Question
For Python 3 The following code prints the sample as the following: Sample Run: How many employees do you want to enter? 3 Enter a
For Python 3
The following code prints the sample as the following:
Sample Run:
How many employees do you want to enter? 3
Enter a name: Guido van Rossum
Enter hours worked: 42.5
Enter hourly rate: 285.11
Guido van Rossum should be paid $12,473.56
Enter a name: Blaise Pascal
Enter hours worked: 45
Enter hourly rate: 294
Blaise Pascal should be paid $13,965.00
Enter a name: Charles Babbage
Enter hours worked: 51
Enter hourly rate: 268
Charles Babbage should be paid $15,142.00
The total amount to be paid is $41,580.56
The average employee is paid $13,860.19
Add code that includes the output of the two upper lines. The total amout to be paid and the average employee pay
class employee: def getName(self): return self.name def getHours(self): return self.hours def getRate(self): return self.wage def setName(self, n): self.name = n def setHours(self, h): if(h>0): self.hours = h def setRate(self, w): if(w>0): self.wage = w def get_input(self): n = input("Enter a name: ") h = eval(input("Enter hours worked: ")) w = eval(input('Enter hourly rate: ')) self.setName(n) self.setHours(h) self.setRate(w) def calculatePay(self): '''Return the total weekly wages for a worker working totalHours, with a given regular hourlyWage. Include overtime for hours over 40. includes doubletime for hour over 60. ''' overtime = 0 if self.hours <= 40: regularHours = self.hours else: overtime = self.hours - 40 regularHours = 40 return self.wage * regularHours + (1.5 * self.wage) * overtime def __str__(self): return self.name+"should be paid $"+str(round(self.calculatePay(),2)) def main(): n = eval(input("How many employees do you want to enter? ")) for x in range(n): emp = employee() emp.get_input() print(emp) main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
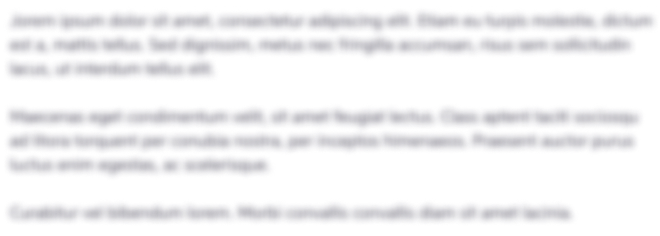
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started