Question
F# Programming Assignment: Guidelines for All Programming Assignments: Create your programs using Visual Studio Scripting; All of your F# code should be purely functional. You
F# Programming Assignment:
Guidelines for All Programming Assignments:
Create your programs using Visual Studio Scripting;
All of your F# code should be purely functional. You should not make any use of mutable variables, loops, etc.;
Do not use built-in F# functions other than the ones discussed in class;
Pay attention to the types that F# infers for your functions! They are very informative;
Put comments in your code as appropriate;
You may define auxiliary functions to help in solving some of the problems, but you should try to use them only when necessary. You must provide a clear specification of each auxiliary function that you define.
Problem 1: Write an F# function pair(xs,ys) that pairs the elements in two lists into a new list:
> pair ['a';'b';'c'] [1;2;3];;
val it : (char * int) list = [('a', 1); ('b', 2); ('c', 3)]
Problem 2: Write a curried F# function cartesian xs ys that takes as input two lists xs and ys and returns a list of pairs that represents the Cartesian product of xs and ys. (The pairs in the Cartesian product may appear in any order.) For example,
> cartesian ['a';'b';'c'] [1;2;3];; val it : (char * int) list =
[('a', 1); ('a', 2); ('a', 3); ('b', 1); ('b', 2); ('b', 3); ('c', 1); ('c', 2); ('c', 3)]
Problem 3: An F# list can be thought of as representing a set, where the order of the elements in the list is irrelevant. Write an F# function powerset such that powerset set returns the set of all subsets of set. For example,
> powerset [1;2;3];;
val it : int list list =
[[]; [3]; [2]; [2; 3]; [1]; [1; 3]; [1; 2]; [1; 2; 3]]
Problem 4: Write an F# program split(x) that splits list x into two lists y and z such that y contains the even numbered elements of x and z contains the odd numbered element of x. For example,
> split [1;2;3;4;5;6;7];;
val it : int list * int list = ([1; 3; 5; 7], [2; 4; 6])
Problem 5: The transpose of a matrix M is the matrix obtained by reflecting M about its diagonal. For example, the transpose of is
An m-by-n matrix can be represented in F# as a list of mrows, each of which is a list of length n. For example, the first matrix above is represented as the list [[1;4]; [2;5]; [3;6]]. Write an efficient F# function to compute the transpose of an m-by-n matrix:
> transpose [[1;4];[2;5];[3;6]];;
val it : int list list = [[1; 2; 3]; [4; 5; 6]]
456 123Step by Step Solution
There are 3 Steps involved in it
Step: 1
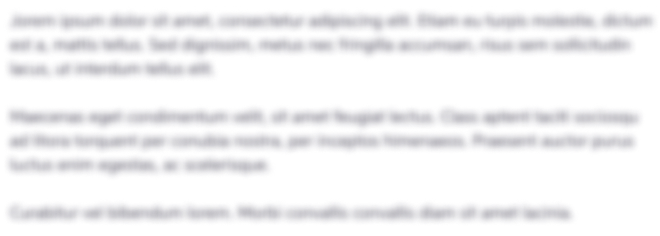
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started