Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Falling Sand Project: Part 1 - Run the Program Run the program in NetBeans. You should see a window pop up . On the left
Falling Sand Project: Part Run the Program
Run the program in NetBeans. You should see a window pop up On the left side is a black rectangular canvas which will soon be inhabited by particles. On the right side there is one button for each tool you will be able to paint with: Empty for erasing and Metal for creating metal particles You can't actually paint now, because you haven't written the code yet.
Look in the SandLab.java file, and you'll see that a SandLab remembers two things:
grid a dimensional array of int values that represent the type of particle found at each location
display the SandDisplay used to show the particles on the screen
Notice that we're using int values to represent particle types, with representing empty, representing metal, and higher values representing the additional particle types you'll be adding. To avoid confusion, we never want to see these particle type numbers etc. in our code! Instead, we've declared variables for each of these types. You'll see these listed near the top of SandLab.java.
public static final int EMPTY ;
public static final int METAL ;
This lets us use meaningful variable names instead of confusing type numbers in our code. For example:
if type METAL
These variables are marked final to indicate that they are constants. Attempts to reassign to these variables will not compile. By convention in Java, we use allcaps names for constants. Traditionally constants are also declared as public and static, so that we can access them from outside the file by writing SandLab.METAL, for example.
Part Constructor
The SandLab constructor lines already initializes the display field to refer to a new SandDisplay with appropriate dimensions and tool names. Insert code to initialize the grid field to refer to a dimensional array of the same dimensions. The rows are the first dimension of the array, and the columns are the second dimension. You won't be able to test this code yet.
Part locationClicked Method
The locationClicked method is called by the run method whenever the user clicks on some part of the canvas. The selected tool empty metal, etc. is passed to the method. Store this value in the corresponding position of the grid array. You won't be able to test this code yet.
package fallingsand;
import java.awt.;
public class SandLab
add constants for particle types here
public static final int EMPTY ;
public static final int METAL ;
do not add any more fields
private int grid;
private SandDisplay display;
public static final int ROWS ;
public static final int COLUMNS ;
public static void mainString args
SandLab lab new SandLabROWS COLUMNS;
lab.run;
public SandLabint numRows, int numCols
String names;
names new String;
namesEMPTY "Empty";
namesMETAL "Metal";
display new SandDisplayFalling Sand", numRows, numCols, names;
called when the user clicks on a location using the given tool
private void locationClickedint row, int col, int tool
copies each element of grid into the display
public void updateDisplay
Color empty new Color;
for int row ; row ROWS; row
for int column ; column COLUMNS; column
if gridrowcolumn EMPTY
display.setColorrow column, empty;
called repeatedly.
causes one random particle to maybe do something.
public void step
do not modify
public void run
while true
for int i ; i display.getSpeed; i
step;
updateDisplay;
display.repaint;
display.pause; wait for redrawing and for mouse
int mouseLoc display.getMouseLocation;
if mouseLoc nulltest if mouse clicked
locationClickedmouseLoc mouseLoc display.getTool;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
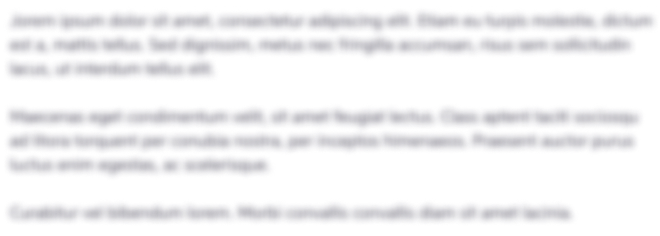
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started