Question
File Server Problem In this problem we will take our solution to JH2 called FileOperations and we will make it work across a network. From
File Server Problem
In this problem we will take our solution to JH2 called FileOperations and we will make it work across a network. From this we will have in effect the beginnings of a minimal File Server. You can start from your solution or you can start from the published answer to JH2.
I would recommend that you use 2 work spaces and 2 instances of Eclipse to develop this .... one for the Client side and one for the FileServer side.
Note that all of our FileOperations commands were a one line command. However, the output for a given command could be many lines. To make this work, we need invent the following protocol:
- Our FileClient software will send one command line to the File Server.
- The File Server will take the command and send back as many lines as it wishes.
- When a command is done, our File Server will send back an empty line which is effectively a call to: somePrintStream.println( );
Client Code
- Include EchoClient.java in your project.
- Create a class called FileClient which extends EchoClient. DO NOT MODIFY EchoClient.java. All of your work can be done in FileClient.
- Override the "communicate" method. A lot of the code will stay the same. The main thing that happens is that you will keep reading from the Server socket until you get a String that has zero length.
- When you print out the output received from your Server, I would like you to start each line with "received from server:" So for example the output from an list command might be:
received from server: list . received from server: Listing files for C:\Users\chasselb.000\Desktop\Archives\ClassWork\workspace_java\JH5\. received from server: .classpath received from server: .project received from server: .settings received from server: bin received from server: cmd.txt received from server: src
Server Code
- To start out, include the following in your project: MotherServer.java, EchoServer.java.
- Take your solution to FileOperations (or use the JH2 published answer) and rename it to FileServer.
- FileServer should extend EchoServer. Do all of your work in this class and DO NOT MODIFY ConnectListener.java, MotherServer.java, or EchoServer.java.
- Create a PrintStream instance variable in FileServer. I called mine outputPs.
- Every place where your old FileOperations did a System.out.println(.....), my code was changed to: outputPS.println(......)
- After these changes, you shouldn't need to change most of your methods: getFile, getNextToken, printUsage, rename, delete, list, etc.
- Your processCommandLine will be the same as for JH2, except that you will want send out a blank line just before it returns. THIS IS VERY IMPORTANT or else your client won't know when a command has finished.
- You will not use the main in FileOperations, nor the processCommandFile methods from your previous JH2 solution.
- Instead, you will override the processStream method in EchoServer. Your main will be similar to the main that you see in EchoServer (minor changes).
- It is useful to have the Server code print out to the screen each command that it is processes. This is useful for debug purposes.
Summary of the above Server changes:
public class FileServer extends EchoServer { StringTokenizer parseCommand; PrintStream outputPs=null; // Other than changing the calls System.out.println(....) to outputPs.println(....), the following routines should need no work: public void delete()..... public void rename()..... public void list()..... public void size()..... public void lastModified()..... public void mkdir()..... public void createFile()..... public void printFile()..... void printUsage()..... private String getNextToken()..... public File getFile()..... public boolean processCommandLine(String line)..... // Additionally the processCommandLine routine should do 2 things: 1.) echo the command to be processed to the Screen 2.) Send a blank line to outputPs just before this routine returns You can eliminate the processCommandFile and main methods and replace them with: 1.) Overriding processStream from EchoServer 2.) A main that is similar to the main in EchoServer Your processStream method should do the following: void processStream(InputStream is, OutputStream os) { Create a Scanner from is, and a PrintStream from os. Set outputPs (instance variable) equal to the PrintStream Read lines as long as the hasNextLine method of the Scanner class returns true. Send the String read to processCommandLine and check the return code. If processCommandLine returns false we need to exit. Make sure you always close your Scanner and PrintStream classes. } public static void main(String[] args) { FileServer fs = new FileServer(); fs.monitorServer(); System.out.println("Exitting FileServer"); } }
My Soultion to JH2 File Operations
import java.io.*; import java.util.*; public class FileOperations { StringTokenizer parseCommand; String fileForAction = ""; File path; public void delete() { boolean returnCode = false; if (path.exists()) { System.out.println("Deleting: " + path.getAbsolutePath()); returnCode = path.delete(); } else { System.out.println("File does not exist: " + path); } if (returnCode == true) { System.out.println("Successful Deletion"); } else { System.out.println("Failed to Delete."); } printSeperator(); // code for handling the delete command // Make sure you check the return code from the // File delete method to print out success/failure status } public void rename(ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
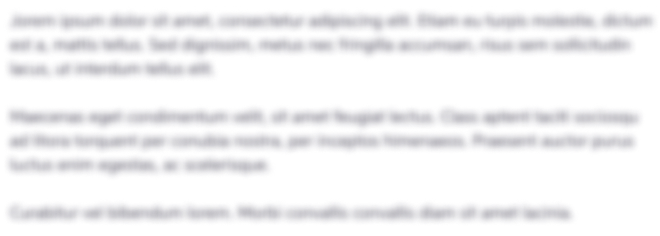
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started