Question
EXISTING CODE class Node: def __init__(self, data, next=None): self.data = data self.next = next def get_data(self): return self.data def get_next(self): return self.next def set_data(self, new_data):
EXISTING CODE
class Node: def __init__(self, data, next=None): self.data = data self.next = next def get_data(self): return self.data def get_next(self): return self.next def set_data(self, new_data): self.data = new_data def set_next(self, new_next): self.next = new_next def add_after(self, value): new_node = Node(value,self.next ) self.next = new_node def remove_after(self): self.next = self.next.get_next() def __str__(self): return str(self.data)
class LinkedList: def __init__(self): self.head = None self.size = 0 def __iter__(self): return LinkedListIterator(self.head) def add(self, item): #add to the beginning of the list new_node = Node(item, self.head) self.head = new_node self.size += 1 def __len__(self): return self.size def is_empty(self): return self.head == None def __str__(self): if self.head != None: result = "Head: " current = self.head while current != None: result = result + str(current) + " -> " current = current.next return result + "None" else: return "Head: " class LinkedListIterator: def __init__(self, head): self.current = head def __next__(self): if self.current == None: raise StopIteration else: item = self.current.get_data() self.current = self.current.get_next() return item class LinkedListHashTable: def __init__(self, size = 7): self.__size = size self.__slots = [LinkedList()] * size def get_hash_code(self, key): return key % self.__size def __str__(self): return ' '.join('{}'.format(self.__slots[index]) for index in range(self.__size)) def put(self, key): def __len__(self):
hash_table = LinkedListHashTable(5) hash_table.put(3) hash_table.put(6) hash_table.put(9) hash_table.put(11) hash_table.put(21) hash_table.put(13) print(hash_table) print(f"The linked list hash table "+ f"contains {len(hash_table)} items.")Continuing on from the previous question, add the following methods to the LinkedListHashTable class: - The put (self, key ) method that takes an integer as a parameter and inserts the parameter key into a hash table. This method should use separate chaining to handle collision resolution. - The __len__ (self) method that returns the total number of elements in the hash table. Note that the hash a list of linked lists. to calculate the total number of elements among all the linked lists in the hash table. Notes: - Submit the entire LinkedListHashTable class definition in the answer box below. - Node and LinkedList implementations are provided to you as part of this exercise - you should not define your own Node/LinkedList classes. You can download their definition here. For example: Answer: (penalty regime: 0,0,5,10,15,20,25,30,35,40,45,50% )
Step by Step Solution
There are 3 Steps involved in it
Step: 1
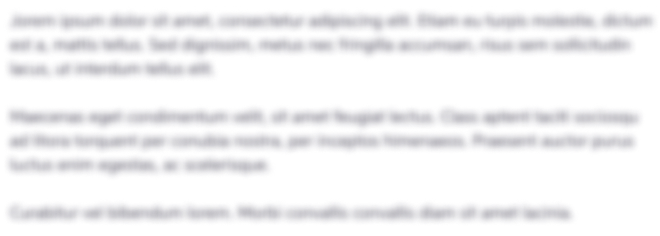
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started