Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Finding Mesas To be clear, all programming assignments in this class are individual assignments. In this assignment, you are tasked with using structs and dynamic
Finding Mesas
To be clear, all programming assignments in this class are individual assignments.
In this assignment, you are tasked with using structs and dynamic memory to find Mesas within a set of integers.
This spec is long, and we recognize that. However, we attempted to answer a lot of questions that may come up within the spec. Please try to find the answers to your questions in here before asking.
What is a Mesa?
A mesa is defined in the following way:
Given a set of nonnegative integers, a mesa is a sequence of at least a given minimum identical values. The length of a mesa is the number of values in the sequence, and the mass of a mesa is the sum of the values in the sequence.
The first number in the dataset is the number of values to follow on that line. In the example below, the first number is so there are values following. The numbers highlighted in blue are on their own line. They represent the minimum length and minimum height of a valid Mesa in this set of data. In other words, there must be at least of the same number in a row and that number must be at least for a Mesa to be considered valid in this set of data.
Given this data above, there are two valid mesas. The first mesa highlighted in yellow is the values of which have a mass of and the other highlighted in purple is the values of which have a mass of In this set of numbers the first mesa is the bigger of the two because it has a higher total mass, so that is the one we are asking you to find in this assignment.
Implementation
Download the starter code for the assignment:
array.cDownload array.c
array.hDownload array.h
mesa.hDownload mesa.h
main.cDownload main.c
Your implementation will be broken down into files: main.c array.c and mesa.c You will have corresponding header files array.h and mesa.h for the declarations in those C files.
array.c
We strongly encourage you to test that your array.c is working correctly and producing a clean Valgrind report before moving on The more code you have, the harder it is to debug.
The array.c file will be responsible for handling operations related to the array input, including the management of the dynamic memory and the display of the formatted array in the output file.
The below declarations are all in the array.h file. You will be implementing of them. readdata is implemented for you.
unsigned int createarray FILE in unsigned int size ;
unsigned int freememory unsigned int pointer ;
void displaydata FILE out, unsigned int count, unsigned int data ;
void readdata FILE in unsigned int data unsigned int count ;
When you submit your code, we will provide a file with this name with the appropriate declarations as shown as well as #includes for stdio.h and stdlib.h If you need other libraries include, you will need to put those in your implementation file. Hint: You shouldnt need any other header files.
unsigned int createarray FILE in unsigned int size ;
FILE in is an already opened pointer to the input file assuming the input file was opened correctly and passed correctly from main
unsigned int size will store the number of elements remaining in the line that is read from the file once the function is over
The function returns a pointer to the dynamically allocated array of the given number of elements.
This function will read an unsigned integer from the file that is targeted by the FILE named in It will then dynamically allocate an array that is capable of storing the number of integers that was indicated by the number that was read. The array will not store fewer nor more integers than the number that was read indicates. Make an array that will store exactly that number of integers.
The function will return the pointer to the array that was dynamically allocated and the size parameter will store the number that was read in
For example, if this is the line:
The array that is created will store integers and the size parameter will store the value
unsigned int freememory unsigned int pointer ;
unsigned int pointer is a pointer to a previously dynamically allocated array of integers.
The function returns NULL so that the pointer can be reset in the calling function the pointer itself will not change permanently in this function other than releasing the memory
This function is given a pointer to a previously dynamically allocated array and releases the targeted memory, then returns NULL.
void displaydata FILE out, unsigned int count, unsigned int data ;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
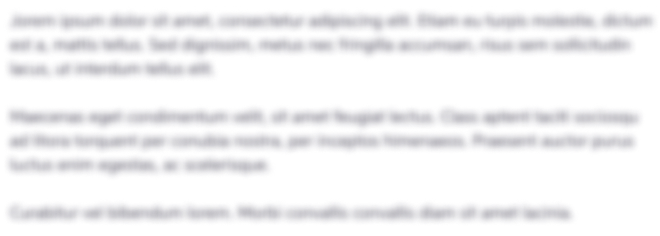
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started