Answered step by step
Verified Expert Solution
Question
1 Approved Answer
//Example program #include #include #include using namespace std; const float WOLF_PRICE = 55.00; const float RABBIT_PRICE = 15.00; const float STEAK_PRICE = 12.00; //all of


//Example program
#include
#include
#include
using namespace std;
const float WOLF_PRICE = 55.00;
const float RABBIT_PRICE = 15.00;
const float STEAK_PRICE = 12.00;
//all of the above are measured in $/yd^3
//---------------------------------------------------------------------------
// Name: GetYorN
// Parameters: Questions, string, input, the YN question to ask
// Returns: char; the character the user entered. Must be 'y' or 'Y' or 'n' or 'N'
// Purpose: This function returns the user's response to a yeso question
//---------------------------------------------------------------------------
char GetYorN(const string Question)
{
char choice;
cout
cin >> choice;
choice = toupper(choice);
while (choice != 'Y' && choice != 'N')
{
cout
cout
cin >> choice;
choice = toupper(choice);
}
return (choice);
}
//---------------------------------------------------------------------------
// Name: GetLoadChoice
// Parameters: reference char choice
// Returns: none (passed in via reference), Must be 'R', 'W', or 'S'
// Purpose: Determine which of the items the user would like to ship
//
//---------------------------------------------------------------------------
void GetLoadChoice(char &choice)
{
cout
cout
cin >> choice;
choice = toupper(choice);
while(choice != 'W' && choice != 'S' && choice != 'R')
{
cout
cout
" (W)olves"
" (S)teak"
" (R)abbits ";
cin >> choice;
choice = toupper(choice);
}
}
//---------------------------------------------------------------------------
// Name: AddLoad
// Parameters: const char loadChoice - the type of item to be shipped
// Returns: float cost
// Purpose: Find the (positive integer) quantity of yards to be shipped, then
// add the appropriate price to the total
//---------------------------------------------------------------------------
float AddLoad(const char loadChoice)
{
float cost = 0.0;
int quantity = 0;
cout
cin >> quantity;
while(quantity
{
cout = 0. ";
cout
cin >> quantity;
}
if (loadChoice == 'W')
cost = cost + quantity * WOLF_PRICE;
else if (loadChoice == 'S')
cost = cost + quantity * STEAK_PRICE;
else
cost = cost + quantity * RABBIT_PRICE;
return cost;
}
//---------------------------------------------------------------------------
// Name: PrintMain
// Parameters: none
// Returns: none
// Purpose: Prints the main menu for the shipping scheduler
//---------------------------------------------------------------------------
void PrintMain()
{
cout
}
//---------------------------------------------------------------------------
// Name: RefrigeratedCar
// Parameters: int &numRCars; add one to the number of refigerated cars used
// Returns: 150 if the user requests a refrigerated car, 0 otherwise.
// Purpose: Ask if the user wants a refrigerated car and return the additional
// cost if so, 0 otherwise.
//---------------------------------------------------------------------------
int RefrigeratedCar(int &numRCars)
{
char wantRefrigeratedCar;
int cost = 0;
// If the user answers 'Y', increment the number of refrigerated cars used
wantRefrigeratedCar = GetYorN("Do you require a refrigerated car? ");
if (wantRefrigeratedCar == 'Y')
{
numRCars++;
cost = 150;
}
return cost;
}
//---------------------------------------------------------------------------
// Name: IsValidOrder
// Parameters: const char firstLoad, const char secondLoad, const int NumRCars
// Returns: true if the order is valid, false otherwise
// Purpose: check the loads to ensure that rabbits and wolves aren't
// being shipped together, and ensure that the user ordered a
// refrigerated car if they are shipping steak. If any violation
// occured, tell the user which and return false. Otherwise, return true.
