Exp 1: Write a recursive function for maximum, minimum, etc.
Exp 4: Use the code provided to answer sub-questions 1 to 5. ONLY USE THE CODE PROVIDED
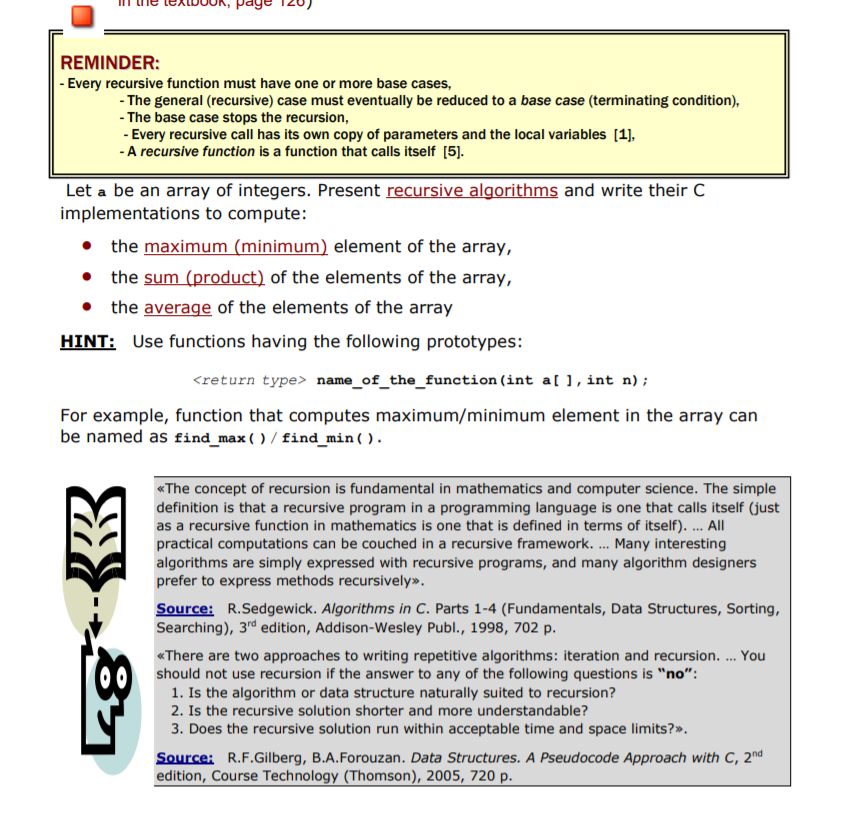
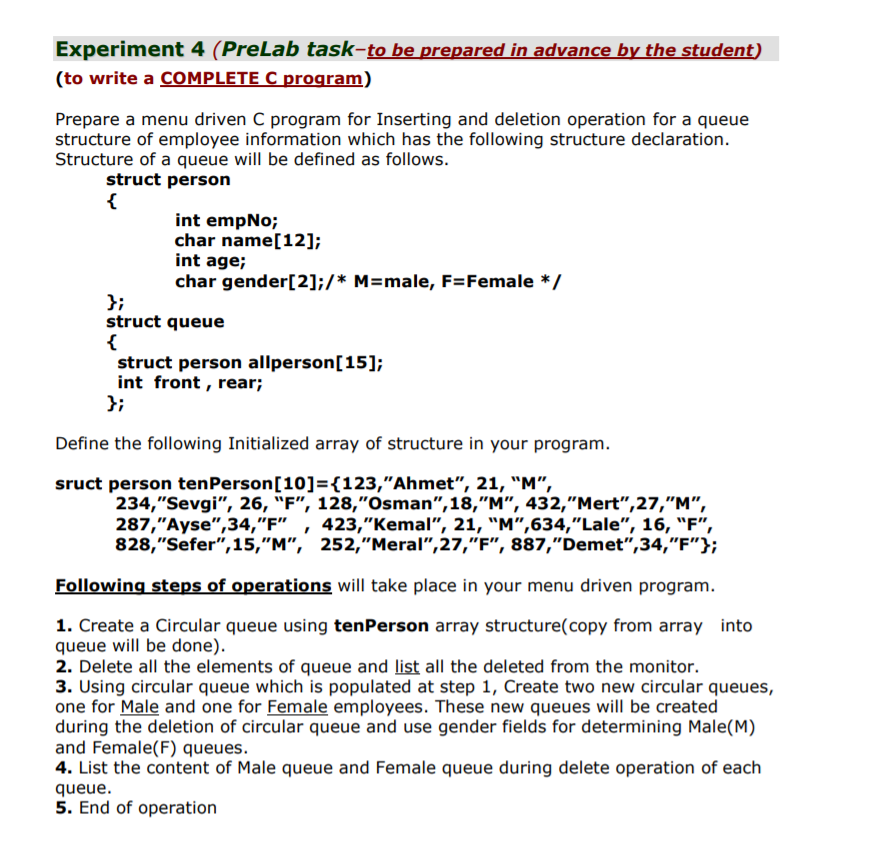
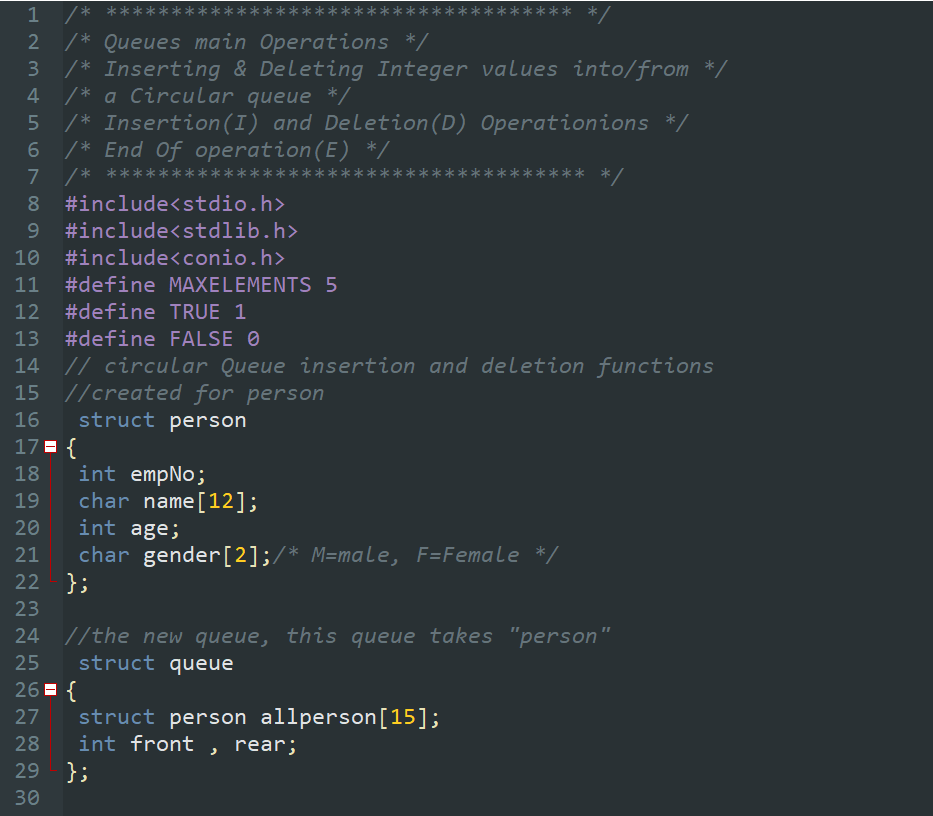
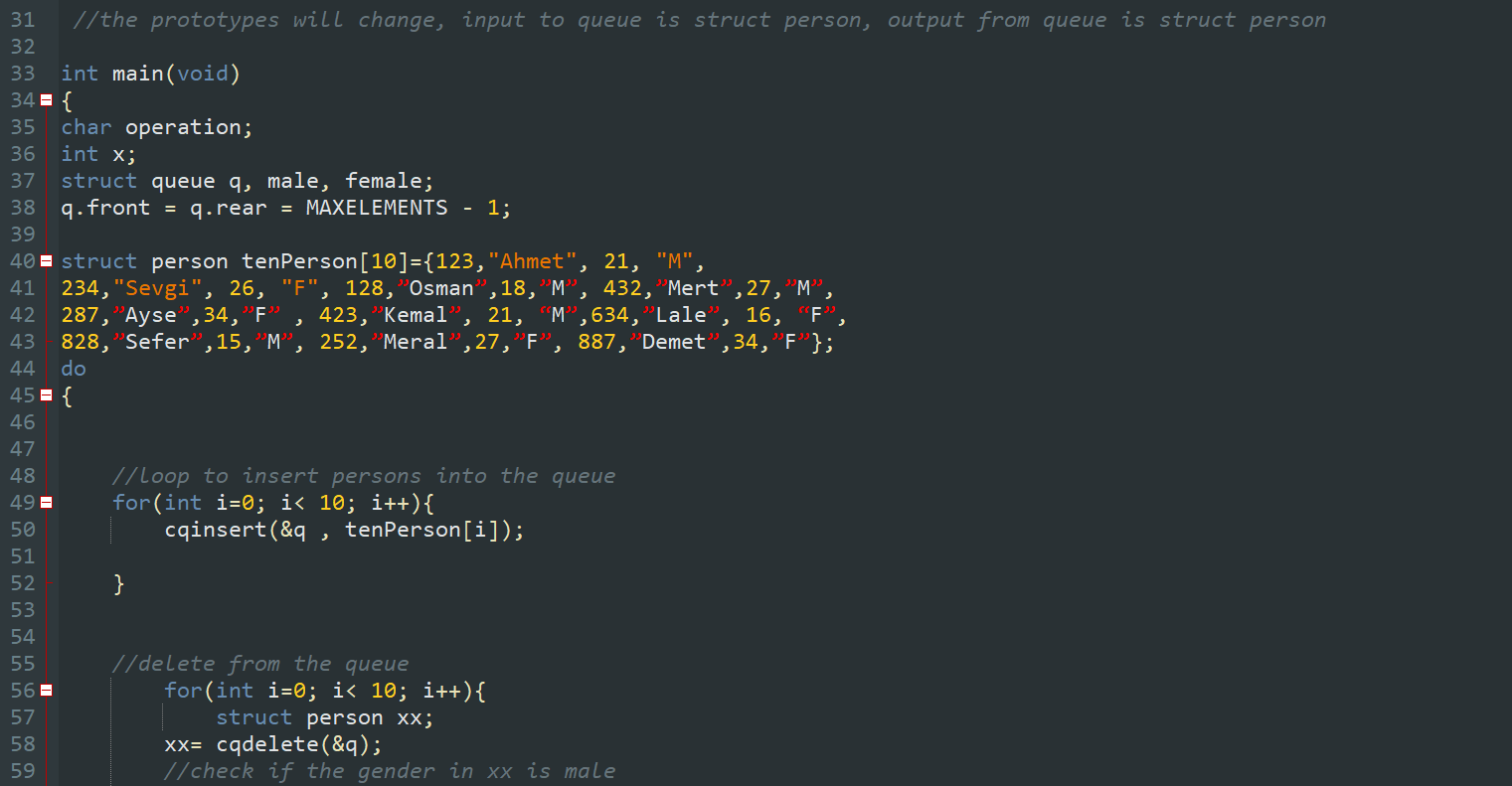
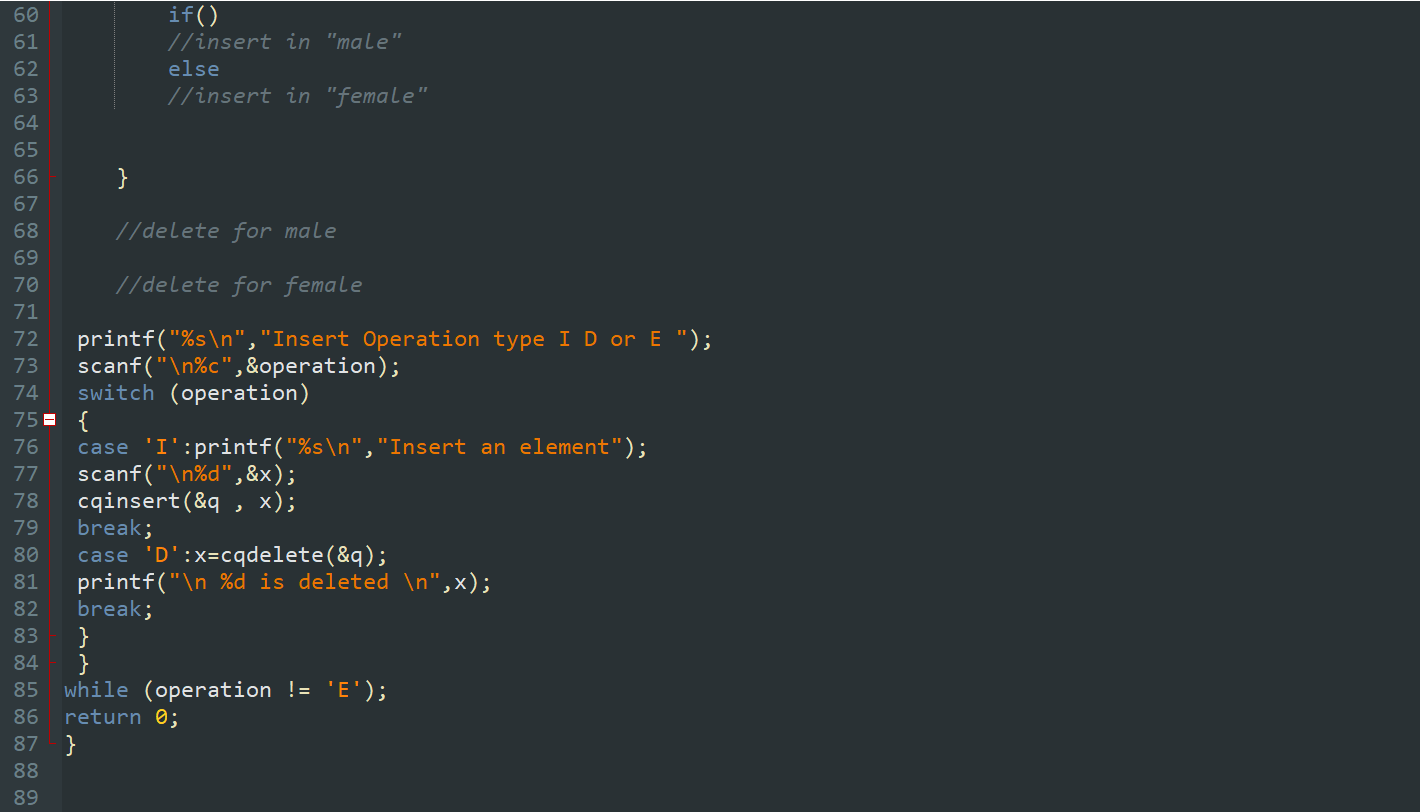
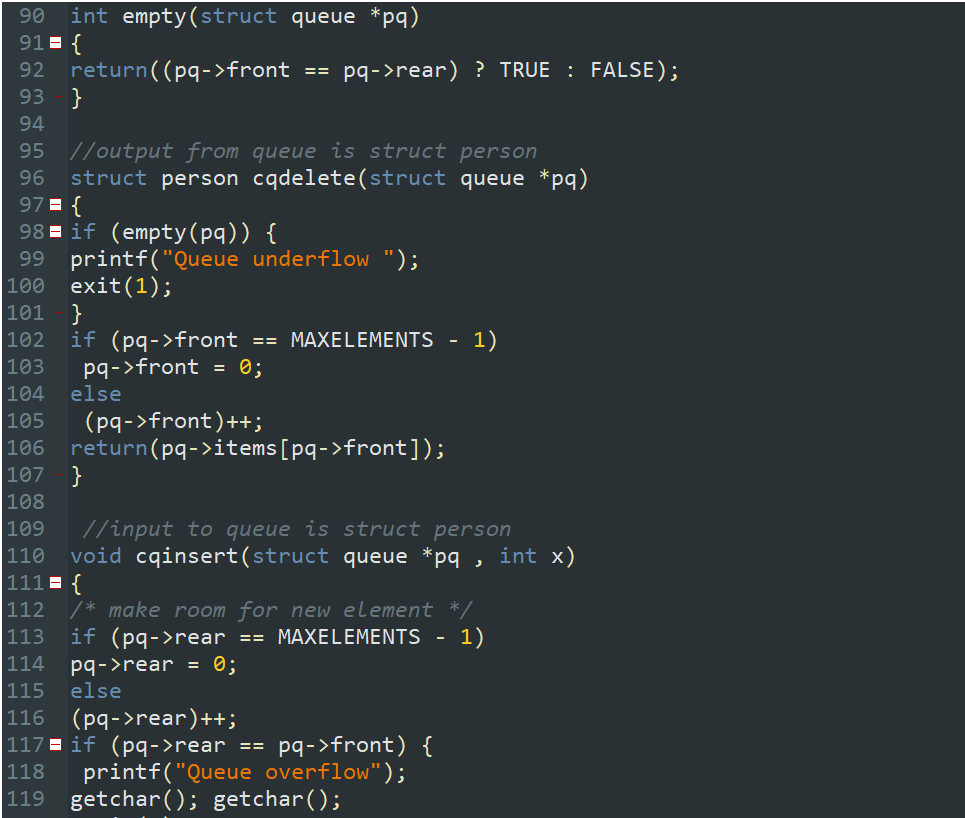
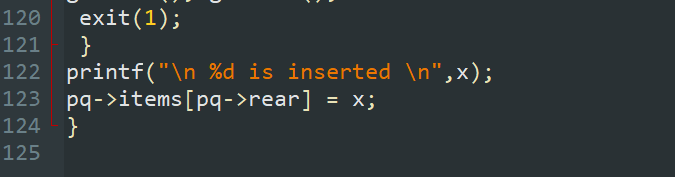
CODE IN TEXT
/* ************************************ */
/* Queues main Operations */
/* Inserting & Deleting Integer values into/from */
/* a Circular queue */
/* Insertion(I) and Deletion(D) Operationions */
/* End Of operation(E) */
/* ************************************* */
#include
#include
#include
#define MAXELEMENTS 5
#define TRUE 1
#define FALSE 0
// circular Queue insertion and deletion functions
//created for person
struct person
{
int empNo;
char name[12];
int age;
char gender[2];/* M=male, F=Female */
};
//the new queue, this queue takes "person"
struct queue
{
struct person allperson[15];
int front , rear;
};
//the prototypes will change, input to queue is struct person, output from queue is struct person
int main(void)
{
char operation;
int x;
struct queue q, male, female;
q.front = q.rear = MAXELEMENTS - 1;
struct person tenPerson[10]={123,"Ahmet", 21, "M",
