Question
Aircraft.java public class Aircraft implements Comparable { int numEconomySeats; int numFirstClassSeats; String model; public Aircraft(int seats, String model) { this.numEconomySeats = seats; this.numFirstClassSeats = 0;
Aircraft.java
public class Aircraft implements Comparable
{
int numEconomySeats;
int numFirstClassSeats;
String model;
public Aircraft(int seats, String model)
{
this.numEconomySeats = seats;
this.numFirstClassSeats = 0;
this.model = model;
}
public Aircraft(int economy, int firstClass, String model)
{
this.numEconomySeats = economy;
this.numFirstClassSeats = firstClass;
this.model = model;
}
public int getNumSeats()
{
return numEconomySeats;
}
public int getTotalSeats()
{
return numEconomySeats + numFirstClassSeats;
}
public int getNumFirstClassSeats()
{
return numFirstClassSeats;
}
public String getModel()
{
return model;
}
public void setModel(String model)
{
this.model = model;
}
public void print()
{
System.out.println("Model: " + model + "t Economy Seats: " + numEconomySeats + "t First Class Seats: " + numFirstClassSeats);
}
public int compareTo(Aircraft other)
{
if (this.numEconomySeats == other.numEconomySeats)
return this.numFirstClassSeats - other.numFirstClassSeats;
return this.numEconomySeats - other.numEconomySeats;
}
}
Flight.java
import java.util.Random;
public class Flight
{
public enum Status {DELAYED, ONTIME, ARRIVED, INFLIGHT};
public static enum Type {SHORTHAUL, MEDIUMHAUL, LONGHAUL};
public static enum SeatType {ECONOMY, FIRSTCLASS, BUSINESS};
private String flightNum;
private String airline;
private String origin, dest;
private String departureTime;
private Status status;
private int flightDuration;
protected Aircraft aircraft;
protected int numPassengers;
protected Type type;
protected ArrayList manifest;
protected Random random = new Random();
private String errorMessage = "";
public String getErrorMessage()
{
return errorMessage;
}
public void setErrorMessage(String errorMessage)
{
this.errorMessage = errorMessage;
}
public Flight()
{
this.flightNum = "";
this.airline = "";
this.dest = "";
this.origin = "Toronto";
this.departureTime = "";
this.flightDuration = 0;
this.aircraft = null;
numPassengers = 0;
status = Status.ONTIME;
type = Type.MEDIUMHAUL;
manifest = new ArrayList();
}
public Flight(String flightNum)
{
this.flightNum = flightNum;
}
public Flight(String flightNum, String airline, String dest, String departure, int flightDuration, Aircraft aircraft)
{
this.flightNum = flightNum;
this.airline = airline;
this.dest = dest;
this.origin = "Toronto";
this.departureTime = departure;
this.flightDuration = flightDuration;
this.aircraft = aircraft;
numPassengers = 0;
status = Status.ONTIME;
type = Type.MEDIUMHAUL;
manifest = new ArrayList();
}
public Type getFlightType()
{
return type;
}
public String getFlightNum()
{
return flightNum;
}
public void setFlightNum(String flightNum)
{
this.flightNum = flightNum;
}
public String getAirline()
{
return airline;
}
public void setAirline(String airline)
{
this.airline = airline;
}
public String getOrigin()
{
return origin;
}
public void setOrigin(String origin)
{
this.origin = origin;
}
public String getDest()
{
return dest;
}
public void setDest(String dest)
{
this.dest = dest;
}
public String getDepartureTime()
{
return departureTime;
}
public void setDepartureTime(String departureTime)
{
this.departureTime = departureTime;
}
public Status getStatus()
{
return status;
}
public void setStatus(Status status)
{
this.status = status;
}
public int getFlightDuration()
{
return flightDuration;
}
public void setFlightDuration(int dur)
{
this.flightDuration = dur;
}
public int getNumPassengers()
{
return numPassengers;
}
public void setNumPassengers(int numPassengers)
{
this.numPassengers = numPassengers;
}
public void assignSeat(Passenger p)
{
int seat = random.nextInt(aircraft.numEconomySeats);
p.setSeat("ECO"+ seat);
}
public String getLastAssignedSeat()
{
if (!manifest.isEmpty())