//---------------------------------------------------------------------------
bool IsValidOrder(const char firstLoad, const char secondLoad, const int numRCars)
{
bool isValid = true;
// If there is anything wrong, change isValid to false
if(firstLoad == 'W' && secondLoad == 'R')
{
cout
isValid = false;
}
if(firstLoad == 'R' && secondLoad == 'W')
{
cout
isValid = false;
}
if((firstLoad == 'S' || secondLoad == 'S') && (numRCars == 0))
{
cout
isValid = false;
}
return isValid;
}
//---------------------------------------------------------------------------
// Name: PrintBill
// Parameters: const float totalCost - the cost of all the shipments
// const int numRCars - the number of refigerator cars
// Returns: void
// Purpose: Prints the bill
//---------------------------------------------------------------------------
void PrintBill(const float totalCost, const float numRCars)
{
cout 1. Problem Statement: The objective of this programming assignment is to become familiar with calling C++ functions. You will be modifying an existing program: hw4a.cpp. Your task is to write a main program to accomplish certain goals by calling functions that are already written and provided to you Aesop Shipping LLC is a freight company that schedules loads of three commodities live arctic wolves, live arctic rabbits, and frozen steak. Due to the nature of the shipments, some restrictions are in place Orders consist of 1 or more shipments Each shipment consists of a pair of loads . Each load consists of one of the three commodities Live wolves and rabbits cannot be shipped in the same shipment, for obvious reasons Steak requires refrigerated cars (the animals won't mind, they're arctic). Your task is to use the provided functions to write a main function that will prompt the user for the information you will need to calculate the total bill. The variables, and functions are already provided. Your task is to get what and in what quantity the user wants to ship, ensure that all restrictions are not being violated, and print the total cost of the shipment if it is valid. prices, necessary 2. Design Once you have an idea of what needs to be done, think about how you can use the provided functions in your main) function, which actual parameters need to be passed to the functions, the type of parameters that are being used and how you can use the return values A skeleton of the main program is included with comments showing the necessary steps where work needs to be provided. The already declared, therefore all you need to do is use them in your work. necessary variables for your program are Do not change the functions themselves
}
//---------------------------------------------------------------------------
// Name: YouAreFinished
// Parameters: none
// Returns: bool: true if finished, false if not finishted
// Purpose: Prints the main menu for the shipping scheduler
//---------------------------------------------------------------------------
bool YouAreFinished()
{
char choice;
choice = GetYorN(" Do you want to schedule another shipment? ");
// if the user entered 'N', then you are finished
return (choice == 'N');
}
int main()
{
//shipment variables
char firstLoad = '\0'; // type of the first load in a shipment
char secondLoad = '\0'; // type of the second load in a shipment
int shipmentRefrigCars = 0; // number of refrigerator cars for this shipment
float shipmentRefrigCost = 0.0; // cost of the refrigerator cars for this shipment
float shipmentCost = 0.0; // cost of this shipment
//total order variables
int numRefrigCars = 0; // number of refrigerator cars total
float totalCost = 0.0; // total cost of all shipments
bool done = false; // whether or not the user is done shipping stuff
//Loop until the user is done
//initialize the shipmentRefrigCars, shipmentRefrigCost and shipmentCost variables
//Print the main menu
//Ask if the user needs a refrigerated car and store the number and cost of the
//refrigerator car (if any) in the appropriate shipment variables
//Find out what the user wants to in the first load
//Set the load cost to the cost of the first load
//Get the user's second choice of load
//Add the user's second load cost to the shipment cost
//If the order is valid,
// add the shipment cost and the refrigerator car cost to the total cost
// add the number of shipment refrigerator cars to the total number of refrigerator cars
//else
// inform the user that their shipment has been cancelled
//Ask the user whether or not they want to continue (Y or N)
//output the total cost and the number of refrigerator cars used
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
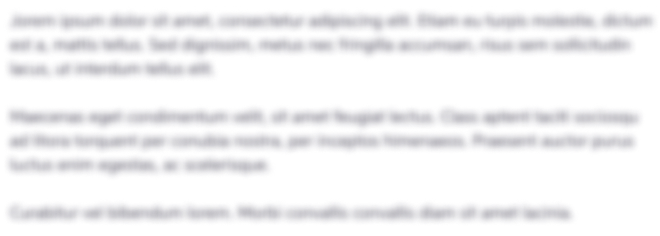
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started