Question
Extend the msh code from below: ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ #include #include int main(){ char str[1000]; printf(msh> ); fgets(str, 120, stdin); while(strcmp(str, exit )!=0) { if(feof(stdin)){ break; }
Extend the msh code from below:
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
#include
#include
int main(){
char str[1000];
printf("msh> ");
fgets(str, 120, stdin);
while(strcmp(str, "exit ")!=0) {
if(feof(stdin)){
break;
}
int len = strlen(str);
if(len>120){
printf("msh> error: input too long ");
}else if(len>1){
printf("%s", str);
}
printf("msh> ");
fgets(str, 1000, stdin);
}
return 0;
}
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
- as before, exit msh when exit or ctrl-d is entered
- run Linux commands that are entered by the user. You must do this by using fork and exec. After a command is executed, the prompt should be displayed as usual.
I recommend that you implement this feature by first breaking the user input into tokens. The tokens are the strings in the input line separated by whitespace (spaces or tabs). The tokens should not include any whitespace.
For example, on input
msh> wc -l
there are two tokens: wc and -l.
Hint: use C library function strtok to break the user input into tokens.
- Add a help command that will simply display enter Linux commands, or exit to exit
- Add a today command that will print the current date in the format mm/dd/yyyy, where mm is month, dd is day of month, and yyyy is year. For example, 01/02/2018 is January 2, 2018. Hint: when I implemented this, I used Linux commands time and localtime. Try man 2 time, and man localtime. Its a little tricky.
Here is some sample output to help you understand what your program should do:
$ msh
msh> ls
temp.txt README.txt
msh> help
enter Linux commands, or exit to exit
msh> foo 1
msh: foo: No such file or directory
msh> today
01/02/2018
msh> exit
$
Note the error message reported when foo was entered. execvp() will return an error message if it couldnt run the command entered by the user. How do you know this? execvp() will only return if there was a problem running the command that was passed to it. Here is the code I use to print an error message if execvp returns:
printf("msh: %s: %s ", toks[0], strerror(errno));
Here toks is an array I used to store the tokens entered at the command line. strerror and errno are defined by Linux. For more information, look for errno in the man page for exec, and look at the man page for strerror.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
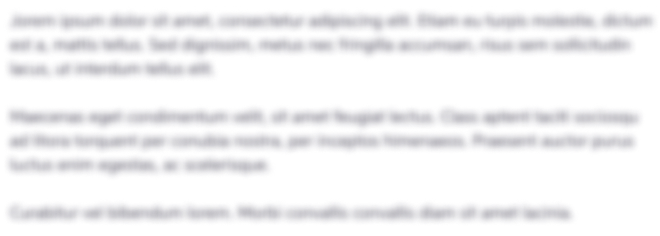
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started