return manifest.get(manifest.size()-1).getSeat();
return "";
}
public boolean seatsAvailable(String seatType)
{
if (!seatType.equalsIgnoreCase("ECO")) return false;
return numPassengers < aircraft.numEconomySeats;
}
public boolean cancelSeat(String name, String passport, String seatType)
{
if (!seatType.equalsIgnoreCase("ECO"))
{
errorMessage = "Flight " + flightNum + " Invalid Seat Type " + seatType;
return false;
}
Passenger p = new Passenger(name, passport);
if (manifest.indexOf(p) == -1)
{
errorMessage = "Passenger " + name + " " + passport + " Not Found";
return false;
}
manifest.remove(p);
if (numPassengers > 0) numPassengers--;
return true;
}
public boolean reserveSeat(String name, String passport, String seatType)
{
if (numPassengers >= aircraft.getNumSeats())
{
errorMessage = "Flight " + flightNum + " Full";
return false;
}
if (!seatType.equalsIgnoreCase("ECO"))
{
errorMessage = "Flight " + flightNum + " Invalid Seat Type Request";
return false;
}
// Check for duplicate passenger
Passenger p = new Passenger(name, passport, "", seatType);
if (manifest.indexOf(p) >= 0)
{
errorMessage = "Duplicate Passenger " + p.getName() + " " + p.getPassport();
return false;
}
assignSeat(p);
manifest.add(p);
numPassengers++;
return true;
}
public boolean equals(Object other)
{
Flight otherFlight = (Flight) other;
return this.flightNum.equals(otherFlight.flightNum);
}
public String toString()
{
return airline + "t Flight: " + flightNum + "t Dest: " + dest + "t Departing: " + departureTime + "t Duration: " + flightDuration + "t Status: " + status;
}
}
FlightManager.java
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.Random;
import java.util.Scanner;
public class FlightManager
{
ArrayList flights = new ArrayList();
String[] cities = {"Dallas", "New York", "London", "Paris", "Tokyo"};
final int DALLAS = 0; final int NEWYORK = 1; final int LONDON = 2; final int PARIS = 3; final int TOKYO = 4;
int[] flightTimes = { 3, // Dallas
1, // New York
7, // London
8, // Paris
16,// Tokyo
};
ArrayList airplanes = new ArrayList();
ArrayList flightNumbers = new ArrayList();
String errMsg = null;
Random random = new Random();
public FlightManager()
{
// Create some aircraft types with max seat capacities
airplanes.add(new Aircraft(85, "Boeing 737"));
airplanes.add(new Aircraft(180,"Airbus 320"));
airplanes.add(new Aircraft(37, "Dash-8 100"));
airplanes.add(new Aircraft(4, "Bombardier 5000"));
airplanes.add(new Aircraft(592, 14, "Boeing 747"));
// Populate the list of flights with some random test flights
String flightNum = generateFlightNumber("United Airlines");
Flight flight = new Flight(flightNum, "United Airlines", "Dallas", "1400", flightTimes[DALLAS], airplanes.get(0));
flights.add(flight);
flightNum = generateFlightNumber("Air Canada");
flight = new Flight(flightNum, "Air Canada", "London", "2300", flightTimes[LONDON], airplanes.get(1));
flights.add(flight);
flightNum = generateFlightNumber("Air Canada");
flight = new Flight(flightNum, "Air Canada", "Paris", "2200", flightTimes[PARIS], airplanes.get(1));
flights.add(flight);
flightNum = generateFlightNumber("Porter Airlines");
flight = new Flight(flightNum, "Porter Airlines", "New York", "1200", flightTimes[NEWYORK], airplanes.get(2));
flights.add(flight);
flightNum = generateFlightNumber("United Airlines");
flight = new Flight(flightNum, "United Airlines", "New York", "0900", flightTimes[NEWYORK], airplanes.get(3));
flights.add(flight);
flightNum = generateFlightNumber("Air Canada");
flight = new Flight(flightNum, "Air Canada", "New York", "0600", flightTimes[NEWYORK], airplanes.get(2));
flights.add(flight);
flightNum = generateFlightNumber("United Airlines");
flight = new Flight(flightNum, "United Airlines", "Paris", "2330", flightTimes[PARIS], airplanes.get(0));
flights.add(flight);
flightNum = generateFlightNumber("Air Canada");
flight = new LongHaulFlight(flightNum, "Air Canada", "Tokyo", "2200", flightTimes[TOKYO], airplanes.get(4));
