Question
First, launch NetBeans and close any previous projects that may be open (at the top menu go to File ==> Close All Projects). Then create
First, launch NetBeans and close any previous projects that may be open (at the top menu go to File ==> Close All Projects).
Then create new Java application called "Parser" (without the quotation marks)that uses methods to:
1.Get a comma-delimited String of integers (e.g. "4, 8, 16, 32") from the user at the command line and then convert the String to an ArrayList of integers (using the wrapper class) with each element containing one of the input integers in sequence
2.Print the integers to the command line, using a for loop, so that each integer is on a separate line
One of the PA requirements is to use an ArrayList where each element is an integer.There are several
implementation approaches. One approach is to declare a local ArrayList in main. The use of two
methods is required.
Method 1: Declare a local string variable. Prompt the user for a list of integers separated by commas.
Read this into the String variable using the nextLine() method (because there will be blank spaces
between the integer values). There are several approaches for extracting the numeric values from the
string. We have done this before.
One approach is to pre-process the string replacing the commas with blank spaces and then using a
while loop with hasNextInt() as the loop condition.
Look up the replaceAll method for replacing the commas.
Using this approach, the integer extraction loop might look similar to this. Notice the storing of the
integer values into ArrayList intArray using the add method.
Scanner listOfNums = new Scanner(strOfNums);
// prompt for string of integers separated by commas
// read in string using nextLine() method
// replace ',' with blank spaces using replaceAll method
// set up local scanner on the string - in this case called listOfNums
while (listOfNums.hasNextInt()) {
curInt = listOfNums.nextInt();
intArray.add(i,curInt);
i++;
}
The header for Method 1 might look similar to this where intArray is a locally declared ArrayList in main.
public static void inputProcess(ArrayList intArray)
Method 2 prints out the integer values from the elements in the ArrayList using the get method.
The method header might look similar to this:
public static void outputProcess(ArrayList intArray)
An approach for implementing main, pseudocode:
Declare local ArrayList
Call 'inputProcess' method with ArrayList parameter
Call 'outputProcess' method with ArrayList parameter
Example run testing for extra spaces and commas, as well as, the case where the user only enters one
value:
Run 1:
Enter list of integers separated by commas:
5, 6, 45,56,67 ,0, 1,
Output:
5
6
45
56
67
0
3
1
Run 2:
Enter list of integers separated by commas:
6
Output:
6
Step by Step Solution
There are 3 Steps involved in it
Step: 1
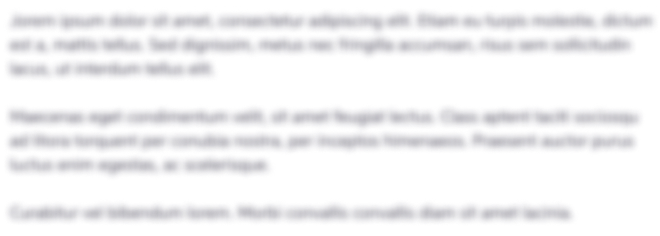
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started