Question
Find AND correct the six errors in the following pseudo code program. Circle the bad code and write the correct code next to your circle.
Find AND correct the six errors in the following pseudo code program. Circle the bad code and write the correct code next to your circle. Problem Statement: The program will read from an input file called students.txt, and write to an output file called summary.txt. The InputFile, students.txt, includes multiple records. Each record includes the following fields: age degreeType (1 = Information Technology, 2 = Computer Science) collegeYears vaResident (1 = yes, 2 = no, 0 = end of file) The OutputFile, summary.txt, will include summary information that is written by the program. The program will write the following fields for each output record: the total number of people with exactly 1 year of college the total number of people with exactly 2 years of college the average age of all people with exactly 1 year of college the average age of all people with exactly 2 years of college the total number of people in the Computer Science program You should: 1 2 Circle ONLY statements that contain LOGIC errors. Next to the circled statement, provide the correct LOGIC. Note: There is no need to validate the input file. You can assume that the data is valid. Pseudo Code: // variables for the fields in the input file Integer age = 0 Integer degreeType = 0 Integer collegeYears = 0 Integer vaResident = 0 // other variables Integer countOneYear = 0 Integer countTwoYear = 0 Integer sumAgeOneYear = 0 Integer sumAgeTwoYear = 0 Real avgAgeOneYear = 0.0 Real avgAgeTwoYear = 0.0 Integer countCompSci= 0 // open the input file Declare InputFile studentFile Open studentFile students.txt // lead read of the input file Read studentFile age, degreeType, collegeYears, vaResident while (vaResident != 0) if (degreeType == 1) countCompSci = countCompSci + 1 endif if (collegeYears == 1) countOneYear = countOneYear + 2 sumAgeOneYear = sumAgeOneYear + age else if (collegeYears == 3) countTwoYear = countTwoYear + 1 sumAgeTwoYear = sumAgeTwoYear + 1 endif Read studentFile age, degreeType, collegeYears, vaResident endWhile avgAgeOneYear = sumAgeOneYear / countOneYear avgAgeTwoYear = sumAgeTwoYear / 2 // open the output file Declare OutputFile summaryFile Open summaryFile summary.txt // write to the output file write summaryFile collegeYears, countTwoYear, avgAgeOneYear, avgAgeTwoYear, countCompSci
Step by Step Solution
There are 3 Steps involved in it
Step: 1
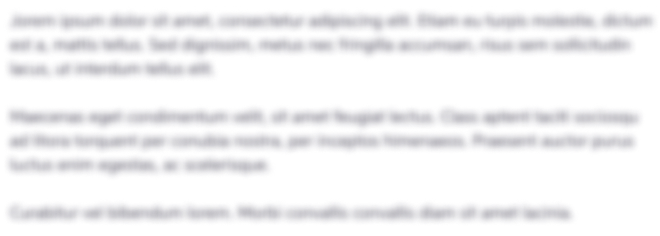
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started