flights.add(flight);
}
private String generateFlightNumber(String airline)
{
String word1, word2;
Scanner scanner = new Scanner(airline);
word1 = scanner.next();
word2 = scanner.next();
String letter1 = word1.substring(0, 1);
String letter2 = word2.substring(0, 1);
letter1.toUpperCase(); letter2.toUpperCase();
// Generate random number between 101 and 300
boolean duplicate = false;
int flight = random.nextInt(200) + 101;
String flightNum = letter1 + letter2 + flight;
return flightNum;
}
public String getErrorMessage()
{
return errMsg;
}
public void printAllFlights()
{
for (Flight f : flights)
System.out.println(f);
}
public boolean seatsAvailable(String flightNum, String seatType)
{
int index = flights.indexOf(new Flight(flightNum));
if (index == -1)
{
errMsg = "Flight " + flightNum + " Not Found";
return false;
}
return flights.get(index).seatsAvailable(seatType);
}
public Reservation reserveSeatOnFlight(String flightNum, String name, String passport, String seatType)
{
int index = flights.indexOf(new Flight(flightNum));
if (index == -1)
{
errMsg = "Flight " + flightNum + " Not Found";
return null;
}
Flight flight = flights.get(index);
if (!flight.reserveSeat(name, passport, seatType))
{
errMsg = flight.getErrorMessage();
return null;
}
String seat = flight.getLastAssignedSeat();
return new Reservation(flightNum, flight.toString(), name, passport, seat, seatType);
}
public boolean cancelReservation(Reservation res)
{
int index = flights.indexOf(new Flight(res.getFlightNum()));
if (index == -1)
{
errMsg = "Flight " + res.getFlightNum() + " Not Found";
return false;
}
Flight flight = flights.get(index);
flight.cancelSeat(res.name, res.passport, res.seatType);
return true;
}
public void sortByDeparture()
{
Collections.sort(flights, new DepartureTimeComparator());
}
private class DepartureTimeComparator implements Comparator
{
public int compare(Flight a, Flight b)
{
return a.getDepartureTime().compareTo(b.getDepartureTime());
}
}
public void sortByDuration()
{
Collections.sort(flights, new DurationComparator());
}
private class DurationComparator implements Comparator
{
public int compare(Flight a, Flight b)
{
return a.getFlightDuration() - b.getFlightDuration();
}
}
public void printAllAircraft()
{
for (Aircraft craft : airplanes)
craft.print();
}
public void sortAircraft()
{
Collections.sort(airplanes);
}
}
FlightReservationSystem.java
import java.util.ArrayList;
import java.util.Scanner;
// Flight System for one single day at YYZ (Print this in title) Departing flights!!
public class FlightReservationSystem
{
public static void main(String[] args)
{
FlightManager manager = new FlightManager();
ArrayList myReservations = new ArrayList(); // my flight reservations
Scanner scanner = new Scanner(System.in);
System.out.print(">");
while (scanner.hasNextLine())
{
String inputLine = scanner.nextLine();
if (inputLine == null || inputLine.equals(""))
{
System.out.print(">");
continue;
}
Scanner commandLine = new Scanner(inputLine);
String action = commandLine.next();
if (action == null || action.equals(""))
{
System.out.print(">");
continue;
}
else if (action.equals("Q") || action.equals("QUIT"))
return;
else if (action.equalsIgnoreCase("LIST"))
{
manager.printAllFlights();
}
else if (action.equalsIgnoreCase("RES"))
{
String flightNum = null;
String passengerName = "";
String passport = "";
String seatType = "";
if (commandLine.hasNext())
{
flightNum = commandLine.next();
}
if (commandLine.hasNext())
{
passengerName = commandLine.next();
}
if (commandLine.hasNext())
{
passport = commandLine.next();
}
if (commandLine.hasNext())
{
seatType = commandLine.next();
Reservation res = manager.reserveSeatOnFlight(flightNum, passengerName, passport, seatType);
if (res != null)
{
myReservations.add(res);
res.print();
}
else
{
System.out.println(manager.getErrorMessage());
}
}
}
else if (action.equalsIgnoreCase("CANCEL"))
{
Reservation res = null;
String flightNum = null;