234,"Sevgi", 26, "F", 128,Osman,18,M, 432,Mert,27,M,
287,Ayse,34,F , 423,Kemal, 21, M,634,Lale, 16, F,
828,Sefer,15,M, 252,Meral,27,F, 887,Demet,34,F};
do
{
//loop to insert persons into the queue
for(int i=0; i
cqinsert(&q , tenPerson[i]);
}
//delete from the queue
for(int i=0; i
struct person xx;
xx= cqdelete(&q);
//check if the gender in xx is male
if()
//insert in "male"
else
//insert in "female"
}
//delete for male
//delete for female
printf("%s ","Insert Operation type I D or E ");
scanf(" %c",&operation);
switch (operation)
{
case 'I':printf("%s ","Insert an element");
scanf(" %d",&x);
cqinsert(&q , x);
break;
case 'D':x=cqdelete(&q);
printf(" %d is deleted ",x);
break;
}
}
while (operation != 'E');
return 0;
}
int empty(struct queue *pq)
{
return((pq->front == pq->rear) ? TRUE : FALSE);
}
//output from queue is struct person
struct person cqdelete(struct queue *pq)
{
if (empty(pq)) {
printf("Queue underflow ");
exit(1);
}
if (pq->front == MAXELEMENTS - 1)
pq->front = 0;
else
(pq->front)++;
return(pq->items[pq->front]);
}
//input to queue is struct person
void cqinsert(struct queue *pq , int x)
{
/* make room for new element */
if (pq->rear == MAXELEMENTS - 1)
pq->rear = 0;
else
(pq->rear)++;
if (pq->rear == pq->front) {
printf("Queue overflow");
getchar(); getchar();
exit(1);
}
printf(" %d is inserted ",x);
