j = 0 possiblities = {} # if board is full, there is no need to solve it any further if isFull(board): print("Board Solved Successfully!") printBoard(board) return else: # find the first vacant spot for x in range(0, 9): for y in range(0, 9): if board[x][y] == 0: i = x j = y break else: continue break # get all the possibilities for i,j possiblities = possibleEntries(board, i, j) # go through all the possibilities and call the the function # again and again for x in range(1, 10): if not possiblities[x] == 0: board[i][j] = possiblities[x] file.write(printFileBoard(board)) sudokuSolver(board) # backtrack board[i][j] = 0
=============================================================================
the entire code for the program can be found here:
https://www.dropbox.com/s/5ssvfh61yzvw2p3/Sudoku.txt?dl=0
what i want you to do is write the code for estimating the efficiency of this backtracking algorithm using monte carlo estimation.
im on a tight deadline, help would be much appreciated.
thank you very much
Step by Step Solution
There are 3 Steps involved in it
Step: 1
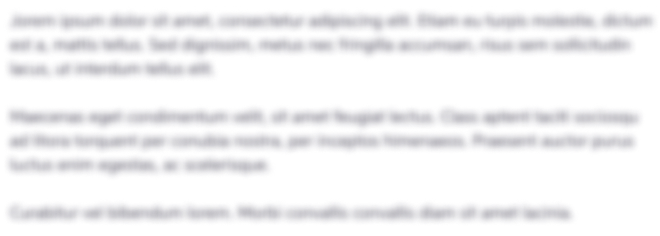
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started