String passengerName = "";
String passport = "";
String seatType = "";
if (commandLine.hasNext())
{
flightNum = commandLine.next();
}
if (commandLine.hasNext())
{
passengerName = commandLine.next();
}
if (commandLine.hasNext())
{
passport = commandLine.next();
int index = myReservations.indexOf(new Reservation(flightNum, passengerName, passport));
if (index >= 0)
{
manager.cancelReservation(myReservations.get(index));
myReservations.remove(index);
}
else
System.out.println("Reservation on Flight " + flightNum + " Not Found");
}
}
else if (action.equalsIgnoreCase("SEATS"))
{
String flightNum = "";
String seatType = "";
if (commandLine.hasNext())
{
flightNum = commandLine.next();
}
if (commandLine.hasNext())
{
seatType = commandLine.next();
if (manager.seatsAvailable(flightNum, seatType)) // "FCL" or "ECO"
System.out.println("Seats are available");
else
System.out.println("No " + seatType + " Seats Available");
}
}
else if (action.equalsIgnoreCase("MYRES"))
{
for (Reservation myres : myReservations)
myres.print();
}
else if (action.equalsIgnoreCase("SORTBYDEP"))
{
manager.sortByDeparture();
}
else if (action.equalsIgnoreCase("SORTBYDUR"))
{
manager.sortByDuration();
}
else if (action.equalsIgnoreCase("CRAFT"))
{
manager.printAllAircraft();
}
else if (action.equalsIgnoreCase("SORTCRAFT"))
{
manager.sortAircraft();
}
System.out.print(">");
}
}
}
LongHaulFlight.java
/*
* A Long Haul Flight is a flight that travels a long distance and has two types of seats (First Class and Economy)
*/
public class LongHaulFlight extends Flight
{
int firstClassPassengers;
public LongHaulFlight(String flightNum, String airline, String dest, String departure, int flightDuration, Aircraft aircraft)
{
super(flightNum, airline, dest, departure, flightDuration, aircraft);
type = Flight.Type.LONGHAUL;
}
public LongHaulFlight()
{
firstClassPassengers = 0;
type = Flight.Type.LONGHAUL;
}
public void assignSeat(Passenger p)
{
int seat = random.nextInt(aircraft.getNumFirstClassSeats());
p.setSeat("FCL"+ seat);
}
public boolean reserveSeat(String name, String passport, String seatType)
{
if (seatType.equalsIgnoreCase("FCL"))
{
if (firstClassPassengers >= aircraft.getNumFirstClassSeats())
{
setErrorMessage("No First Class Seats Available");
return false;
}
Passenger p = new Passenger(name, passport, "", seatType);
if (manifest.indexOf(p) >= 0)
{
setErrorMessage("Duplicate Passenger " + p.getName() + " " + p.getPassport());
return false;
}
assignSeat(p);
manifest.add(p);
firstClassPassengers++;
return true;
}
else // economy passenger
return super.reserveSeat(name, passport, seatType);
}
public boolean cancelSeat(String name, String passport, String seatType)
{
if (seatType.equalsIgnoreCase("FCL"))
{
Passenger p = new Passenger(name, passport);
if (manifest.indexOf(p) == -1)
{
setErrorMessage("Passenger " + p.getName() + " " + p.getPassport() + " Not Found");
return false;
}
manifest.remove(p);
if (firstClassPassengers > 0) firstClassPassengers--;
return true;
}
else
return super.cancelSeat(name, passport, seatType);
}
public int getPassengerCount()
{
return getNumPassengers() + firstClassPassengers;
}
public boolean seatsAvailable(String seatType)
{
if (seatType.equals("FCL"))
return firstClassPassengers < aircraft.getNumFirstClassSeats();
else
return super.seatsAvailable(seatType);
}
public String toString()
{
return super.toString() + "t LongHaul";
}
}
Passenger.java
public class Passenger
{
private String name;
private String passport;
private String seat;
private String seatType;
public Passenger(String name, String passport, String seat, String seatType)
{
this.name = name;
this.passport = passport;
this.seat = seat;
this.seatType = seatType;
}
public Passenger(String name, String passport)
{
this.name = name;
this.passport = passport;
}
public boolean equals(Object other)
{
Passenger otherP = (Passenger) other;
return name.equals(otherP.name) && passport.equals(otherP.passport);
}
public String getSeatType()