pq->items[pq->rear] = x;
}
REMINDER: - Every recursive function must have one or more base cases, - The general (recursive) case must eventually be reduced to a base case (terminating condition), - The base case stops the recursion, - Every recursive call has its own copy of parameters and the local variables [1], - A recursive function is a function that calls itself [5]. Let a be an array of integers. Present recursive algorithms and write their C implementations to compute: the maximum (minimum) element of the array, the sum (product) of the elements of the array, the average of the elements of the array HINT: Use functions having the following prototypes:
name_of_the_function (int a[ ], int n); For example, function that computes maximum/minimum element in the array can be named as find_max ( ) / find_min(). The concept of recursion is fundamental in mathematics and computer science. The simple definition is that a recursive program in a programming language is one that calls itself (just as a recursive function in mathematics is one that is defined in terms of itself). ... All practical computations can be couched in a recursive framework. ... Many interesting algorithms are simply expressed with recursive programs, and many algorithm designers prefer to express methods recursively. Source: R.Sedgewick. Algorithms in C. Parts 1-4 (Fundamentals, Data Structures, Sorting, Searching), 3rd edition, Addison-Wesley Publ., 1998, 702 p. There are two approaches to writing repetitive algorithms: iteration and recursion. ... You should not use recursion if the answer to any of the following questions is "no": 1. Is the algorithm or data structure naturally suited to recursion? 2. Is the recursive solution shorter and more understandable? 3. Does the recursive solution run within acceptable time and space limits?. Source: R.F.Gilberg, B.A.Forouzan. Data Structures. A Pseudocode Approach with C, 2nd edition, Course Technology (Thomson), 2005, 720 p. Experiment 4 (PreLab task-to be prepared in advance by the student) (to write a COMPLETE C program) Prepare a menu driven C program for Inserting and deletion operation for a queue structure of employee information which has the following structure declaration. Structure of a queue will be defined as follows. struct person { int empNo; char name[12]; int age; char gender[2];/* M=male, F=Female */ }; struct queue { struct person allperson[15]; int front, rear; }; Define the following Initialized array of structure in your program. sruct person tenPerson[10]={123,"Ahmet", 21, "M", 234,"Sevgi", 26, "F", 128,"Osman", 18,"M", 432,"Mert",27,"M", 287,"Ayse", 34,"F" , 423,"Kemal", 21, "M",634,"Lale", 16, "F", 828,"Sefer",15,"M", 252,"Meral", 27,"F", 887,"Demet", 34,"F"}; Following steps of operations will take place in your menu driven program. 1. Create a Circular queue using tenPerson array structure(copy from array into queue will be done). 2. Delete all the elements of queue and list all the deleted from the monitor. 3. Using circular queue which is populated at step 1, Create two new circular queues, one for Male and one for Female employees. These new queues will be created during the deletion of circular queue and use gender fields for determining Male(M) and Female(F) queues. 4. List the content of Male queue and Female queue during delete operation of each queue. 5. End of operation 1 /* 2 /* Queues main Operations */ 3 /* Inserting & Deleting Integer values into/from */ 4. /* a Circular queue */ 5 /* Insertion(I) and Deletion(D) Operationions */ 6 /* End of operation (E) */ 7 /* *** 8 #include #include 10 #include 11 #define MAXELEMENTS 5 12 #define TRUE 1 13 #define FALSE O 14 // circular Queue insertion and deletion functions 15 //created for person 16 struct person 17 = { 18 int empNo; 19 char name[12]; 20 21 char gender[2]; /* M=male, F=Female */ 22 }; 23 24 //the new queue, this queue takes "person" 25 struct queue 26 = { 27 struct person allperson[15]; 28 int front, rear; 29 }; 30 int age; int x; > 31 //the prototypes will change, input to queue is struct person, output from queue is struct person 32 33 int main(void) 34 = { 35 char operation; 36 37 struct queue q, male, female; 38 q.front = q.rear = MAXELEMENTS - 1; 39 40 = struct person tenPerson[10]={123, "Ahmet", 21, "M". 41 234, "Sevgi", 26, "F", 128, "Osman", 18,"M", 432, "Mert", 27,'M", 42 287, "Ayse", 34, "F" 423, Kemal, 21, M,634, "Lale'', 16, F 43 828, Sefer'',15,"M", 252, Meral, 27,"F", 887, "Demet", 34, "F}; 44 do 45 = { 46 47 48 // loop to insert persons into the queue 49= for(int i=0; ifront == pq->rear) ? TRUE : FALSE); 93 } 94 = 95 //output from queue is struct person 96 struct person cqdelete(struct queue *pq) 97= { 98 = if (empty(pq)) { 99 printf("Queue underflow "); 100 exit(1); 101 } 102 if (pq->front == MAXELEMENTS - 1) 103 pq->front 0; 104 else 105 (pq->front)++; 106 return(pq->items[pq->front]); 107 108 109 //input to queue is struct person 110 void cqinsert(struct queue *pq , int x) 111 = { 112 /* make room for new element */ 113 if (pq->rear == MAXELEMENTS - 1) 114 pq->rear = 0; 115 else 116 (pq->rear)++; 117 = if (pq->rear pq->front) { printf("Queue overflow"); 119 getchar(); getchar(); == 118 120 exit(1); 121 } 122 printf(" %d is inserted ",x); 123 pq->items [pq->rear] = x; 124 } 125