{
return seatType;
}
public void setSeatType(String seatType)
{
this.seatType = seatType;
}
public String getName()
{
return name;
}
public void setName(String name)
{
this.name = name;
}
public String getPassport()
{
return passport;
}
public void setPassport(String passport)
{
this.passport = passport;
}
public String getSeat()
{
return seat;
}
public void setSeat(String seat)
{
this.seat = seat;
}
public String toString()
{
return name + " " + passport + " " + seat;
}
}
Reservation.java
public class Reservation
{
String flightNum;
String flightInfo;
String name;
String passport;
String seat;
String seatType;
public Reservation(String flightNum, String info)
{
this.flightNum = flightNum;
this.flightInfo = info;
}
public Reservation(String flightNum, String name, String passport)
{
this.flightNum = flightNum;
this.name = name;
this.passport = passport;
}
public Reservation(String flightNum, String info, String name, String passport, String seat, String seatType)
{
this.flightNum = flightNum;
this.flightInfo = info;
this.name = name;
this.passport = passport;
this.seat = seat;
this.seatType = seatType;
}
public String getFlightNum()
{
return flightNum;
}
public String getFlightInfo()
{
return flightInfo;
}
public void setFlightInfo(String flightInfo)
{
this.flightInfo = flightInfo;
}
public boolean equals(Object other)
{
Reservation otherRes = (Reservation) other;
return flightNum.equals(otherRes.flightNum)&& name.equals(otherRes.name) && passport.equals(otherRes.passport);
}
public void print()
{
System.out.println(flightInfo + " " + name + " " + seat);
}
}
flights.txt
United_Airlines Dallas 1400 42
Air_Canada London 2300 78
Air_Canada Paris 2200 76
Porter_Airlines New_York 1200 16
United_Airlines New_York 0900 4
Air_Canada New_York 0600 14
United_Airlines Paris 2330 42
Air_Canada Tokyo 2200 100
You are to extend the Flight Reservation System program from the code and files given above (also add comments where necessary. This programming assignment will increase your knowledge of Java Collections, file IO and exceptions, objects and classes, inheritance, and interfaces. Finish before April 14 at least.
I strongly suggest you build your program in pieces. Get one command (e.g. SEATS command) compiled and working before working on the next piece. Read the marking scheme below and use it as a guide. You are permitted to add other instance variables and other methods if you think they are necessary.
- Make sure your files can be compiled as is without any further modification!!
- Include a README.txt file that describes what aspects of your program works and what doesn't work (or partially works). If your program does not compile, state this!!
Expected Output:
https://youtu.be/7dUWfryOBI8 (PLEASE watch the video and follow output SUPER closely)
Again, DO NOT forget about the README.txt part here again.
File I/O and exception handling for reading the flights.txt file and populating the flights map (or array list if you are not using a map) Good use of customized exceptions instead of Boolean+errMsg 2 marks 2 marks RES and CANCEL modifications as 2 marks specified above. PASMAN command. Correct printing of Seat Layout via 2 marks SEATS command Correct use of a Map for mapping seats to passengers and correct updating of seats occupied ("XX") Use of a Map in class FlightManager instead of array list and appropriate changes to all methods Finally a Finally b Penalty for insufficient comments 1 mark 1 1 mark 1 mark Up to -1 marks or bad program structure or bad use of exceptions, maps etc
Step by Step Solution
3.45 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
Aircraftjava public class Aircraft implements Comparable int numEconomySeats int numFirstClassSeats String model String seatLayout Boolean seatStatus public Aircraftint seats String model thisnumEcono...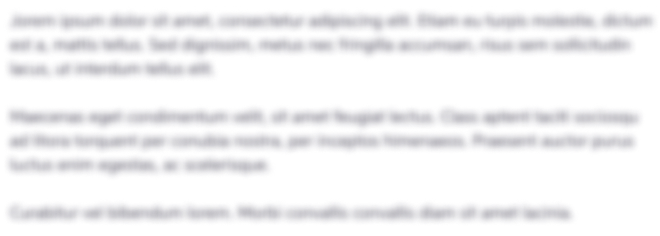
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started